Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial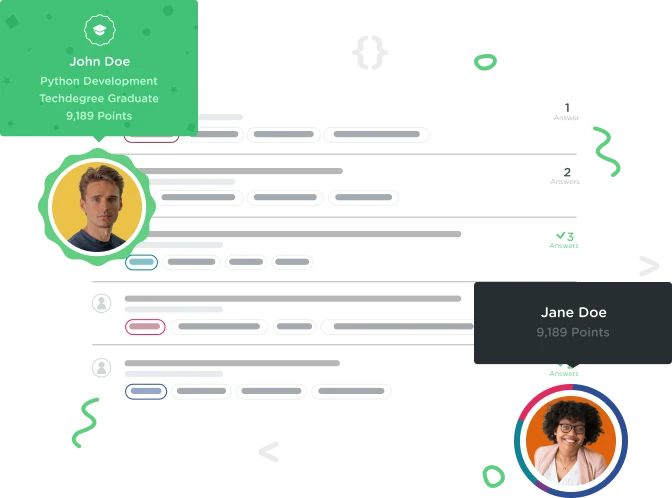
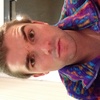
Garan Guillory
Courses Plus Student 19,291 Pointsundefined method 'user_id'
I'm running Rails version 3.2.6 and Ruby version 1.9.3p545.
I installed the latest version of Bootstrap, and not the version found in the videos (maybe that is the problem). Or maybe it is another unseen compatibility issue with "simple_form." I will investigate how to install an older version of Bootstrap, but I am tired of uninstalling and reinstalling.
I would like to finish this track smoothly without error pages every 5 minutes. I have put so much work into this version of the track. I would like to finish this step (Creating a Simple RoR Application) before updating to the newer version.
My code matches the code found in the video.
<%= simple_form_for(@status, html: {class: "form-horizontal"}) do |f| %>
<% if @status.errors.any? %>
<div id="error_explanation">
<h2><%= pluralize(@status.errors.count, "error") %> prohibited this status from being saved:</h2>
<ul>
<% @status.errors.full_messages.each do |msg| %>
<li><%= msg %></li>
<% end %>
</ul>
</div>
<% end %>
<%= f.input :user_id %>
<%= f.input :content %>
<div class="form-actions">
<%= f.button :submit %>
</div>
<% end %>
class Status < ActiveRecord::Base
attr_accessible :content, :user_id
end
16 Answers

Nick Fuller
9,027 PointsDo you have any users in your database?
What you're doing is specifically setting the id of a user record to 1 on your status model, right?
So when you are going to the show page for that status, rails automatically sees the user_id column on the status model and thinks "Oh I need to load the User with an id of 1 for this status!", but what's happening is since that user does not exist in the database, rails ends up sending the object nil
. Then in the view when you call @status.user.first_name
you're calling the method first_name
for the object nil
. That method doesn't existing for nil, which is why you're seeing:
undefined method `first_name' for nil:NilClass
Try navigating to http://localhost:3000/users/sign_up and create a new user. Once you have a user created it will automatically be assigned the id of 1. Then go back to your statuses show page, and it should work!
I hope that makes sense, it's Saturday and I had a long week :P

Nick Fuller
9,027 PointsHi Garan!
Ok, I believe I know what may be going on. It looks like you created an empty migration, then ran it, and then added the code to change the table schema. The issue here is that if you try to run the migration again, rails will not do it because it thinks that it already ran it. So to fix this we need to do what's called a rollback (http://guides.rubyonrails.org/migrations.html#rolling-back).
Basically we are going to "undo" our previous migration. So in console type:
bundle exec rake db:rollback
Once you have successfully rolled back, try running bundle exec rake db:migrate
again to run your actual code.
If this still doesn't solve the problem.. You can completely wipe your database and restart:
bundle exec rake db:drop
bundle exec rake db:create
bundle exec rake db:migrate
Let me know if this doesn't make sense! I hope it helps!

Nick Fuller
9,027 PointsWhen you created the Status model, did you add a user_id column?
A quick way to find out, is to go to terminal and load up your console rails c
and then build a new status object s = Status.new
. Do you see an attribute that reads user_id: nil
?
Another way to check is to go through your database migrations and see if perhaps you misspelled it or possibly forgot it?
This error usually means rails is trying to call .user_id
on your model and it doesn't find anything. Part of the magic of rails is that it maps each database column name as an attribute to your model (check out arel here for some info https://github.com/rails/arel)
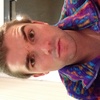
Garan Guillory
Courses Plus Student 19,291 Pointsclass AddUserIdToStatuses < ActiveRecord::Migration
def change
add_column :statuses, :user_id, :integer
add_index :statuses, :user_id
remove_column :statuses, :name
end
end
From the code that I pasted in this reply, I believe that I added the user_id column correctly (db > migrate > add_user_id_to_status).
I followed your instructions ("rails c" and then I built a new status object "s = Status.new), and the error message is the same (the same error message when I attempt to post a new status from the browser). I also tried from the terminal, and I was alerted with this error message:
irb(main):001:0> s = Status.new => #<Status id: nil, name: nil, content: nil, created_at: nil, updated_at: nil>
And no, I did not see "user_id: nil"
I checked in my database migrations, and I did not misspell anything, nor did I forget anything.
I'll read over the rails/arel that is in your reply, but I am not very familiar with Ruby on Rails, so I do not know if the literature will help much.
Thanks for responding. Nick Fuller

Nick Fuller
9,027 PointsTry running in console rake db:migrate
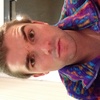
Garan Guillory
Courses Plus Student 19,291 PointsRan it, and the error code is the same.

Nick Fuller
9,027 PointsInteresting, is your code on github? Could you provide me with a link?
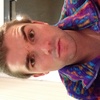
Garan Guillory
Courses Plus Student 19,291 PointsFo sho, I'll add you to my repository. I assume you are the Nick Fuller on github...

Nick Fuller
9,027 Pointsnfuller52
can you give me a link too?
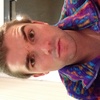
Garan Guillory
Courses Plus Student 19,291 Pointshttps://github.com/garanguillory/treebook
Is this what you need?

Nick Fuller
9,027 Pointsyes! do you have any pending commits that need to pushed? it seems your migration file is empty
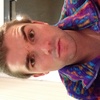
Garan Guillory
Courses Plus Student 19,291 PointsI'll commit all that I have changed recently. One second.
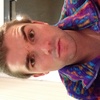
Garan Guillory
Courses Plus Student 19,291 PointsCommitted every thing, let me know if that worked.
Thanks.
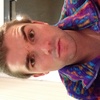
Garan Guillory
Courses Plus Student 19,291 PointsYour solution worked... for a second, and then I ran into more problems. This is what I see when I try to update a status with the user id of "1":
NoMethodError in Statuses#show
Showing /Users/garanguillory/treehouse/projects/treebook/app/views/statuses/show.html.erb where line #5 raised:
undefined method `first_name' for nil:NilClass
Extracted source (around line #5):
2: 3: <p> 4: <b>Name:</b> 5: <%= @status.user.first_name %> 6: </p> 7: 8: <p>
Rails.root: /Users/garanguillory/treehouse/projects/treebook
I have watched the video several times (Creating Relationships), and my code still does not cooperate. Nick Fuller Thanks in advance for the help.
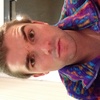
Garan Guillory
Courses Plus Student 19,291 PointsThanks for all the help. I'm cruising now. You are a genius. Nick Fuller

Nick Fuller
9,027 Pointsgood man keep it up! it's all the fun of learnin