Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial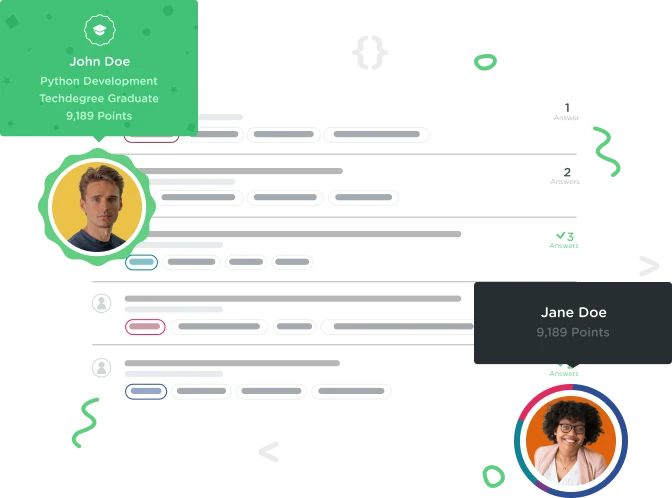
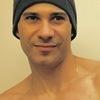
Ronaldo Fialho
5,105 Pointsunable to retrieve single data
function full_catalog_array() { include 'connection.php';
try {
$results = $db->query("SELECT media_id, title, category, img FROM Media");
} catch(Exception $e) {
echo "Unable to retrieve results";
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
function single_item_array($id) { include 'connection.php';
try {
$results = $db->query("SELECT media_id, title, category, img, format, year, genre, publisher, isbn
FROM Media JOIN Genres ON Media.genre_id = Genres.genre_id
LEFT OUTER JOIN Books ON Media.media_id = Books.media_id
WHERE Media.media_id = $id");
} catch(Exception $e) {
echo "Unable to retrieve single results";
exit;
}
$catalog = $results->fetch();
return $catalog;
}
var_dump(single_item_array(1));
1 Answer

Eric Butler
33,512 PointsRonaldo, I'm going to guess that you need to specify which table some (or all, for good measure) of your SELECT fields are coming from, for example:
SELECT Media.media_id, Media.title, [...] Books.publisher, Books.isbn FROM Media [...]
Because SQL might be confused on which table you want to pull the field from.
However, you're really missing out on the built-in debugger that comes with your try-catch block, which will probably tell you exactly what's wrong and help you debug your SQL and other PHP errors for a long time to come:
<?php
try {
// all your existing stuff
} catch(Exception $e) {
echo "<p>Unable to retrieve single results. The error is: " . $e->getMessage() . " and the complete error object is:</p>";
echo "<pre>";
print_r($e);
echo "</pre>";
exit();
}
?>
The $e
variable holds all the details about the error that was encountered. Its getMessage
method is a great clue about what's wrong. Use it and debug easier! Hope this helps, and hope you figure it out and can get back to coding. I'd be happy to hear what you find, so come back and let me know. Good luck.
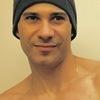
Ronaldo Fialho
5,105 PointsThx Eric!! SQLSTATE[HY000]: General error: 1 ambiguous column name: media_id

Eric Butler
33,512 PointsExcellent! Glad you got it. Those error messages are always so helpful for debugging.
Nick Girling
4,607 PointsNick Girling
4,607 PointsIt would really help us help you if you could put this code into 3 backticks (```) so it was more readable. See the markdown Cheatsheet for more info.