Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial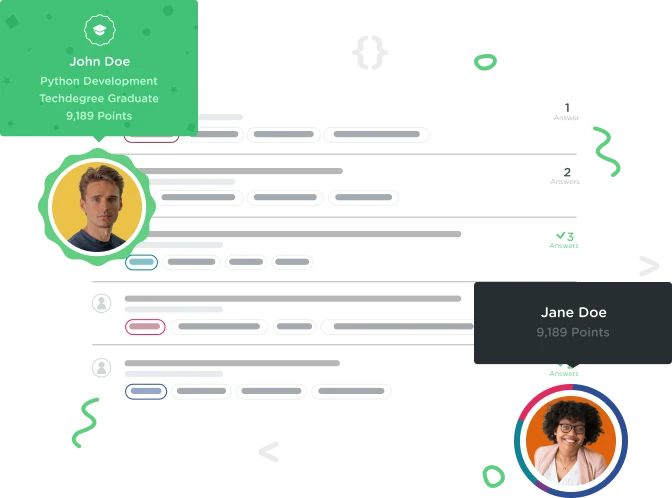

Hassan Noueilaty
3,713 Points'undefined' is not a function (evaluating 'document.getElementsById('rainbow')
Hello!
I'm having some trouble on this challenge task. Any help would be greatly appreciated.
Thanks!
let listItems = document.getElementsById('rainbow');
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
let listItems = document.getElementsById('rainbow');
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
10 Answers
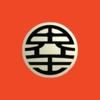
Aj Broman
2,668 PointsAs I commented above, it's document.getElementById('');
However this exercise is asking for multiple things, your best bet here is to target by tagname
document.getElementsByTagName('li');
This will fix your errors, make sure to check all the different methods available to you :) GL learning

Hassan Noueilaty
3,713 PointsThat worked! I'm still a little unsure why but I'll rewatch the videos.
Thanks for your help.
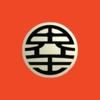
Aj Broman
2,668 Pointslet listItems = document.getElementsByTagName('li'); // will give listItems all of your lis const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) { listItems[i].style.color = colors[i]; //here is where it's looping through your lis with [i] and applying .style.color }
I'm pretty new to this stuff too, and I'm actually mid-way into DOM Manipulation myself

Christopher Kehl
18,180 Pointsgreat, it worked for me too. That was a little confusing treehouse.
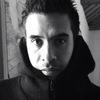
Jose Gutiérrez
9,370 PointsBesides the typo, you are trying to get many elements (li) from a HTML element so the List Items[i] will be undefined because listItems only contains one element: the ul. Try with getElementsByTagName('li');
let listItems = document.getElementsByTagName('li'); console.log(listItems); const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(let i = 0; i < colors.length; i ++) {
console.log(listItems[i]);
listItems[i].style.color = colors[i];
}
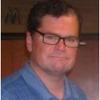
Mark Wilkowske
Courses Plus Student 18,131 Points.getElementById('rainbow') This returns an element object and as such you can access the children of the UL - the li's - with listItems.children.
for(var i = 0; i < colors.length; i ++) {
listItems.children[i].style.color = colors[i];
}
If you try that in CodePen or in Chrome it works but will not in the Treehouse code challenage compiler.
.getElementsByTagName('li') passes challenge because the question asks to select only the li's, not the whole ul object.

dallas kelley
7,884 PointsWhile document.getElementsByTagName('li'); works for this particular problem it wouldn't work if you had more than one list that had different IDs. For example document.getElementsByTagName('li'); would select all <li> tags in a list that may not need to be selected where as document.querySelectorAll("ul#rainbow > li"); will only select <li> tags inside the UL with the ID: rainbow.
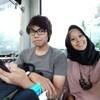
Salma Yarista
4,595 Pointsthanks, it works

Arturo Perez
Front End Web Development Techdegree Student 12,902 PointsLooks like it's a small typo. It's document.getElementById("");
Instead of
document.getElementsById("");
It's element and not elements.

Hassan Noueilaty
3,713 PointsThanks for the replies. I removed the 's' and made it singular. Unfortunately, I still encountered an error:
Bummer! There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'listItems[i].style')

Daven Hietala
8,040 Pointsdallas kelley, your solution worked for me. Thank you! @Hassan Noueilaty, perhaps you can change the best answer to dallas kelley considering her answer is the actual solution to your question.
Happy coding!

Binod Regmi
7,038 PointsTRY THIS!
var listItems;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
listItems = document.getElementsByTagName('li')
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
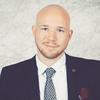
Roald Jurrian Kamman
Front End Web Development Techdegree Graduate 15,544 PointsgetElementsByTagName('li') worked for me but I was also confused as I used getElementsByTagName('ul') and that provided the same error. Can anyone explain why using <ul> tag didn't work?
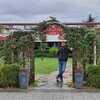
Hassan Al Manawy
24,859 PointsActually, the twist here is not with the code itself as this challenge could be addressed in multiple ways as shown below. It's just that you should declare the var listItems and your code before the "for loop", otherwise you would get this error.
In other words, the system exits the loop and then works on your code which has some unidentified objects. However, I think it should work fine with other text editors :)
Bummer: There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'listItems[i]').
Correct Answers: var listItems = document.querySelector('#rainbow').children; var listItems = document.getElementById('rainbow').children; var listItems = document.getElementsByTagName('li');
just write any of the correct answers anywhere above the for loop (to be more specific; line 1 by declaring value within or anywhere between lines 1-3).
Hope that helps.
Regards, H
Aj Broman
2,668 PointsAj Broman
2,668 PointsThere can only be one unique ID. Not plural:
document.getElementById();