Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial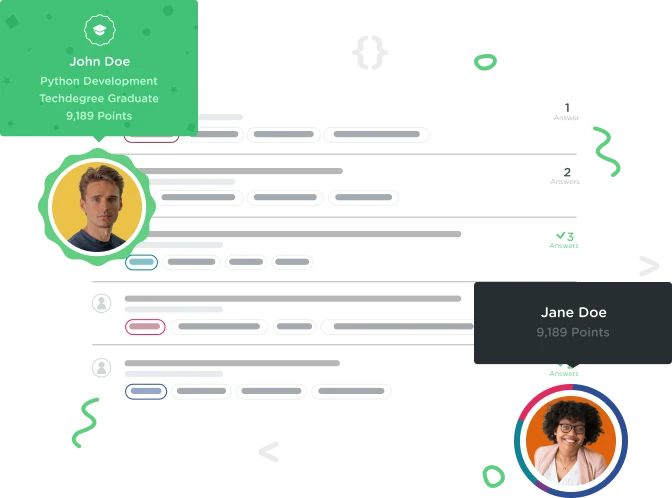

Andrew Serrano
13,755 PointsUncaught TypeError: Cannot read property '0' of undefined
Hello guys I can't find out why I keep getting this error in my console. I've watched this video numerous of times and also the previous video to find my error but for some reason I just can't find what I am doing wrong. I get this error when I try to use the Onclick event for my buttons.
Also if someone could explain what this error means it would greatly help. Thank you guys so much! :)
APP.JS
var playlist = new Playlist();
var hereComesTheSun = new Song("Here Comes the Sun", "The Beatles", "2:54");
var walkingOnSunshine = new Song("Walking on Sunshine", "Katrina and the Waves", "3:43");
playlist.add(hereComesTheSun);
playlist.add(walkingOnSunshine);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
var playButton = document.getElementById("play");
playButton.onclick = function () {
playlist.play();
playlist.renderInElement(playlistElement);
}
var nextButton = document.getElementById("next");
nextButton.onclick = function () {
playlist.next();
playlist.renderInElement(playlistElement);
}
var stopButton = document.getElementById("stop");
stopButton.onclick = function () {
playlist.stop();
playlist.renderInElement(playlistElement);
}
PLAYLIST.JS
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(song) {
//adds new songs to the playlist
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.song[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.song[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
}
this.play();
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML = "";
for (var i = 0; i < this.songs.length; i++) {
list.innerHTML += this.songs[i].toHTML();
}
};
SONG.JS
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
this.isPlaying = false;
}
Song.prototype.play = function() {
this.isPlaying = true;
};
Song.prototype.stop = function() {
this.isPlaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if (this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};

Andrew Serrano
13,755 PointsHi Mark thanks for getting back to me :).
It's telling me line 12 and 17 of the playlist.js is causing the error which is this code right down below
Line 12:
var currentSong = this.song[this.nowPlayingIndex];
and Line 17 is the exact same code.
It's referring back to the Playlist function that was declared at the top of playlist.js, just not to sure why its not liking 0

Andrew Serrano
13,755 PointsThank you so much jimmy! I cant believe one letter was stopping my whole script haha. Thank you so much for taking the time to help me out and the script works! :)
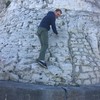
Jimmy Saul
9,851 PointsNo problem, the benefit of a fresh pair of eyes can't be underestimated! Pleased to hear it's working for you now
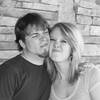
Cale Matteson
11,303 PointsHello Everyone!
Jimmy, since you have provided an answer, I suggest you may copy and paste your answer into the "Add an answer" section. That way people on the video with this problem know this is an answer to the question.
Keep up the good work! Cale
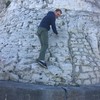
Jimmy Saul
9,851 PointsThanks Cale that's a good point, I've now moved the comment to an answer.
1 Answer
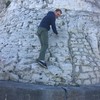
Jimmy Saul
9,851 PointsHi Andrew,
I think this.song on this line needs to have an 's' on the end to be this.songs :)
var currentSong = this.songs[this.nowPlayingIndex];
miikis
44,957 Pointsmiikis
44,957 PointsHey Andrew,
The console error should tell you what line the error is occurring on. You should check.