Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial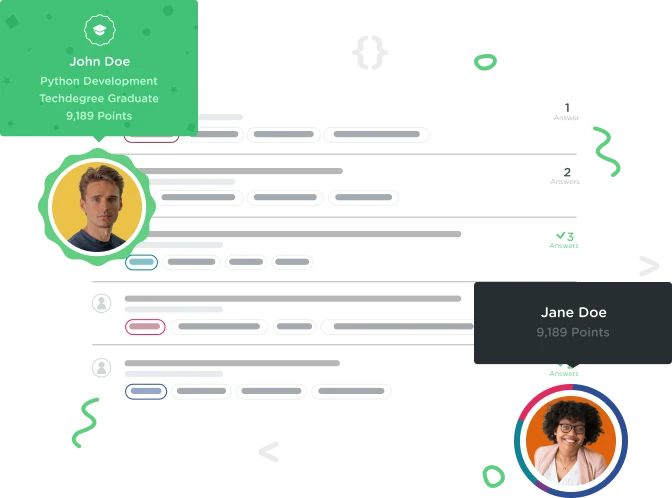
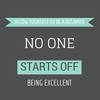
Hammad Nasir
Courses Plus Student 2,801 PointsUnable to import 'org.apache.commons.io.IOUtils' even after adding common-io-2.4.jar file to the libs folder.
Here's my FileHelper.java file's code: `
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
// error on this line
import org.apache.commons.io.IOUtils;
import android.content.Context;
import android.graphics.Bitmap;
import android.net.Uri;
import android.util.Log;
public class FileHelper {
public static final String TAG = FileHelper.class.getSimpleName();
public static final int SHORT_SIDE_TARGET = 1280;
public static byte[] getByteArrayFromFile(Context context, Uri uri) {
byte[] fileBytes = null;
InputStream inStream = null;
ByteArrayOutputStream outStream = null;
if (uri.getScheme().equals("content")) {
try {
inStream = context.getContentResolver().openInputStream(uri);
outStream = new ByteArrayOutputStream();
byte[] bytesFromFile = new byte[1024*1024]; // buffer size (1 MB)
int bytesRead = inStream.read(bytesFromFile);
while (bytesRead != -1) {
outStream.write(bytesFromFile, 0, bytesRead);
bytesRead = inStream.read(bytesFromFile);
}
fileBytes = outStream.toByteArray();
}
catch (IOException e) {
Log.e(TAG, e.getMessage());
}
finally {
try {
inStream.close();
outStream.close();
}
catch (IOException e) { /*( Intentionally blank */ }
}
}
else {
try {
File file = new File(uri.getPath());
FileInputStream fileInput = new FileInputStream(file);
fileBytes = IOUtils.toByteArray(fileInput); // error here
}
catch (IOException e) {
Log.e(TAG, e.getMessage());
}
}
return fileBytes;
}
public static byte[] reduceImageForUpload(byte[] imageData) {
Bitmap bitmap = ImageResizer.resizeImageMaintainAspectRatio(imageData, SHORT_SIDE_TARGET);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 100, outputStream);
byte[] reducedData = outputStream.toByteArray();
try {
outputStream.close();
}
catch (IOException e) {
// Intentionally blank
}
return reducedData;
}
public static String getFileName(Context context, Uri uri, String fileType) {
String fileName = "uploaded_file.";
if (fileType.equals(ParseConstants.TYPE_IMAGE)) {
fileName += "png";
}
else {
// For video, we want to get the actual file extension
if (uri.getScheme().equals("content")) {
// do it using the mime type
String mimeType = context.getContentResolver().getType(uri);
int slashIndex = mimeType.indexOf("/");
String fileExtension = mimeType.substring(slashIndex + 1);
fileName += fileExtension;
}
else {
fileName = uri.getLastPathSegment();
}
}
return fileName;
}
}
`
What should I do now? Please tell me @BenJakuben
1 Answer
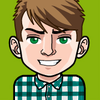
Harry James
14,780 PointsHey Hammad!
Although you've added the library, in Android Studio, we still need to set it as a dependency.
If you want to add anything in the libs directory to your project, you can add this line to your dependencies:
compile fileTree(dir: 'libs', include: ['*.jar'])
Alternatively, you can change the include statement to just include each individual jar file.
Hope it helps and if you run into any problems on the way, give me a shout :)
Hammad Nasir
Courses Plus Student 2,801 PointsHammad Nasir
Courses Plus Student 2,801 PointsThanks you, Harry! It worked!
Harry James
14,780 PointsHarry James
14,780 PointsWoohoo! Glad it helped! Happy coding :)