Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial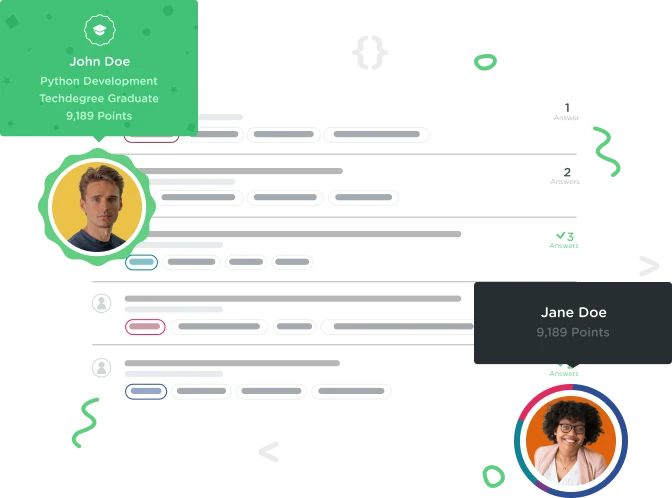

Greg Schudel
4,090 PointsUncaught TypeError: Cannot read property 'value' of null
I cannot add a li
element to the ul
element. Whenever I try, I get the following error on my console:
Uncaught TypeError: Cannot read property 'value' of null at HTMLButtonElement.addItemButton.addEventListener (app.js:51)
Here is my Code. I also have other questions in the code. Very confused on this one.
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const addItemInput = document.querySelector('descriptionInput.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
toggleList.addEventListener("click", () => {
if (listDiv.style.display === 'none') {
toggleList.textContent = 'Hide list'; /*this changes the actual text in the button*/
listDiv.style.display= 'block';
} else {
toggleList.textContent = 'Show list'; /*this changes the actual text in the button*/
listDiv.style.display= 'none';
}
});
descriptionButton.addEventListener("click", () => {
descriptionP.innerHTML= descriptionInput.value + ':';
});
//BELOW IS THE PART I'M GETTING THE MOST CONFUSED ABOUT!!
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
//not quite clear on why he is using [0] on this. I know it's 'like' an array, but not really.
//If so, what exactly is it called?
let li = document.createElement('li');
li.textContent = addItemInput.value;
//why is .the word `. value` used here? Why not just addItemInput?
//This is where I'm getting the error, it shows up on mine, but not on the instructor's, why?
ul.appendChild(li);
//okay? why is the word node not used for the ul? Also, why is li not have quotations on it?
});
1 Answer
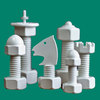
Steven Parker
229,745 PointsYou have the wrong selector.
On line 6, you call querySelector using 'descriptionInput.addItemInput'
as the selector, but it should be 'input.addItemInput'
. Since the query you used cannot be found in the document, it stores null in the variable instead of a reference to the element.
As for your other questions:
let ul = document.getElementsByTagName('ul')[0];
//not quite clear on why he is using [0] on this. ...what exactly is it called?
getElementsByTagName returns an HTMLCollection which can be indexed like an array. It would include all 'ul' elements on the page. Putting "[0]
" after it selects just the first element of that type.
li.textContent = addItemInput.value;
//why is .the word . value
used here? Why not just addItemInput?
By itself, addItemInput represents the whole element. Adding ".value
" to it selects just the contents of the "value" attribute of that element (which would be what the user typed in the box).
ul.appendChild(li);
//why is the word node not used for the ul? Also, why is li not have quotations on it?
Both ul
and li
are variables that were assigned a few lines above, and they both represent elements. And since they are variables, you would not put quotes around their names.