Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial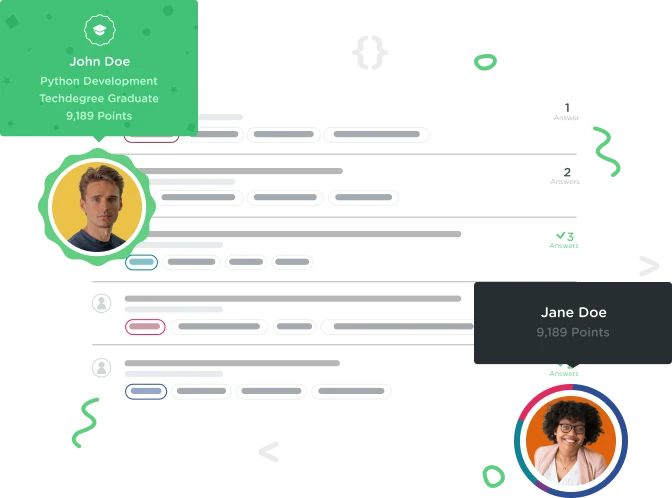
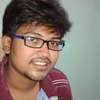
Vamsi Pavan Mahesh Gunturu
4,541 Pointsunable to use methods inside methods
class MyName
def initialize(title, firstName, middleName, lastName)
@title = title
@first = firstName
@middle = middleName
@last = lastName
end
def title
puts @title
end
def first
puts @first
end
def middle
puts @middle
end
def last
puts @last
end
def full
title() + first()
end
end
name = MyName.new("mr","vamsi","pavan","mahesh")
name.title
name.first
name.middle
name.last
name.full
This gives me
myclass.rb:28:in `full': undefined method `+' for nil:NilClass (NoMethodError)
from myclass.rb:37:in `<main>'
3 Answers

Roberto Alicata
Courses Plus Student 39,959 Pointsdelete the "puts" statements in the functions and it will works

Tim Knight
28,888 PointsVamsi, Ruby actually makes this a lot easier on you by just creating reader accessors. Additionally it's more idiomatic in Ruby to use snake-case over camel case, so another way to rewrite this whole block would be:
class MyName
attr_reader :title, :first_name, :middle_name, :last_name
def initialize(title, first_name, middle_name, last_name)
@title = title
@first_name = first_name
@middle_name = middle_name
@last_name = last_name
end
def full_name
"#{title} #{first_name} #{middle_name} #{last_name}"
end
end
name = MyName.new("mr","vamsi","pavan","mahesh")
puts name.title # => mr
puts name.first_name # => vamsi
puts name.middle_name # => pavan
puts name.last_name # => mahesh
puts name.full_name # => mr vamsi pavan mahesh

Chris Ward
12,129 PointsIs puts returning nil?
Try this code instead in your custom defined getters:
retVal = puts @instanceVarName
retVal = (retVal.is_nil?) ? @instanceVarName.to_s : retVal.to_s
DONT FORGET TO REPLACE @instanceVarName WITH THE NAME OF THE INSTANCE VAR!

Tim Knight
28,888 PointsRemember that a method returns an output, it doesn't "put" an output... if you run puts by itself you'll see it returns nil. If you puts with string you'll get the string and then nil back. So as far as I can see it, if you us puts as the last thing in a method it's going to return the last thing in the method (which Ruby does by default), which is in fact nil.
2.2.1 :001 > @name = "Tim"
=> "Tim"
2.2.1 :002 > @name
=> "Tim"
2.2.1 :003 > def name
2.2.1 :004?> puts @name
2.2.1 :005?> end
=> :name
2.2.1 :006 > name
Tim
=> nil
2.2.1 :007 > def name
2.2.1 :008?> @name
2.2.1 :009?> end
=> :name
2.2.1 :010 > name
=> "Tim"
2.2.1 :011 >
Vamsi Pavan Mahesh Gunturu
4,541 PointsVamsi Pavan Mahesh Gunturu
4,541 PointsThanks!