Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial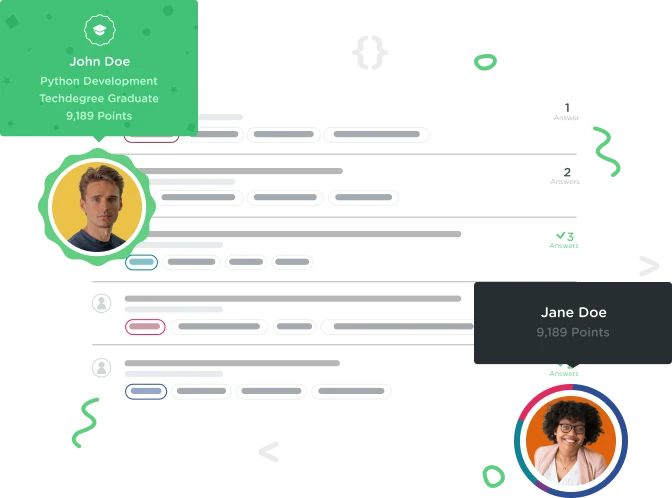

Kenton Hubner
6,314 PointsUncaught TypeError: Cannot read property 'querySelector' of undefined
I don't know if it is a grammatical error causing this or my wonderful network (at work :D) Below is my code.
var taskInput = document.getElementById("new-task"); // new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //ul #incomplete-tasks
var completeTasksHolder = document.getElementById("complete-tasks"); // ul #complete-tasks
//New-task list item
var createNewTaskElement = function(taskString){
var listItem = document.createElement("li");
//input (checkbox)
var checkbox = document.createElement("input");//checkbox
// label
var label = document.createElement("label"); // label
// input (text)
var editInput = document.createElement("label"); // label
// button.edit
var editButton = document.createElement("button"); // button
//button.delete
var deleteButton = document.createElement("button"); // button
//Each element, needs modifying
// Each element needs appending
listItem.appendChild(checkbox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
// add new task
var addTask = function(){
console.log("add task...");
//create a new list item with the text from #new-task:
var listItem = createNewTaskElement("Some new Task");
// Append listItem to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
;
}
// edit existing task
var editTask = function(){
console.log("edit task...");
// when the dit button is pressed:
//if the class of the parent is .edit-mode
//Switch from .edit-mode
// label text become the input's value
// elese
//switch to .editMode
//input value becomes the labels text
//toggle .editMode on the parent
}
// delete an existing task
var deleteTask = function() {
console.log("delete task...");
//when the delet button is pressed
// remove parent list itemfrom the ul
}
// mark a task as complete
var taskComplete = function() {
console.log("task complete...");
//when the checkbox is checked
//append the task list item to the #completed-tasks
}
// mark a task as incomplete
var taskIncomplete = function() {
console.log("task incomplete ...");
//when the checkbox is unchecked
//append this task to list item to the #incomplete-tasks
}
var bindTaskEvents = function( taskListItem, checkboxEventHandler) {
console.log("bind list item...");
// select list items's children
var checkbox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind the editTask to edit button
editButton.onclick = editTask;
//bind the delete task to the delete button
deleteButton.onclick = deleteTask;
//bind taskComplete to checkbox
checkbox.onchange = checkboxEventHandler;
}
// Set the click handler to the addTask function
addButton.onclick = addTask;
// cycle over the incompletetaskholder ul list items
// for each list item
for(var i=0; 1 < incompleteTasksHolder.children.length; i++){
// bind events to listItems Children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskComplete);
}
// cycle over the completetaskholder ul list items
// for each list item
for(var i=0; 1 < completeTasksHolder.children.length; i++){
// bind events to listItems Children (taskCompleted)
bindTaskEvents(completeTaskHolder.children[i], taskIncomplete);
}
1 Answer
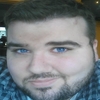
Marcus Parsons
15,719 PointsHey Kenton,
It looks like you have the number 1 in the conditional part of both of your for loops at the bottom that are binding the task events. Changing the 1 to i should fix your error:
// cycle over the incompletetaskholder ul list items
// for each list item
//changed 1 < to i <
for(var i=0; i < incompleteTasksHolder.children.length; i++){
// bind events to listItems Children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskComplete);
}
// cycle over the completetaskholder ul list items
// for each list item
//changed 1 < to i <
for(var i=0; i < completeTasksHolder.children.length; i++){
// bind events to listItems Children (taskCompleted)
bindTaskEvents(completeTaskHolder.children[i], taskIncomplete);
}