Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial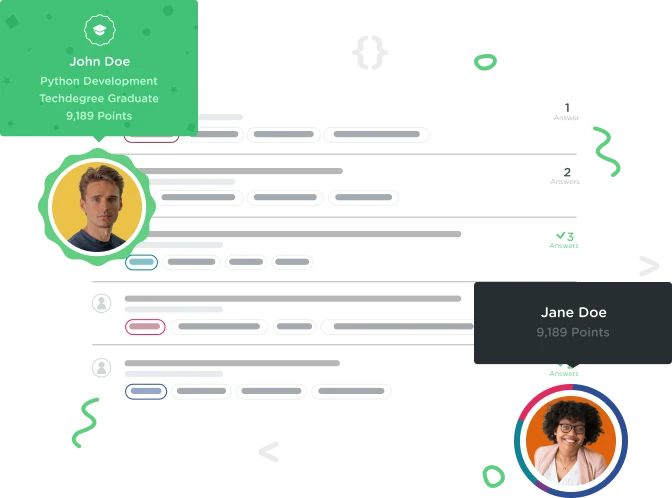

Alan Christensen
2,319 PointsUnable to get the Tag element to work
Hi!
I am having trouble with the description() instance method. I have created an init function in order to initialize the the Tag element of the struct however the description method is not returning the desired String. The online IDE says that code does not compile when it fact it does.
Can anyone give me a hand?
Thanks,
Alan
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, name: String){
self.title = title
self.author = author
self.tag = Tag(name: name)
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag)"
}
}
let firstPost = Post(title:"iOSDevelopment", author:"Apple", name: "swift")
let postDescription = firstPost.description()
1 Answer
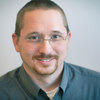
Jesse Anderson
iOS Development Techdegree Student 9,702 PointsI totally got stuck on this as well. And the web compiler didn't give much direction. The code you have works, you can put it in a playground and it will run. But what you want is for it to display the string iOSDevelopment by Apple. Filed under swift which I think it checks for. To fix this, remember that your Tag has a property called name. Even though the tag you set the init for has the name assigned to it, using it returns the full Tag struct of Tag(name: "swift"). If you type in tag in the playground, you can use the dot notation to pull up the name property that is assigned in the tag property you created. You should be able to get something like this:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, name: String){
self.title = title
self.author = author
self.tag = Tag(name: name)
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title:"iOSDevelopment", author:"Apple", name: "swift")
let postDescription = firstPost.description()
Try that and see if it works.
// UPDATED:
Ok, I got it now. I had to go back and double check what was actually being asked. And again, you did just what I did. This challenge isn't asking for that custom init method that you have in there. It just asked that you make a constant called tag of type Tag. So, if you remove that init method and just put in the line of code like this:
let firstPost = Post(title:"iOSDevelopment", author:"Apple", tag: Tag(name: "swift"))
Then that should work. Here we are not initializing the tag constant but passing it a value of type Tag when we assign it I think.
So try that. Remove the init and update that assignment line. Should work now.
Alan Christensen
2,319 PointsAlan Christensen
2,319 PointsThank you Jesse! great idea using the dot notation! it works just fine in Xcode but still no luck with the online IDE. Still stuck :(
Jesse Anderson
iOS Development Techdegree Student 9,702 PointsJesse Anderson
iOS Development Techdegree Student 9,702 PointsI've updated my answer. I hope that helps!