Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial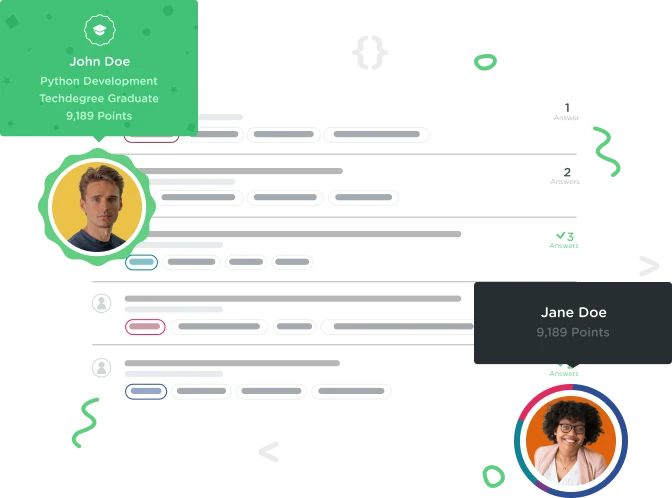
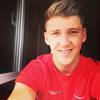
Jay Valentine
Courses Plus Student 4,389 PointsUncaught SyntaxError: missing ) after argument list
Console log says ----> "quiz.js:46" So apparently there is a missing ')' on line 46..
This is my script..
/*
This is my attempt to make a program to do the following:
1- Create a 5 questions quiz.
2- Keep track of player's correct answers.
3- Print a final message telling the player how many correct answer did he/she got.
4- Rank the player.
*/
// Player Name
var playerName = prompt('Please provide your name before we start the quiz.');
// quiz begins no answers correct
var correct = 0;
// Ask questions
var answer1 = prompt("Name a programming language that's the name of a gem?");
if (answer1.toUpperCase() === 'RUBY') {
correct += 1;
}
var answer2 = prompt("What is the name of the man who thought of the theory of relativity?");
if (answer2.toUpperCase() === 'ALBERT' || answer2 === 'ALBERT EINSTEIN' || answer2 === 'EINSTEIN') {
correct += 1;
}
var answer3 = prompt("How many days in a full week");
if (answer3.toUpperCase() === 'SEVEN' || answer3 === '7') {
correct += 1;
}
var answer4 = prompt("What animal was the inspiration for the film 'Jaws'");
if (answer4.toUpperCase() === 'shark') {
correct += 1;
}
var answer5 = prompt("Who was the President of the United States in 2014");
if (answer5.toUpperCase() === 'OBAMA' || answer5.toUpperCase === 'BARACK OBAMA' || answer5.toUpperCase === 'BARACK') {
correct += 1;
}
// Output results
// LINE 46 BELOW
document.write('<p>Well done,v' + playerName ' you got ' + correct 'out of 5 correct</p>');
2 Answers
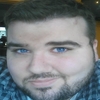
Marcus Parsons
15,719 PointsHi Jay,
I found a few problems with your code. The main problem was some missing + operators in your bottom document.write() command which caused that error. JavaScript errors are rarely, if ever, actually helpful lol. Also, there are some logic errors with missing calls to toUpperCase.
You can verify this is correct by running it in your console in Chrome.
I put comments everywhere something needed to be changed:
/*
This is my attempt to make a program to do the following:
1- Create a 5 questions quiz.
2- Keep track of player's correct answers.
3- Print a final message telling the player how many correct answer did he/she got.
4- Rank the player.
*/
// Player Name
var playerName = prompt('Please provide your name before we start the quiz.');
// quiz begins no answers correct
var correct = 0;
// Ask questions
var answer1 = prompt("Name a programming language that's the name of a gem?");
if (answer1.toUpperCase() === 'RUBY') {
correct += 1;
}
var answer2 = prompt("What is the name of the man who thought of the theory of relativity?");
//missing toUpperCase on last 2 answer checks
if (answer2.toUpperCase() === 'ALBERT' || answer2.toUpperCase() === 'ALBERT EINSTEIN' || answer2.toUpperCase() === 'EINSTEIN') {
correct += 1;
}
var answer3 = prompt("How many days in a full week");
if (answer3.toUpperCase() === 'SEVEN' || answer3 === '7') {
correct += 1;
}
var answer4 = prompt("What animal was the inspiration for the film 'Jaws'");
//changed shark to SHARK for toUpperCase call
if (answer4.toUpperCase() === 'SHARK') {
correct += 1;
}
var answer5 = prompt("Who was the President of the United States in 2014");
//missing () on toUpperCase
if (answer5.toUpperCase() === 'OBAMA' || answer5.toUpperCase() === 'BARACK OBAMA' || answer5.toUpperCase() === 'BARACK') {
correct += 1;
}
// Output results
// LINE 46 BELOW
//missing two + operators between playerName and string
//and one between correct variable and string
//this was causing the ) error
//also deleted the strange v after the comma in the first string
//also added space before the word out in last string
document.write('<p>Well done, ' + playerName + ' you got ' + correct + ' out of 5 correct</p>');
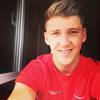
Jay Valentine
Courses Plus Student 4,389 PointsGreat comment Marcus Parsons , thanks for the corrections, must of overlooked them, my bad, also why did it say missing ')' when it wasnt, it was the '+' characters and a few .toUpperCase syntaxes?
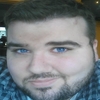
Marcus Parsons
15,719 PointsJavaScript errors are rarely, if ever, actually helpful. You'll learn that as you progress. It's because the parser is a machine and doesn't know the logic behind the concatenation you're making. It thinks that the command should end right after playerName because there are no other operators immediately following playerName to signify that there is further concatenation. Therefore, the parser will say that it is missing a ) to end the write command at that location.
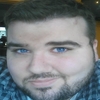
Marcus Parsons
15,719 PointsAlso, the toUpperCase calls had nothing to do with the parse error that was preventing the code from running. These were logic errors. They wouldn't allow for the correct answer to be counted. For example, if you put "shark" into the input, it is converted into "SHARK" by your program but it was then being compared to "shark" and "shark" of course doesn't equal "SHARK" so it was counting the question wrong. And a few other missing things involving that method, as well.
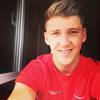
Jay Valentine
Courses Plus Student 4,389 PointsLaura Kyle Shed some light hmm?
Jay Valentine
Courses Plus Student 4,389 PointsJay Valentine
Courses Plus Student 4,389 PointsMy program wont run because of this mysterious missing closing bracket.. i cant see where i am going wrong here?