Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial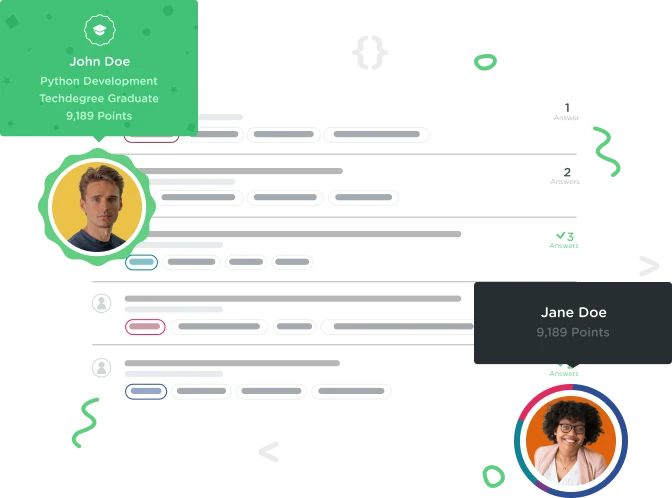

Shahrier Akram
6,503 PointsUnable to pass challenge task in error handling on swift
Not sure why this isn't an accepted answer:
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.emptyDictionary
}
guard case let someValue?? = data["someKey"] else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
try parser.parse()
} catch ParserError.invalidKey {
print("Got invalid key")
} catch ParserError.emptyDictionary {
print("Got an empty dictionary")
}
enum ParserError: Error {
case emptyDictionary
case invalidKey
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
func parse() throws {
guard let data = data else {
throw ParserError.emptyDictionary
}
let keyExists = data["someKey"] != nil
if let value {
throw ParserError.InvalidKey
}
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
3 Answers

Shahrier Akram
6,503 PointsGotcha. Thanks Max!

Max Ramirez
iOS Development Techdegree Graduate 17,361 PointsYou're welcome!
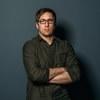
Jesse Gay
Full Stack JavaScript Techdegree Student 14,045 PointsThanks Max, great explanation!
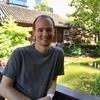
Zach Hudson
7,702 PointsHow does this code make sure the dictionary is not empty?
guard let data = data else {
throw ParserError.emptyDictionary
}
Max Ramirez
iOS Development Techdegree Graduate 17,361 PointsMax Ramirez
iOS Development Techdegree Graduate 17,361 PointsHey Shahrier, In the challenge it says "Hint: To get a list of keys in a dictionary you can use the keys property which returns an array. Use the contains method on it to check if the value exists in the array" Your top portion for emptyDictionary is correct. Lets work on the invalidKey. Following the hint: keys property & use the contains method to check if the value exists in the array.
We get keys & contains using dot notation on data
Then when we do the do catch statement for task 2:
And using pattern matching We do the following using our ParserError Enum for task 3
I hope this helped!