Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial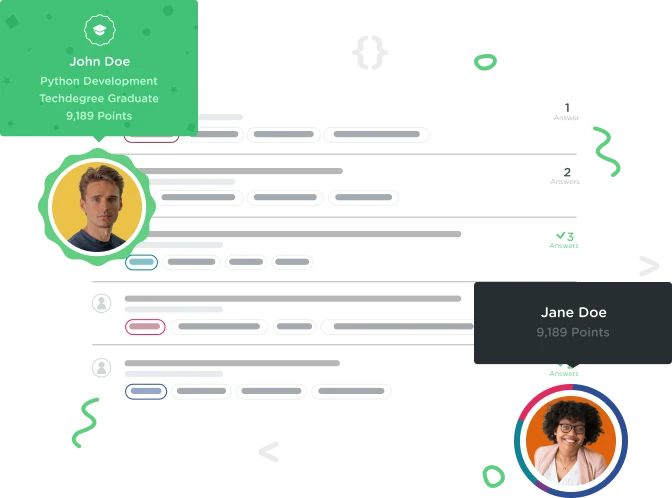

jun cheng wong
17,021 PointsUnboundLocalError: local variable 'tickets_remaining' referenced before assignment
How can I solve this? UnboundLocalError: local variable 'tickets_remaining' referenced before assignment
TICKET_PRICE = 10
tickets_remaining = 100
#run this program until tickets finished.
def runProgram():
#Output how many tickets are remaining using the tickets_remaining variable
print(("There are {} tickets remaining, be sure to grab it quick!!!").format(tickets_remaining))
#Gather the users' name and assign it to a new variable.
user_name = input("What is your name? ")
#Prompt the users by name and ask the user how many tickets they want
ticket_number = int(input("Welcome to the concert, {}. How many tickets do you want to purchase? ".format(user_name)))
if ticket_number < tickets_remaining:
#Calculate the price (number of tickets x ticket price) and assign thhat to a variable
total_price = TICKET_PRICE * ticket_number
#output the price to the screen
print('You have ordered {} tickets. The total price is ${}'.format(ticket_number,total_price))
#prompt users if they want to proceed? Y/N
user_proceed = input('Do you want to proceed to the payment process? Y or N ')
user_proceed = user_proceed.upper()
#If they want to proceed,
if user_proceed == "Y":
#we output it on the screen "sold" to confirm purchase.
#Gather credit card information and process it.
print('SOLD!!!')
#and then decrement the ticket remaining.
tickets_remaining -= ticket_number
#otherwise just thank them
else:
print('Thank you, {}'.format(user_name))
else:
print('We are sorry, there are only {} tickets available.')
if tickets_remaining > 0:
runProgram()
else:
print("We have sold out all the tickets")
1 Answer
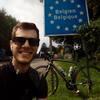
Felipe Giovanni de Moura Santos
23,702 PointsWhats up Jun...
On the error, tickets_remaining is being called as a "local variable". That gives us a hint that you're having some scope issue with it.
tickets_remaining is a global variable and in Python, there is no problem in using it inside a function. However, you're not just using it, you're updating it from inside the function. To change the value of a global variable from inside a function in Python, according to the w3schools.com you must reference it inside the function using the global keyword.
This will do the work:
def runProgram():
global tickets_remaining
#rest of the block
reference: https://www.w3schools.com/python/python_variables_global.asp
jun cheng wong
17,021 Pointsjun cheng wong
17,021 Pointsthanks, i understand already