Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial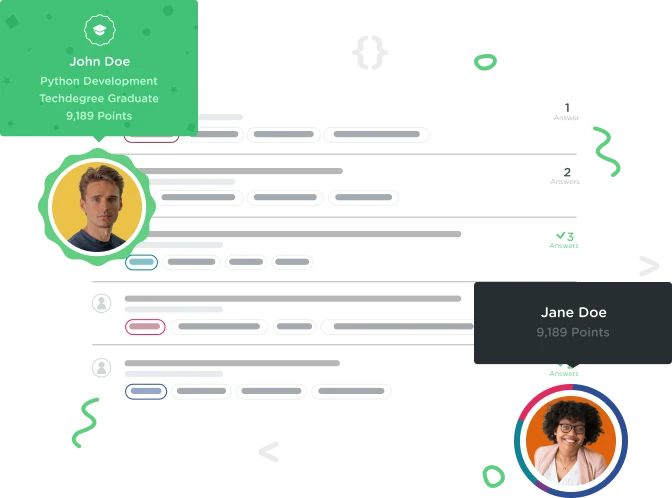

Shane McC
3,005 PointsUnable to pass variable from one function to another function in php
This is what I'm tying to do.
(When I refer to state and city. I literally mean US states and cities, New York, Texas, Houston etc...)
In order for me to display similar dealers on every dealership page I'm going to have to use the state and city variables. In my original function I'm passing the city variable but not the state variable. I've extracted the state variable via a function I've made (syntax below) but this is where I'm running into trouble.
When I extract the state I'm attempting to convert it as a variable but it's not working (syntax below). My question is, how would I convert what I'm extracting (the state) to a variable?
In the below function I'm getting the state from the id that I'm passing.
function get_state_from_the_id($id){
$this->db->select('state');
$this->db->from('dealers');
$this->db->where('id',$id);
$query = $this->db->get();
return $query->result();
}
This is where I'm running into trouble
$state = ($this->Dealer_data->get_state_from_the_id($id) > 0) ? FALSE : TRUE;
In the above line of code I'm attempting to extract the state as a variable from my function but it's not working. My objective is to extract the state (IE, new york, texas, california etc...) and insert that variable into another function I made. What am I doing wrong? How can I correct my mistakes?
Thanks
1 Answer

Ryan Stratton
19,181 PointsIs this Codeigniter? If not where are you getting the 'db' object from?
If this is Codeigniter, which I suspect it is, then the $query->result() method will always return an array of objects, or an empty array if no rows are returned. Because the object your looking for is inside an array, the ternary expression won't work as expected. Here's the Codeigniter docs on query results: http://ellislab.com/codeigniter/user-guide/database/results.html.
And here's how I would tackle the problem.
function get_state_from_the_id($id){
$this->db->select('state');
$this->db->from('dealers');
$this->db->where('id',$id);
$query = $this->db->get();
if ($query->num_rows() > 0) {
return $query->row(); // return a single row, or first row
}
}
// should really check for results first or you might get an error
$state = $this->Dealer_data->get_state_from_the_id($id)->state ?: false;
If this isn't Codeingiter, where's the db object coming from? And how are results being returned?
Shane McC
3,005 PointsShane McC
3,005 PointsRyan, it is codeigniter.
I figured it out.
In this function I'm getting the state that's associated with the ID. I was messing up with how I was getting my state variable. That's why my syntax above didn't work. My syntax below is working great.
My Controller
$data['simInfo'] = $this->Dealer_data->get_similiar_dealers($state/*, $city*/);
My View