Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial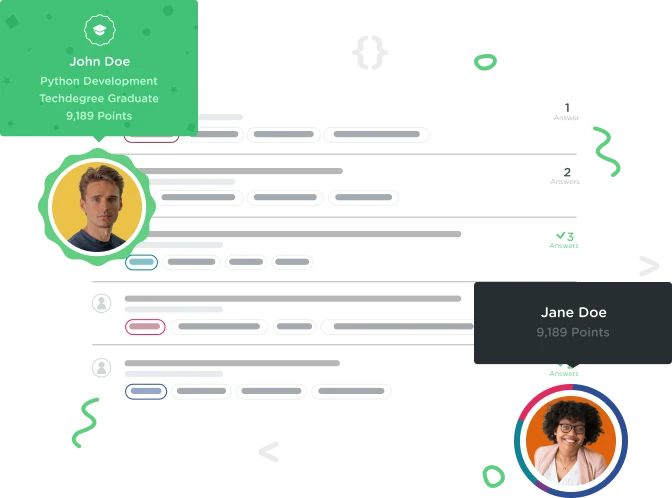

Bernard Chan
36,471 PointsUnable to import Scoreboard component
I'm unable to import the Scoreboard component in app.jsx.
This is my code in app.jsx
// libs
import React from 'react';
import ReactDOM from 'react-dom';
// components
import Scoreboard from './components/Scoreboard';
// css
import './app.css';
ReactDOM.render(
<Application initialPlayers={PLAYERS}/>, document.getElementById('container'));
This is my code in components/Scoreboard.jsx
import React from 'react';
var PLAYERS = [
{
name: "User One",
score: 69,
id: 1
},
{
name: "User two",
score: 69,
id: 2
}
];
var nextId = 3;
var Stopwatch = React.createClass({
getInitialState: function () {
return {
running: false,
elapsedTime: 0,
previousTime: 0,
}
},
componentDidMount: function () {
this.interval = setInterval(this.onTick, 100);
},
componentWillUnmount: function () {
clearInterval(this.interval);
},
onTick: function () {
if (this.state.running) {
var now = Date.now();
this.setState({
previousTime: now,
elapsedTime: this.state.elapsedTime + (now - this.state.previousTime),
});
}
},
onStart: function () {
this.setState({
running: true,
previousTime: Date.now(),
});
},
onStop: function () {
this.setState({running: false});
},
onReset: function () {
this.setState({
elapsedTime: 0,
previousTime: Date.now(),
});
},
render: function () {
var seconds = Math.floor(this.state.elapsedTime/ 1000);
return (
<div className = "stopwatch">
<h2>Stopwatch</h2>
<div className = "stopwatch-time">
{seconds}
</div>
{this.state.running ? <button onClick= {this.onStop}>Stop</button> : <button onClick = {this.onStart}>Start</button>}
<button onClick = {this.onReset}>Reset</button>
</div>
)
}
});
var AddPlayerForm = React.createClass({
propTypes: {
onAdd: React.PropTypes.func.isRequired,
},
getInitialState: function () {
return {
name: "",
};
},
onNameChange: function(e) {
this.setState({name:e.target.value});
},
onSubmit: function(e) {
e.preventDefault();
this.props.onAdd(this.state.name);
this.setState({name:""});
},
render: function() {
return (
<div className="add-player-form">
<form onSubmit={this.onSubmit}>
<input type="text" value={this.state.name} onChange={this.onNameChange}/>
<input type="submit" value="Add Player"/>
</form>
</div>
);
}
});
function Stats(props) {
var totalPlayers = props.players.length;
var totalPoints = props.players.reduce(function(total, player) {
return total + player.score;
}, 0);
return (
<table className="stats">
<tbody>
<tr>
<td>Players:</td>
<td>{totalPlayers}</td>
</tr>
<tr>
<td>Total Points:</td>
<td>{totalPoints}</td>
</tr>
</tbody>
</table>
);
}
Stats.propTypes = {
players: React.PropTypes.array.isRequired,
};
function Header(props) {
return (
<div className="header">
<Stats players={props.players}/>
<h1>{props.title}</h1>
<Stopwatch />
</div>
);
}
Header.proptypes = {
title: React.PropTypes.string.isRequired,
players: React.PropTypes.array.isRequired,
};
function Counter(props) {
return(
<div className="counter">
<button className="counter-action decrement" onClick={function() {props.onChange(-1);}}>
-
</button>
<div className="counter-score">
{props.score}
</div>
<button className="counter-action increment" onClick={function() {props.onChange(1);}}>
+
</button>
</div>
);
}
Counter.propTypes = {
score : React.PropTypes.number.isRequired,
onChange: React.PropTypes.func.isRequired,
}
function Player(props) {
return (
<div className="player">
<div className="player-name">
<a className="remove-player" onClick = {props.onRemove}> X </a>
{props.name}
</div>
<div className="player-score">
<Counter score={props.score} onChange={props.onScoreChange} />
</div>
</div>
);
}
Player.proptypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
onScoreChange: React.PropTypes.func.isRequired,
onRemove: React.PropTypes.func.isRequired,
}
var Application = React.createClass({
propTypes: {
title: React.PropTypes.string,
initialPlayers: React.PropTypes.arrayOf(React.PropTypes.shape({
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
id: React.PropTypes.number.isRequired
})).isRequired
},
getDefaultProps: function () {
return {
title: "ScoreBoard",
};
},
getInitialState: function () {
return {
players: this.props.initialPlayers,
};
},
onScoreChange: function (index, delta) {
this.state.players[index].score += delta;
this.setState(this.state);
},
onPlayerAdd: function(name) {
this.state.players.push({
name: name,
score: 0,
id: nextId,
});
this.setState(this.state);
nextId += 1;
},
onRemovePlayer: function(index) {
this.state.players.splice(index, 1);
this.setState(this.state);
},
render: function() {
return (
<div className="scoreboard">
<Header title={this.props.title} players={this.state.players} />
<div className="players">
{this.state.players.map(function(player, index) {
return (
<Player
onScoreChange ={function(delta) {this.onScoreChange(index ,delta)}.bind(this)}
onRemove={function() {this.onRemovePlayer(index)}.bind(this)}
name={player.name}
score={player.score}
key={player.id} />
);
}.bind(this))}
</div>
<AddPlayerForm onAdd={this.onPlayerAdd}/>
</div>
);
}
});
export default Scoreboard;
The error I'm receiving is this.
ERROR in ./app.jsx
Module not found: Error: Can't resolve './components/Scoreboard' in
'/Users/user1/rails_projects/Scoreboard'
@ ./app.jsx 6:0-49
@ multi (webpack)-dev-server/client?http://localhost:8080 webpack/hot/dev-server ./app.jsx
webpack: Failed to compile.
1 Answer
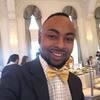
Kyree S. Williams
1,713 PointsI had this issue, too. If you're going to use JSX, you need to specify the .jsx file extension on your import of Scoreboard.. So, instead of import Scoreboard from './components/Scoreboard';
, you will need to use the statement import Scoreboard from './components/Scoreboard.jsx';