Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial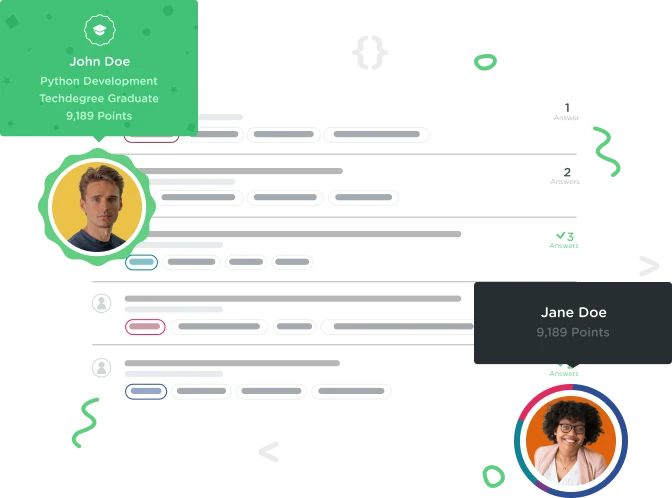

Oğulcan Girginc
24,848 Pointsundefined method `push' for nil:NilClass (NoMethod Error)
def create_list
print "What is the list name?: "
name = gets.chomp
hash = { name: name, items: Array.new }
return hash
end
def add_list_item
print "What is the item called?: "
item_name = gets.chomp
print "How much?: "
quantity = gets.chomp.to_i
hash = { name: item_name, quantity: quantity }
return hash
end
list = create_list()
puts list.inspect
list['items'].push(add_list_item())
puts list.inspect
When I use
hash = { name: name, items: Array.new }
instead of
hash = { name: name, "items" => Array.new }
I am getting an error. Does anyone know why?
3 Answers
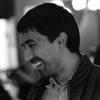
Martin Cornejo Saavedra
18,132 PointsThe error is here:
list['items'].push(add_list_item())
#Should be
list[:items].push(add_list_item())
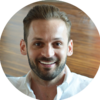
Alan Matthews
10,161 PointsI believe it would depend upon on what the hash key is. In your above code you made hash = { name: name, items: Array.new }
a hash, where items:
is a symbol that is the key and a new Array is the value. Newer versions of Ruby are deprecating the old hash syntax in favor of using symbols as keys. Docs here: http://rubylearning.com/satishtalim/ruby_hashes.html
If you had made items
a string in the create_list
method, then your original syntax list['items'].push(add_list_item())
should work if I'm not mistaken.
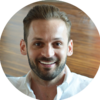
Alan Matthews
10,161 PointsYou also don't need to specifically return anything in a Ruby method. It will always return the last expression evaluated.
Oğulcan Girginc
24,848 PointsOğulcan Girginc
24,848 PointsSo if I want to use
name: item_name
I always need to use
list[:name]
Am I correct?