Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial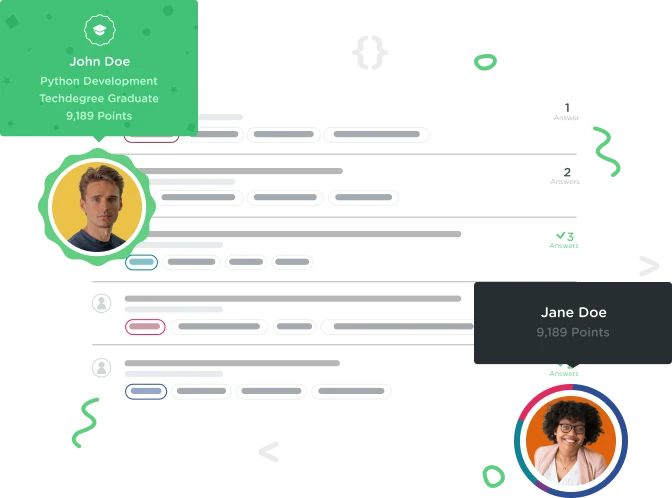

alexlitel
25,059 PointsUnable to print JSON values
I'm trying to work on a simple HTML/CSS/jQuery site that returns movie showtimes and reviews when someone types in a ZIP code.
I'm able to use my API key and generate a JSON file using the variables and such, but I can't seem to get the callback function in the second part of the .getJSON method to work. Unlike the Flickr site in the AJAX course, there are no names to the JSON array, which might be causing issues. If anyone could help it would be appreciated.
The generated JSON file looks like this: http://i.imgur.com/6bnjaZP.png
$('form').submit(function(evt) {
//prevent default activity
evt.preventDefault();
//cache value of zip search
//
var search = $('#submit');
$('#search').prop("disabled", true);
search.attr("disabled", true).val("searching...");
// highlight the button
// not AJAX, just cool looking
// the AJAX part
var apiKey = "xxxxxx";
var zipCode = $('#search').val();
var currentDate = new Date();
var today = currentDate.getFullYear() + '-' + (currentDate.getMonth()+1) + '-' + currentDate.getDate();
var showtimesUrl = "http://data.tmsapi.com/v1.1/movies/showings?startDate=" + today + "&zip=" + zipCode + "&jsonp=displayShowtimes&api_key=" + apiKey;
function displayShowtimes(data) {
$.each([], function(index,movie) {
listings += '<li class="movie">';
listings += movie.title;
listings += '</li>';
console.log(movie.title);
}); // end each
listings += '</ul>';
$('#showtimes').append(listings);
}
//function
$.getJSON(showtimesUrl, {}, displayShowtimes);
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Movie Theaters and Showtimes</title>
<link href='http://fonts.googleapis.com/css?family=Droid+Serif|Open+Sans:300,800,400' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="container">
<div class="header">
<h1>Movie Showtimes and Reviews</h1>
<p>Check local showtimes and movie reviews</p>
</div>
<div class="form-section">
<form>
<label for="search">Enter your ZIP code</label>
<input type="search" name="search" id="search">
<input type="submit" value="Search" id="submit">
</form>
</div>
<div id="showtimes">
</div>
</div>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="js/app.js"></script>
</body>
</html>
1 Answer

LaVaughn Haynes
12,397 PointsYou are super close. You need to pass "data" to your each loop. You are giving it an empty array. Try this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div id="showtimes"></div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script>
var movies = [
{ title: 'Minions'},
{ title: 'Jurassic World'},
{ title: 'Ant-Man'}
];
function displayShowtimes(data) {
//define listings
var listings ='<ul>';
//add data, not empty array
//$.each([], function(index,movie) {
$.each(data, function(index, movie) {
listings += '<li class="movie">';
listings += movie.title;
listings += '</li>';
console.log(movie.title);
}); // end each
listings += '</ul>';
$('#showtimes').append(listings);
}
displayShowtimes(movies);
</script>
</body>
</html>
alexlitel
25,059 Pointsalexlitel
25,059 PointsI used "data" as the first parameter of the $.each method previously, and it wasn't working. I'm not trying to trying to work through a static list in the HTML/script, but dynamically generated JSONs from an external source, hence utilizing APIs and such. Do I need to parse the JSON data in some other method beforehand?
LaVaughn Haynes
12,397 PointsLaVaughn Haynes
12,397 PointsYep, the static list is just to have some data to work with. I think you had it correct the first time using data though. You are using getJSON so you shouldn't have to parse the returned data. It should return json by default.
The second part of the problem was that you don't define listings in your function which causes an error when you try to concat a string to an undefined variable.
"Uncaught ReferenceError: listings is not defined"
I would add the listings definition to your code your code AND the data param and try it.
var listings ='<ul>';
To see what I mean run my example code in your browser. It displays the movies. Then remove the line that defines listings. It then doesn't display anything. But if you check the console it will show that error. I think that both of these things are why you are not seeing output. Anyway, that's my best guess without having access to the data.
Good luck. The app looks good so far!
alexlitel
25,059 Pointsalexlitel
25,059 PointsI moved up the variable and fiddled around a little bit and it finally worked. Thanks!
LaVaughn Haynes
12,397 PointsLaVaughn Haynes
12,397 PointsExcellent. Seeing how nice your project looks actually inspired me to hop back on a project I've been wanting to do (game stuff)