Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial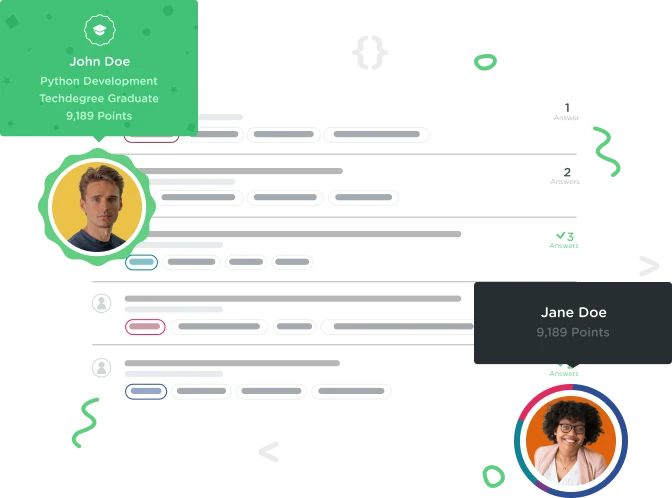
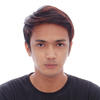
Karl Michael Figuerrez
963 PointsUnboundLocalError: local variable 'num' referenced before assignment
what's wrong with my code? the statement in the under except won't run?
# EXAMPLES
def squared(x):
try:
num = int(x)
except ValueError:
print(num +" not a number.")
return num*len(num)
return num ** 2
squared("five")
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
2 Answers

Charlie Gallentine
12,092 PointsAfter doing some testing on my own text editor, it looks like you should have no issue declaring a variable under the try statement.
After looking at it some more, in the except block, num is never defined in your code. I was looking at the problem wrong but it appears that after executing the 'try' block, it resets completely and loses the num declaration. Your code passed when I wrote it as:
def squared(x):
try:
num = int(x)
except ValueError:
num = x
print(" not a number.{}".format(num))
return num*len(num)
return num ** 2
I was wrong in how I addressed your problem the first time, but maybe this will clear it up?

Charlie Gallentine
12,092 PointsNum isn't defined because it is being called without being defined. The error that num isn't defined can be fixed by taking the number variable out of the script entirely and only using the 'x' parameter.
def squared(x):
try:
int(x)
except ValueError:
print(x +" not a number.")
return x*len(x)
return x ** 2
However, this will still not work as needed for the challenge. It will throw a TypeError. To fix this, I deleted the end 'return' statement and put it in the 'try' block.
def squared(x):
try:
int(x)
return x**2
except ValueError:
print(x +" not a number.")
return x*len(x)
This still does not convert the argument into an int, it simply checks if it is one, moves on, and returns a TypeError as it can't square a string with the ** operator. The final code I wrote to pass the code was:
def squared(x):
try:
return int(x)**2
except:
print("{} not a number.".format(x))
return x * len(x)
This will try to square an integer value of the parameter. If that's not possible, it will go into the except block. Once there, it prints a message and multiplies the string by its length. I also changed the implementation of the print statement's formatting to insert the value.
I know that doesn't really answer your question, but I hope it was helpful!
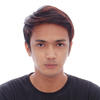
Karl Michael Figuerrez
963 Pointsso you can't really assign anything under the try statement?

Charlie Gallentine
12,092 PointsAfter doing some testing on my own text editor, it looks like you should have no issue declaring a variable under the try statement.
After looking at it some more, in the except block, num is never defined in your code. I was looking at the problem wrong but it appears that after executing the 'try' block, it resets completely and loses the num declaration. Your code passed when I wrote it as:
def squared(x): try: num = int(x) except ValueError: num = x print(" not a number.{}".format(num)) return num*len(num) return num ** 2 I was wrong in how I addressed your problem the first time, but maybe this will clear it up?
Karl Michael Figuerrez
963 PointsKarl Michael Figuerrez
963 Pointsok bro, tnx for feedback.