Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial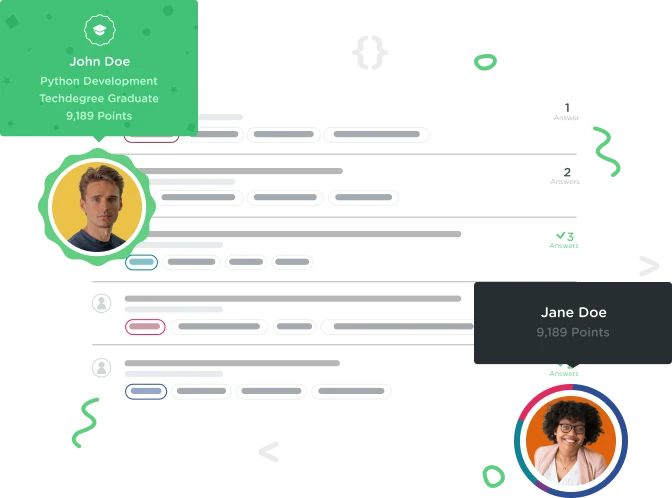

mohamedhalabi
1,968 PointsUncaught Error: This is not a Number ? Appreciate your help
var lower = parseInt (prompt ('Enter Min Number') ) ;
var upper = parseInt (prompt ('Enter Max Number') ) ;
function getRandomNumber (lower , upper){
if (upper > lower){
var randomNumber = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return randomNumber;
} else if (lower > upper) {
var randomNumber = Math.floor(Math.random() * (lower - upper + 1)) + upper;
return randomNumber;
}else if (isNaN(upper) || isNaN(lower))
throw new Error ('This is not a Number');
}
console.log( getRandomNumber());
Im getting this Error: Uncaught Error: This is not a Number ~!
3 Answers
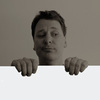
Sean T. Unwin
28,690 Pointsa quick question if you dont mind: i thought all functions can access the global scope and use the variables available if they are not found inside the function
This is true; you are correct.
This is not the issue, however. The error is being given because when calling, getRandomNumber()
with console.log( getRandomNumber());
there are no parameters being passed -- it takes 2 numbers.
Also keep in mind that a function's named parameters are only accessible within the function. This means that while getRandomNumber(lower, lower)
is stated in the function declaration, the labels upper
and lower
do NOT reflect any other part of the overall script. i.e. when upper
and/or lower
are declared outside the function, they have no relevance within the function. The parameters may have the same name as an external variable (external to the function), but that is where the relationship ends.
TL;DR: For the code to work in the OP, we need to pass upper
and lower
to the function call.
i.e. getRandomNumber(lower , upper);
If using this method it's probably best not to call the function from within console.log
.
However, if you do intend to use One Weru 's answer, it's probably best to remove the parameters in the function declaration as the prompts' input will be stored within each function call.
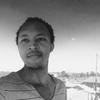
Dan Weru
47,649 PointsHey, I think your variable declarations should be inside the function
function getRandomNumber (lower , upper){
var lower = parseInt (prompt ('Enter Min Number') ) ;
var upper = parseInt (prompt ('Enter Max Number') ) ;
if (upper > lower){
var randomNumber = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return randomNumber;
} else if (lower > upper) {
var randomNumber = Math.floor(Math.random() * (lower - upper + 1)) + upper;
return randomNumber;
} else if (isNaN(upper) || isNaN(lower)){
throw new Error ('This is not a Number');
}
}
console.log( getRandomNumber());
In my opinion, the reason you're getting the error is because of function hoisting.

mohamedhalabi
1,968 Pointsthanks for this ! just a quick question if you dont mind: i thought all functions can access the global scope and use the variables available if they are not found inside the function >?
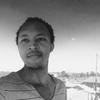
Dan Weru
47,649 PointsThereβs a subtle difference in this situation. While, functions can access the global variables, this situation is unique in that the variables values are being assigned later, when you fill in the prompt dialogue. On the other hand, the function is being declared immediately. Therefore, in your earlier code, when the function is declared and called, the values of lower and upper variables are both undefined, hence your code throws an error all the time.

mohamedhalabi
1,968 Pointsthat makes sense as well ! much appreciated
mohamedhalabi
1,968 Pointsmohamedhalabi
1,968 PointsGreat feedback ! thanks !