Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial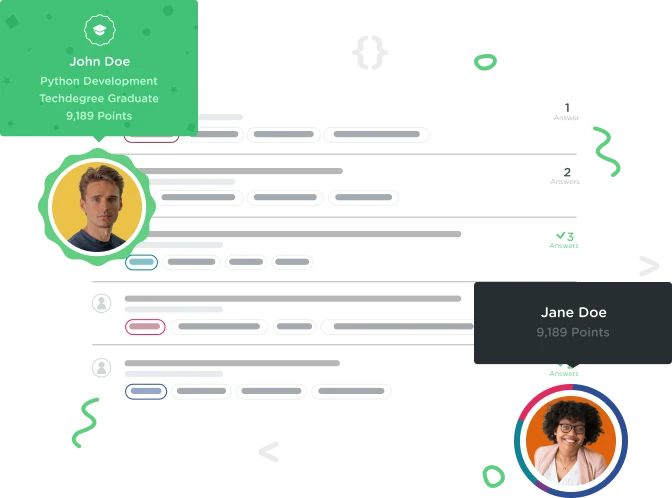
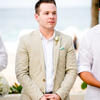
Michael German
Full Stack JavaScript Techdegree Student 2,017 PointsUncaught SyntaxError: Unexpected token +=
My code works fine for this challenge, but only if I don't use the += operator. If I use the += operator to add scores to my FinalScore variable I get a syntax error that appears in both Chrome and Safari. I would like to be able to use the += operator to shorten my code so wondering if anyone knows why it's not working for me?
1 Answer
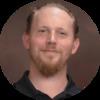
Michael Davis
Courses Plus Student 12,508 PointsIn your code you initialize the variable
// Start game with player at a score of 0
var FinalScore = 0;
Then, in the logic of your look, in initialize a scoped variable with the same name.
if ( parseInt(QuestionOne) === 2 ) {
var FinalScore = FinalScore + 1;
}
So, in the statement you are not using the global variable FinalScore
, you're using the statement's scoped variable. Instead, try:
if ( parseInt(QuestionOne) === 2 ) {
FinalScore += 1;
}
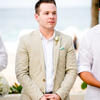
Michael German
Full Stack JavaScript Techdegree Student 2,017 PointsThank you! You were right I should not have been adding var
to the front of my FinalScore
variable. It caused the loop to use the scoped variable and not the global variable.

andren
28,558 PointsWhile the conclusion is correct (the var keyword was causing the issue) your explanation is actually slightly off.
In JavaScript (unlike many other languages) var
does not create variables scoped to local code blocks (that is what the let
keyword is for). Variables are either scoped to a function (if declared within a function) or global. Since no function is used here all of the variables are global, both the first one declared outside the if statements and the ones inside the if statements. So you don't end up with multiple variables with the same name as you imply, but instead you end up recreating the same variable (with the same scope) over and over again.
When you define a variable you have to either leave it empty or set it to a value with =
. The +=
operator is not supported when declaring variables. That is why using that operator resulted in a syntax error.
Also I realize this is likely a typo on your end but for clarity's sake (Since Michael German is just starting out), I'll point out that there is no loop used in this code. An if statement is a type of conditional statement, not a loop.
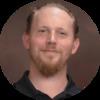
Michael Davis
Courses Plus Student 12,508 PointsCorrect, my explainatinon was poorly worded and did not explain the mechanism of hoisting and overwriting the global. I guess that's what I get for replying before sleeping, lol!

andren
28,558 PointsNo worries, I've made my share of half-a-sleep rushed posts too that I'm not too happy with after the fact. I just wanted to clarify it a bit in order to avoid Michael German ending up misunderstanding some of these topics.
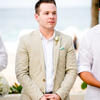
Michael German
Full Stack JavaScript Techdegree Student 2,017 PointsThanks for clarifying and further explanation from you both.
Michael German
Full Stack JavaScript Techdegree Student 2,017 PointsMichael German
Full Stack JavaScript Techdegree Student 2,017 PointsHere is my code: