Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial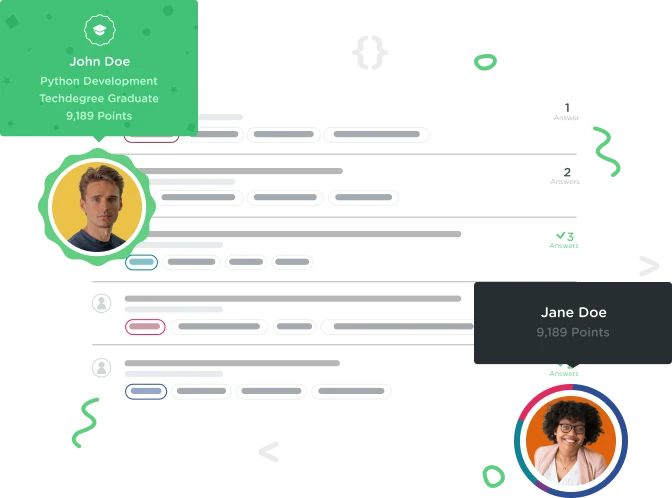
Nick Prodromou
12,824 PointsUncaught TypeError: Cannot read property 'text' of undefined
Okay so, full transparency here.. I didn't feel up to writing the program myself, so I've copied Andrew's solutions and commented them in an attempt to better understand what is going on.. because OOJS is really not sticking with me in this course...
but I've been debugging for ages, and I can't workout why.. even with copying Andrews code, I still get this error..
quiz_ui.js
var QuizUI = {
displayNext: function () {
if (quiz.hasEnded()) { //checks if the quiz has ended
this.displayScore(); //displays score
} else {
this.displayQuestion(); // gets the text from the current question and inserts into #question
this.displayChoices(); //displays what question we are currently on
this.displayProgress();
}
},
displayQuestion: function() {
this.populateIdWithHTML("question", quiz.getCurrentQuestion().text);
},
displayChoices: function() {
var choices = Quiz.getCurrentQuestion().choices;
for (var i = 0; i < choices.length; i++) {
this.populateIdWithHTML("Choice" + choices[i]);
this.guessHandler("guess" + i, choices[i]);
}
},
displayScore: function() {
var gameOverHTML = '<h1>Game Over</h1>';
gameOverHTML += "<h2> Your score is: " + quiz.score + "</h2>";
this.populateIdWithHTML( "quiz", gameOverHTML );
},
populateIdWithHTML: function(id, text) {
var element = Document.getElementById(id);
element.innerHTML = text;
},
guessHandler : function(id, guess) {
var button = document.getElementById(id);
button.onclick = function() {
quiz.guess(guess);
QuizUI.displayNext();
}
},
displayProgress: function() {
var currentQuestionNumber = quiz.currentQuizIndex + 1;
this.populateIdWithHTML("progress","Question " + currentQuestionNumber + " of " + quiz.questions.length);
},
}
app.js
// Create questions (stored in an array
var questions= [new Question("Who was the first president of the United States?"["George Washington", "Thomas Jefferson"],"George Washington"),
new Question("What is the answer to the ultimate question of Life, the Universe and Everything?"["pi","42"], "42" )
];
//create quiz
var quiz = new Quiz(questions);
//Display quiz
QuizUI.displayNext();
quiz.js
function Quiz(questions) { // takes an array of questions
this.score = 0; //starts at zero
this.questions = 0; //starts at 0
this.currentQuizIndex = 0;
}
Quiz.prototype.guess = function(answer) { //takes in a string of answer, checks against current question
if(this.getCurrentQuestion().isCorrectAnswer(answer)){ //if correct, add to score
this.score++;
}
this.currentQuestionIndex++; //move current index forward by 1
};
Quiz.prototype.getCurrentQuestion = function() { //return current question and the index
return this.questions[this.currentQuestionIndex];
};
Quiz.prototype.hasEnded = function() { //the quiz has ended, if the number is the same length or greater than, then the game is over
return this.currentQuestionIndex >= this.questions.length;
};
question.js
function Question (text, choices, answer) { //constructor function
this.text = text;
this.choices = choices; //Array with 2 items, wrong answer and correct answer
this.answer = answer;
}
Question.prototype.isCorrectAnswer = function (choice) { //method
return this.answer === choice;
};
2 Answers

Amy Norris
9,718 PointsYou may just be missing a couple commas in your app.js, questions array:
var questions = [
new Question("Who was the first president of the United States?", ["George Washington", "Thomas Jefferson"],"George Washington"),
new Question("What is the answer to the ultimate question of Life, the Universe and Everything?", ["pi","42"], "42" )
];

Matt Juszczyk
12,824 PointsThere was a missing common in his Question instantiation, but the error here is a mismatch of property names: In quiz.js, you have the property
this.currentQuizIndex
and your method
Quiz.prototype.getCurrentQuestion
is trying to use the property
this.currentQuestionIndex