Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial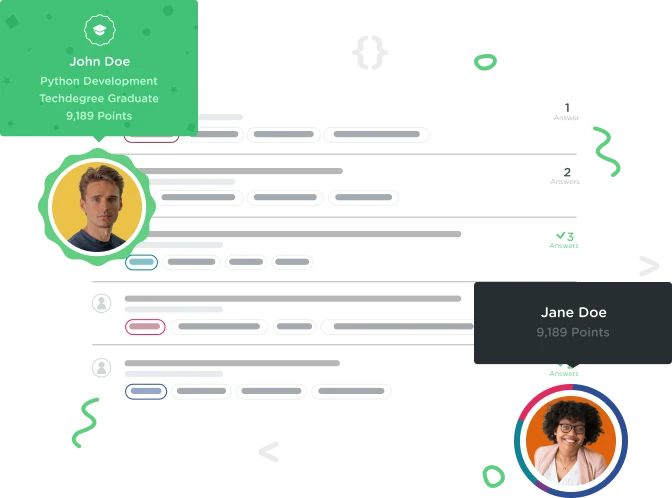

Sirin Srikier
7,671 PointsUncaught TypeError: Cannot set property 'innerHTML' of null
I'm not sure why I'm getting this error. Please help. https://w.trhou.se/klufprtbgv
var QuizUI = {
displayNext: function() {
if (quiz.hasEnded()) {
this.displayScore();
} else {
this.displayQuestion();
this.displayChoices();
this.displayProgress();
}
},
displayQuestion: function() {
this.populateIdWithHTML("question", quiz.getCurrentQuestion().text);
},
displayChoices: function() {
var choices = quiz.getCurrentQuestion().choices;
for(var i=0; i < choices.length; i++) {
this.populateIdWithHTML("choice", i, choices[i]);
this.guessHandler("guess" + i, choices[i]);
}
},
displayScore: function() {
var gameOverHTML = "<h1>Game Over</h1>";
gameOverHTML += "<h2> Your score is: " + quiz.score + "</h2>";
this.populateIdWithHTML("quiz", gameOverHTML);
},
populateIdWithHTML: function(id, text) {
var element = document.getElementById(id);
element.innerHTML = text;
},
guessHandler: function(id, guess) {
var button = document.getElementById(id);
button.onclick = function() {
quiz.guess(guess);
QuizUI.displayNext();
}
},
displayProgress: function() {
var currentQuestionNumber = quiz.currentQuestionIndex + 1;
this.populateIdWithHTML("progress", "Question" + currentQuestionNumber + " of " + quiz.questions.length);
}
};
1 Answer
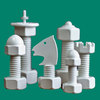
Steven Parker
231,275 PointsThe snapshot is very helpful and you don't need to paste in code separately with it.
So here's what's happening:
- As the program starts, app.js calls "
QuizUI.displayNext()
" on line 12. - Then in quiz_ui.js, eventually line 7 calls "displayChoices()".
- Then line 18 calls "
populateIdWithHTML("choice", i, choices[i])
" (when "i" is 0) - Then line 28 calls "
getElementById
" passing the argument "choice" - but there is no element in the HTML with the id "choice", so the result is null
- on line 29, attempting to set a property on null causes the error
Also note that on line 18, "populateIdWithHTML
" is passed 3 arguments, but it is defined (on line 27) to take only 2.
Perhaps you meant to concatenate the index to the name on line 18?
this.populateIdWithHTML("choice" + i, choices[i]);