Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial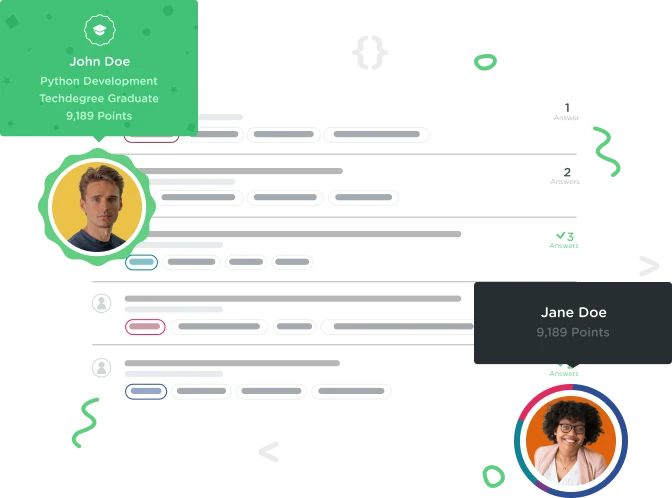
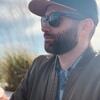
C.J. O'Leary
Front End Web Development Techdegree Graduate 20,330 PointsUncaught TypeError: this.board.drawHTMLBoard is not a function
Getting the error above when I click to start the game and not sure why. I even get the error if I copy and paste the code from the solution. Any help would be much appreciated!
/**
* Listens for click on `#begin-game` and calls startGame() on game object
*/
const game = new Game();
document.getElementById('begin-game').addEventListener('click', function() {
this.style.display = 'none';
document.getElementById('play-area').style.opacity = '1';
game.startGame();
});
class Game {
constructor() {
this.board = new Board();
this.players = this.createPlayers();
this.ready = false;
}
/**
* Returns active player.
* @return {Object} player - The active player.
*/
get activePlayer() {
return this.players.find(player => player.active);
}
/**
* Creates two player objects
* @return {array} An array of two player objects.
*/
createPlayers() {
const players = [new Player('Player 1', 1, '#e15258', true),
new Player('Player 2', 2, '#e59a13')];
return players;
}
/**
* Initializes game.
*/
startGame(){
this.board.drawHTMLBoard();
this.activePlayer.activeToken.drawHTMLToken();
this.ready = true;
}
}
class Board {
constructor() {
this.rows = 6;
this.columns = 7;
this.spaces = this.createSpaces();
}
/**
* Generates 2D array of spaces.
* @return {array} An array of space objects
*/
createSpaces() {
const spaces = [];
for (let x = 0; x < this.columns; x++) {
const col = [];
for (let y = 0; y < this.rows; y++) {
const space = new Space(x, y);
col.push(space);
}
spaces.push(col);
}
return spaces;
}
/**
* Draws associated SVG spaces for all game spaces.
*/
drawHTMLBoard() {
for (let column of this.spaces) {
for (let space of column) {
space.drawSVGSpace();
}
}
}
}
class Space {
constructor ( x, y ) {
this.x = x;
this.y = y;
this.id = `space${x}-${y}`;
this.token = null;
this.diameter = 76;
this.radius = this.diameter/2;
}
drawSVGSpace() {
const svgSpace = document.createElementNS("http://www.w3.org/2000/svg", "circle");
svgSpace.setAttributeNS(null, "id", this.id);
svgSpace.setAttributeNS(null, "cx", (this.x * this.diameter) + this.radius);
svgSpace.setAttributeNS(null, "cy", (this.y * this.diameter) + this.radius);
svgSpace.setAttributeNS(null, "r", this.radius - 8);
svgSpace.setAttributeNS(null, "fill", "black");
svgSpace.setAttributeNS(null, "stroke", "none");
document.getElementById("mask").appendChild(svgSpace);
}
}