Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial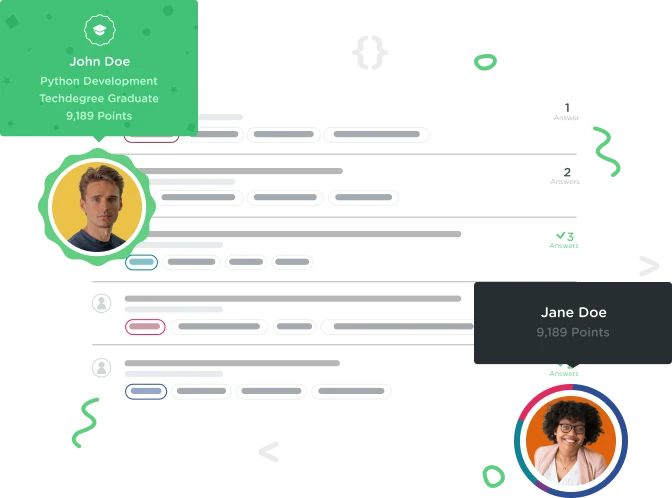
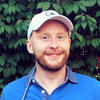
Michael Kilcorse
Full Stack JavaScript Techdegree Graduate 17,643 PointsUncaught TypeError: Cannot read property "drawHTMLBoard" of undefined
I've been working on this for awhile, and maybe I'm overlooking something simple, but I've gone back and have worked through the videos again and checked each file line by line and I still can't understand why I can't access the drawHTMLBoard property once it's initialized in the gameStart() method. I would appreciate any help or pointers that can get this working for me. Thank You!
class Board {
constructor(){
this.rows = 6;
this.columns = 7;
this.spaces = this.createSpaces();
}
/**
* Generates 2D array of spaces
* @return {Array} An array of space objects
*/
createSpaces(){
const spaces = [];
for(let x = 0; x < this.columns; x++){
const col = [];
for (let y = 0; y < this.rows; y++){
const space = new Space(x, y);
col.push(space);
}
spaces.push(col);
}
return spaces;
}
drawHTMLBoard(){
for(let column of this.spaces){
for(let space of column){
space.drawSVGSpace();
}
}
}
}
class Game {
contructor(){
this.board = new Board();
this.players = this.createPlayers();
this.ready = false;
}
/**
* returns active player
* @returns {Object} player - the active player
*/
get activePlayer(){
return this.players.find(player => player.active);
}
/**
* Creates two player objects
* @returns {Array} an array of two player objects
*/
createPlayers(){
const players = [new Player("Player 1", 1, "#e15258", true),
new Player("Player 2", 2, "#e59a13")];
return players;
}
/*
* gets game ready for play
*/
startGame(){
this.board.drawHTMLBoard();
this.activePlayer.activeToken.drawHTMLToken();
this.ready = true;
}
}
1 Answer

KRIS NIKOLAISEN
54,972 PointsUnder class Game you have
contructor(){
it should be
constructor(){
Michael Kilcorse
Full Stack JavaScript Techdegree Graduate 17,643 PointsMichael Kilcorse
Full Stack JavaScript Techdegree Graduate 17,643 PointsWow, do I feel silly, thank you for reading over it and helping!