Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial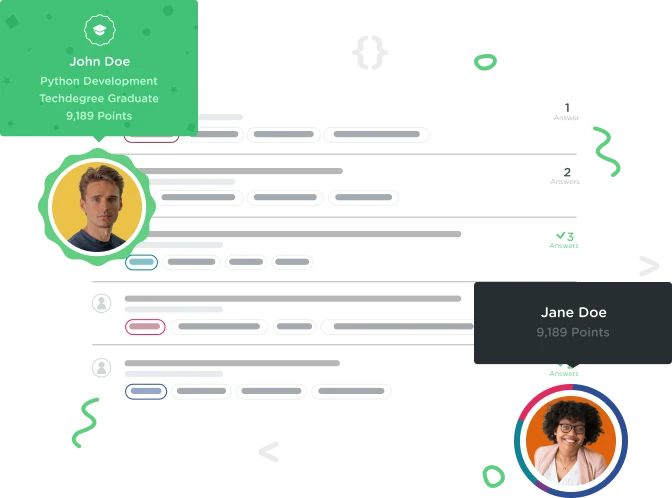

Shreyas Sreenivasa
7,804 PointsUndefined is displayed instead of the question
I created a quiz but for some reason it says undefined instead of the question itself.
My code:
var questonsAndAnser = [['What is 1 + 1?', 2],
['How many states are there in India?', 29],
['How many planets are there in Solar System', 8]];
var question;
var correctAnswers = 0;
var answer;
var response;
var html = "";
var correct = [];
var wrong = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr){
var listHtml = '<ol>';
for (var i = 0; i < arr.length; i += 1) {
listHtml += '<li>' + arr[i] + '</li>';
}
listHtml += '</ol>';
}
for (var i = 0; i < questonsAndAnser.length; i += 1) {
question = questonsAndAnser[i][0];
answer = questonsAndAnser[i][1];
response = parseInt(prompt(question));
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
html = 'You got' + correctAnswers + 'question(s) right.'
html += '<h2> You got these questions correct: </h2>';
html += '<p>' + buildList(correct); '</p>'
html += '<h2> You got these questions wrong </h2>';
html += '<p>' + buildList(wrong) + '</p>';
print(html);
3 Answers
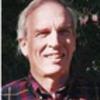
jcorum
71,830 PointsI had a bit more time. So this time I put it into a page in my IDE and ran it through my debugger.
Other than the items I mentioned earlier, the real problem is that your buildList() function wasn't returning the lists it had built.
Here's the page with comments showing the changes:
<body>
<div id="output"></div>
<script>
var questonsAndAnser = [['What is 1 + 1?', 2],
['How many states are there in India?', 29],
['How many planets are there in Solar System', 8]];
var question;
var correctAnswers = 0;
var answer;
var response;
var html = "";
var correct = [];
var wrong = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr){
var listHtml = '<ol>';
for (var i = 0; i < arr.length; i += 1) {
listHtml += '<li>' + arr[i] + '</li>';
}
listHtml += '</ol>';
return listHtml; //added a return statement
}
for (var i = 0; i < questonsAndAnser.length; i += 1) {
question = questonsAndAnser[i][0];
answer = questonsAndAnser[i][1];
response = parseInt(prompt(question));
if (response == answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
html = 'You got ' + correctAnswers + ' question(s) right.'; //added spaces and final ;
html += '<h2> You got these questions correct: </h2>';
html += '<p>' + buildList(correct) + '</p>'; //moved ; to end, and replaced it with a +
html += '<h2> You got these questions wrong </h2>';
html += '<p>' + buildList(wrong) + '</p>';
print(html);
</script>
</body>
</html>
I got the following output:

Salma Alshabib
2,957 Pointsyou may need to put the last part of your code (from the for loop to just before calling the print function) inside a function and call it when the page loads.
Also did you use the defer attribute in the script tag for this javascript in your HTML? sometimes getElementById returns null because the javascript code loads before the element you're trying to get. The defer attribute means that the javascript will not start loading before the HTML is completely loaded and so it solves this issue.

Shreyas Sreenivasa
7,804 PointsNo, I didn't add the define attribute. And if I move the for loop just before the print statement then nothing comes out as output.

Salma Alshabib
2,957 PointsCan you post your HTML? The problem might be there.

Shreyas Sreenivasa
7,804 PointsSalma i just got the answer thank you though. I had forgotten to put return. jcorum solved my problem.
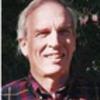
jcorum
71,830 PointsThere are also a couple of typos:
html = 'You got' + correctAnswers + 'question(s) right.' //need a semi-colon at the end of the statement
html += '<h2> You got these questions correct: </h2>';
html += '<p>' + buildList(correct); '</p>' //replace the ; with a +, and then put a semi-colon at the end of the statement
html += '<h2> You got these questions wrong </h2>';
html += '<p>' + buildList(wrong) + '</p>';

Shreyas Sreenivasa
7,804 PointsThe output is still the same.