Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial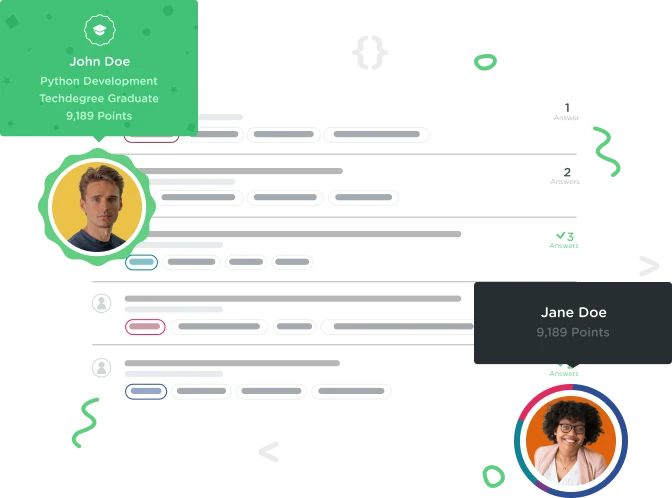
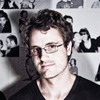
Ian Horner
22,587 Pointsundefined method `user_friendships' for nil:NilClass – Creating the Friendship
Im stuck in "Creating the Friendship" at about 7:55. Any help would be greatly appreciated. Getting two errors...
test: #create when logged in with a valid friend_id should assign a friend object. (UserFriendshipsControllerTest):
NoMethodError: undefined method `user_friendships' for nil:NilClass
/../user_friendships_controller.rb:19:in `create'
and
test: #create when logged in with a valid friend_id should assign a user_friendship object. (UserFriendshipsControllerTest):
NoMethodError: undefined method `user_friendships' for nil:NilClass
/../user_friendships_controller.rb:19:in `create'
here is my user_friendships_controller_test.rb
require 'test_helper'
class UserFriendshipsControllerTest < ActionController::TestCase
context "#new" do
context "when not logged in" do
should "redirect to the login page" do
get :new
assert_response :redirect
assert_redirected_to login_path
end
end
context "when logged in" do
setup do
sign_in users(:ian)
end
should "get new and return success" do
get :new
assert_response :success
end
should "should set a flash error if the friend_id params is massing" do
get :new, {}
assert_equal "Friend required", flash[:error]
end
should "display the friend's name" do
get :new, friend_id: users(:mike)
assert_match /#{users(:mike).full_name}/, response.body
end
should "assign a new user friendship" do
get :new, friend_id: users(:mike)
assert assigns(:user_friendship)
end
should "assign a new user friendship to the correct friend" do
get :new, friend_id: users(:mike)
assert_equal users(:mike), assigns(:user_friendship).friend
end
should "assign a new user friendship to the currently logged in user" do
get :new, friend_id: users(:mike)
assert_equal users(:ian), assigns(:user_friendship).user
end
should "returns a 404 status if no friend is found" do
get :new, friend_id: 'invalid'
assert_response :not_found
end
should "ask if you really want to friend the user" do
get :new, friend_id: users(:mike)
assert_match /Do you really want to friend #{users(:mike).full_name}?/, response.body
end
end
end
context "#create" do
context "when not logged in" do
should "redirect to the login page" do
get :new
assert_response :redirect
assert_redirected_to login_path
end
end
context "when logged in" do
setup do
sign_in(:ian)
end
context "with no friend_id" do
setup do
post :create
end
should "set the flash error message" do
assert !flash[:error].empty?
end
should "redirect to the site root" do
assert_redirected_to root_path
end
end
context "with a valid friend_id" do
setup do
post :create, friend_id: users(:mike)
end
should "assign a friend object" do
assert assigns(:friend)
end
should "assign a user_friendship object" do
assert assigns(:user_friendship)
end
end
end
end
end
..and here is my user_friendships_controller.rb
class UserFriendshipsController < ApplicationController
before_filter :authenticate_user!, only: [:new]
def new
if params[:friend_id]
@friend = User.where(profile_name: params[:friend_id]).first
raise ActiveRecord::RecordNotFound if @friend.nil?
@user_friendship = current_user.user_friendships.new(friend: @friend)
else
flash[:error] = "Friend required"
end
rescue ActiveRecord::RecordNotFound
render file: 'public/404', status: :not_found
end
def create
if params[:friend_id]
@friend = User.where(profile_name: params[:friend_id]).first
@user_friendship = current_user.user_friendships.new(friend: @friend)
else
flash[:error] = "Friend required"
redirect_to root_path
end
end
end
4 Answers

Mark Lavelle
2,910 PointsAppreciate the reply Ian It was a typo !! 'twas ever thus! in the controller test in the context of "with a valid friend_id" I had the context closed after the signing in the user setup, meaning the rest of the tests below had no logged in user. Its all working, for now.......

Naomi Freeman
Treehouse Guest TeacherMy initial instinct is to say make this somewhere:
def user_friendship
end
See if that stops it throwing nil:NilClass and throws some other error instead. I'm not very strong on what you need to put inside that def end so that the object can be called by your def create (and I haven't done this module yet) but given my experience with other things, I think you need to define user_friendship first and then call it in the def create.
I may be wrong. But give it a shot.

Mark Lavelle
2,910 PointsIan I am at the same spot, I am using rails 4. but have the exact same error. Have you got anywhere? any solution?
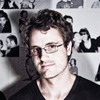
Ian Horner
22,587 PointsHi Mark. Unfortunately not. After spending a great deal of time on it I ended up downloading the project files from a point just after this part in the Rails dev track and continued from there. Let me know if you have any luck.
Esther Olatunde
424 PointsEsther Olatunde
424 PointsThanks Mark, struggled with this for a few hours before I found your comment.