Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial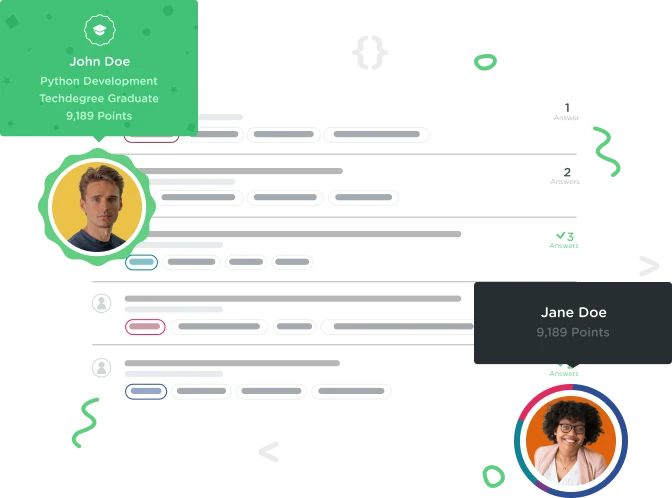

Jose Sanchez
7,849 PointsUncaught TypeError: Cannot read property 'querySelector' of undefined + console shows add task when editing???
// Problem: user interaction doesnt provide desired results.
// Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first-button
var incompleteTasksHolder = document.getElementById("incomplete-tasks");//incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); // completed-tasks
//Add a new task
var addTask = function () {
console.log("Add task..");
//When the button is pressed
//Creat a new list item with the text from #new-task:
//input checkbox
//label
//input (text)
//button.edit
//button.delete
//Each elements, needs to be modified and appended
}
//Edit an existing task
var editTask = function (){
console.log("Edit task...");
//When the Edit button is pressed
//If the class of the parent is .editMode
//Switch from .editMode
//label text become the input's value
//else
//Switch to .editmode
//input value becomes the label's text
//Toggle .editMode on the parent
}
//Delete an existing task
var deleteTask = function (){
console.log("delete task...");
//When the delete button is pressed
//Remove the parent list item from the unordered list (ul)
}
//Mark a task as complete
var taskCompleted = function(){
console.log("Task complete..");
//When the checkbox is checked
//Append the task list item to the #completed-tasks
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete..");
//When the checkbox is unchecked
//Append the task list item to the #complete-tasks
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list items");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit") ;
var deleteButton = taskListItem.querySelector("button.delete") ;
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to the checkbox
checkBox.onchange = addTask;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over the incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
for(var i = 0; i < incompleteTasksHolder.children.length; i++){
//bind events to list item's children (taskIncompleted)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
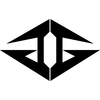
Julian Gutierrez
19,201 PointsEdited your code's markdown.
John Coolidge
12,614 PointsCan you tell us what line of code is in error? The Dev Tools should point you to what line the error occurred on. Also, could you paste your HTML too? It might be that the JavaScript can't find the element in the DOM which may mean that you've labeled something in your HTML incorrectly.
Also, your code needs to be changed here:
//bind checkBoxEventHandler to the checkbox
checkBox.onchange = addTask;
It should look like this:
//bind checkBoxEventHandler to the checkbox
checkBox.onchange = checkBoxEventHandler;
John
Jose Sanchez
7,849 PointsJose Sanchez
7,849 PointsIt didnt seem to add my code in code blocks :( anyways it may be easier to look at it in my workspace https://teamtreehouse.com/workspaces/12183262