Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial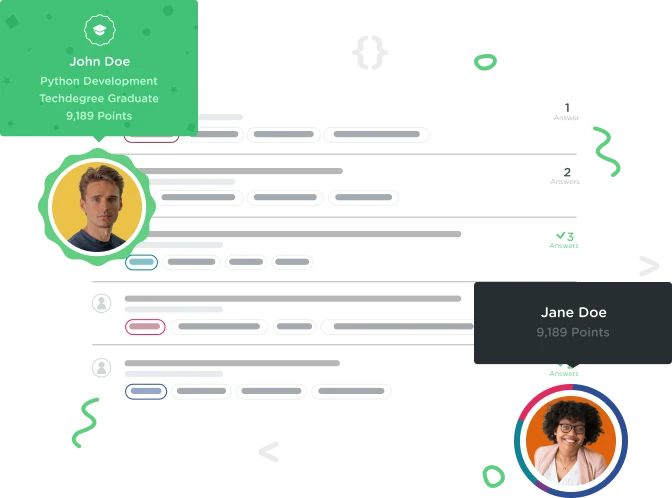
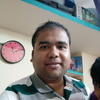
Lakshman Erukulla
5,688 PointsUnable to get the SpringBootApplication dependencies
Dear Team,
I am unable to get the Spring boot dependencies. I am using the latest Intellij IDE.
4 Answers

ejs27
10,205 PointsIn case you're like me and having issues with:
> Failed to apply plugin [class 'io.spring.gradle.dependencymanagement.DependencyManagementPlugin']
> Could not create task of type 'DependencyManagementReportTask'.
using IntelliJ IDEA and updating Gradle to version 4.8.1 as IntelliJ suggests, copy below to build.gradle
group 'com.teamtreehouse'
version '1.0-SNAPSHOT'
buildscript {
repositories {
maven {
url "https://plugins.gradle.org/m2/"
}
}
dependencies {
classpath "org.springframework.boot:spring-boot-gradle-plugin:2.2.2.RELEASE"
classpath "io.spring.gradle:dependency-management-plugin:1.0.8.RELEASE"
}
}
allprojects {
apply plugin: 'maven'
apply plugin: "io.spring.dependency-management"
group = 'com.wnp'
version = '6.5.0-SNAPSHOT'
}
apply plugin: 'java'
apply plugin: "org.springframework.boot"
sourceCompatibility = 1.8
targetCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
'io.spring.gradle:dependency-management-plugin:0.5.3.RELEASE'
compile 'org.springframework.boot:spring-boot-starter-web'
compile 'org.springframework.boot:spring-boot-starter-data-jpa'
compile 'org.springframework.boot:spring-boot-starter-thymeleaf'
compile 'com.h2database:h2'
compile 'org.eclipse.mylyn.github:org.eclipse.egit.github.core:2.1.5'
testCompile group: 'junit', name: 'junit', version: '4.11'
}
and copy below to gradle-wrapper.properties under /gradle/wrapper.
distributionUrl=https\://services.gradle.org/distributions/gradle-5.2.1-all.zip
distributionBase=GRADLE_USER_HOME
distributionPath=wrapper/dists
zipStorePath=wrapper/dists
zipStoreBase=GRADLE_USER_HOME
It'll update gradle to 5.2.1 and update build.gradle to correct format to apply io.spring.dependency-management. Which means, newer version of Spring Boot, which does not have "findOne" function so you'll also have to update FlashCardServiceimpl.java, which will pop up when you try to run App.java. Update with this:
package com.teamtreehouse.flashy.services;
import com.teamtreehouse.flashy.domain.FlashCard;
import com.teamtreehouse.flashy.repositories.FlashCardRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.*;
import static java.util.stream.Collectors.toList;
@Service
public class FlashCardServiceImpl implements FlashCardService {
private FlashCardRepository flashCardRepository;
@Autowired
public void setFlashCardRepository(FlashCardRepository flashCardRepository) {
this.flashCardRepository = flashCardRepository;
}
@Override
public Long getCurrentCount() {
return flashCardRepository.count();
}
@Override
public FlashCard getFlashCardById(Long id) {
return flashCardRepository.findById(id).get();
}
@Override
public FlashCard getNextUnseenFlashCard(Collection<Long> seenIds) {
List<FlashCard> unseen;
if (seenIds.size() > 0) {
unseen = flashCardRepository.findByIdNotIn(seenIds);
} else {
unseen = flashCardRepository.findAll();
}
FlashCard card = null;
if (unseen.size() > 0) {
card = unseen.get(new Random().nextInt(unseen.size()));
}
return card;
}
@Override
public FlashCard getNextFlashCardBasedOnViews(Map<Long, Long> idToViewCounts) {
FlashCard card = getNextUnseenFlashCard(idToViewCounts.keySet());
if (card != null) {
return card;
}
Long leastViewedId = null;
for (Map.Entry<Long, Long> entry : idToViewCounts.entrySet()) {
if (leastViewedId == null) {
leastViewedId = entry.getKey();
continue;
}
Long lowestScore = idToViewCounts.get(leastViewedId);
if (entry.getValue() >= lowestScore) {
break;
}
leastViewedId = entry.getKey();
}
return flashCardRepository.findById(leastViewedId).get();
}
@Override
public List<FlashCard> getRandomFlashCards(int amount) {
List<FlashCard> cards = flashCardRepository.findAll();
Collections.shuffle(cards);
return cards.stream()
.limit(amount)
.collect(toList());
}
}
I just replaced the "flashCardRepository.findOne(id)" to "flashCardRepository.findById(id).get()" to return correct variable (Optional<FlashCard> to FlashCard) in methods.

Andrzej Krzemień
15,339 PointsI have a problem with this solution and it gets like this: error: method save in interface CrudRepository<T,ID> cannot be applied to given types; flashCardRepository.save(cards); ^ required: S found: List<FlashCard> reason: inference variable S has incompatible bounds lower bounds: FlashCard lower bounds: List<FlashCard> where S,T,ID are type-variables: S extends FlashCard declared in method <S>save(S) T extends Object declared in interface CrudRepository ID extends Object declared in interface CrudRepository
What is wrong with it?

radoslawsoltan
12,043 PointsYou need to use saveAll instead of save method. As it takes list as a parameter. Your DatabaseLoader.java shoud look something like that:
package com.teamtreehouse.flashy.bootstrap;
import com.teamtreehouse.flashy.domain.FlashCard;
import com.teamtreehouse.flashy.repositories.FlashCardRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.List;
@Component
public class DatabaseLoader implements ApplicationRunner {
@Autowired
private FlashCardRepository flashCardRepository;
public void run(ApplicationArguments args) throws Exception {
List<FlashCard> cards = new ArrayList<>();
cards.add(new FlashCard("JDK", "Java Development Kit"));
cards.add(new FlashCard("YAGNI", "You Ain't Gonna Need It"));
cards.add(new FlashCard("SDK", "Software Development Kit"));
cards.add(new FlashCard("Java SE", "Java Standard Edition"));
cards.add(new FlashCard("Java EE", "Java Enterprise Edition"));
cards.add(new FlashCard("JRE", "Java Runtime Environment"));
cards.add(new FlashCard("JCL", "Java Class Library"));
cards.add(new FlashCard("JVM", "Java Virtual Machine"));
flashCardRepository.saveAll(cards);
}
}

Jose Hernandez-Inzunza
7,326 PointsIf you upgrade Gradle from the IntelliJ suggestion, all you need to do is add the gradle dependency management plugin that was available at that time. So since the spring boot gradle plugin version provided with the project is version 1.3.5
, looking here for 2016 releases of the dependency management plugin, https://mvnrepository.com/artifact/io.spring.gradle/dependency-management-plugin, we see that 0.6.0
or 0.6.1
should be the most compatible.
In the end I only added one line to the build.gradle
file.
group 'com.teamtreehouse'
version '1.0-SNAPSHOT'
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath("io.spring.gradle:dependency-management-plugin:0.6.1.RELEASE")
classpath("org.springframework.boot:spring-boot-gradle-plugin:1.3.5.RELEASE")
}
}
apply plugin: 'java'
apply plugin: 'spring-boot'
sourceCompatibility = 1.8
targetCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile 'org.springframework.boot:spring-boot-starter-web'
compile 'org.springframework.boot:spring-boot-starter-data-jpa'
compile 'org.springframework.boot:spring-boot-starter-thymeleaf'
compile 'com.h2database:h2'
compile 'org.eclipse.mylyn.github:org.eclipse.egit.github.core:2.1.5'
testCompile group: 'junit', name: 'junit', version: '4.11'
}
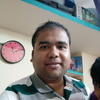
Lakshman Erukulla
5,688 PointsI am getting the below 2 lines after import in red colour
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication;
Oshedhe Munasinghe
8,108 PointsOshedhe Munasinghe
8,108 Pointsyou have saved my coding life thank you
Brian Spinos
15,722 PointsBrian Spinos
15,722 PointsDude, how the heck did you find this?