Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial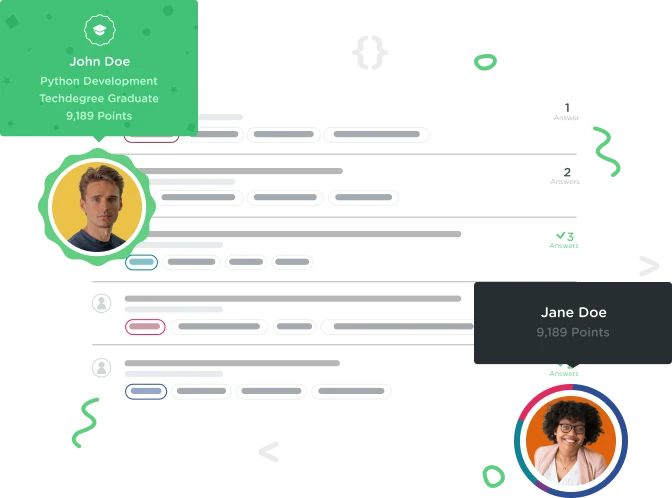

Homero Mendoza
12,782 PointsUncaught (in promise) TypeError: data.map
have this error when adding the Promise.all
Uncaught (in promise) TypeError: data.map is not a function at generateOptions (app.js:46:22) at app.js:26:1
const select = document.getElementById('breeds');
const card = document.querySelector('.card');
const form = document.querySelector('form');
// ------------------------------------------
// FETCH FUNCTIONS
// ------------------------------------------
// function use to make code better an fetch with json
function fetchData(url){
return fetch(url)
.then(checkStatus)
.then(res => res.json())
.catch(error => console.log('looks like there was a problem', error) )
}
//fetch the img api
Promise.all([
fetchData('https://dog.ceo/api/breeds/image/random'),
fetchData('https://dog.ceo/api/breeds/list')
])
.then(data => {
const breedList = data[0].message;
const randomImage = data[1].message;
generateOptions(breedList);
generateImage(randomImage)
});
// ------------------------------------------
// HELPER FUNCTIONS
// ------------------------------------------
function checkStatus(response){
if (response.ok){
return Promise.resolve(response);
}else {
return Promise.reject(new Error(response.statusText));
}
}
function generateOptions(data){
const options = data.map(item => `
<option value ='${item}'>${item}</option>
`).join('');
select.innerHTML = options;
}
function generateImage(data){
const html= `
<img src ='${data}' alt>
<p>Click to view images of ${select.value}s</p>` ;
card.innerHTML = html;
}
function fetchBreedImage(){
const breed = select.value;
const img = card.querySelector('img');
const p = card.querySelector('p');
fetchData(`https://dog.ceo/api/breed/${breed}/images/random`)
.then(data => {
img.src = data.message;
img.alt = breed;
p.textContent = `Click to view more ${breed}s`;
})
}
// ------------------------------------------
// EVENT LISTENERS
// ------------------------------------------
select.addEventListener('change', fetchBreedImage);
card.addEventListener('click', fetchBreedImage);
// ------------------------------------------
// POST DATA
// ------------------------------------------
1 Answer
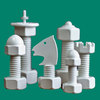
Steven Parker
231,268 PointsThe index values for breedList and randomImage are reversed. The breedList should be made from data[1], and randomImage from data[0].
Or, you could change the order of the fetchData calls.