Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial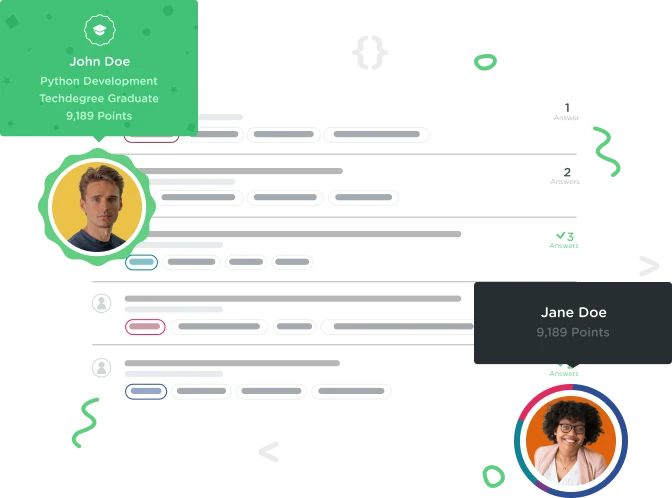

Samuel Harrison
3,484 PointsUndefined Variable match error
Ok so I am splitting my code up for best Practice: Notice: Undefined variable: match in /Users/thebegerks/Desktop/DESIGN WORK/Clients/Web Devlopment/wwwroot/PHP/DJRequests/admin/check.php on line 19
Model:
<?php
function match_users() {
require("../connect.php");
try {
$results = $db->prepare("SELECT * FROM users WHERE username = :username and password =:password LIMIT 1");
$results->bindParam(':username', $username);
$results->bindParam(':password', $password);
$results->execute();
} catch(Exception $e) {
echo "Could not recieve Data from Database";
exit;
}
$match = $results->fetch(PDO::FETCH_ASSOC);
return $match;
}
?>
Controller
<?php
session_start();
require("admin-model.php");
//Set Session info
$_SESSION['username'] = isset($_SESSION['username']) ? $_SESSION['username'] : false;
$_SESSION['id'] = isset($_SESSION['id']) ? $_SESSION['id'] : false;
$_SESSION['nickname'] = isset($_SESSION['nickname']) ? $_SESSION['nickname'] : false;
$_SESSION['rank'] = isset($_SESSION['rank']) ? $_SESSION['rank'] : false;
if( !$_SESSION['username'] or !$_SESSION['id'] or !$_SESSION['nickname'] or !$_SESSION['rank'])
$username= ($_POST['username']);
$password= (md5($_POST['password']));
// create a query string
match_users();
echo $match;
// a salt value, not apparently attached to anything!
/* $salt=uniqid(mt_rand());
// Check connection
if(!$match){
// User Was Not Found
echo "Nothing Found";
} else {
// we found a user in the database, set some session values for future reference
if( $match['active'] == 1){
$_SESSION['username'] = $match['username'];
$_SESSION['id'] = $match['id'];
$_SESSION['nickname'] = $match['nickname'];
$_SESSION['rank'] = $match['rank'];
// now goto whatever page is next
header("Location: ./index.php"); // set to whatever page needs to goto on login success
}else{
echo "Your account is suspended";
echo $match['username'];
}
} */
?>
3 Answers
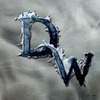
Hugo Paz
15,622 PointsHi Samuel,
You are not assigning the return value to any variable and you need to pass the values to the match_users function so it can bind the values to the query.
//model
function match_users($username, $password)
//controller
$username = ($_POST['username']);
$password = (md5($_POST['password']));
$match = match_users($username, $password);
echo $match;
}

Samuel Harrison
3,484 PointsNotice: Undefined variable: username in /Users/thebegerks/Desktop/DESIGN WORK/Clients/Web Devlopment/wwwroot/PHP/DJRequests/admin/check.php on line 19 bool(false)
MODEL
<?php
function match_users($username, $password) {
require("../connect.php");
try {
$results = $db->prepare("SELECT * FROM users WHERE username = :username and password = :password");
$results->bindParam(':username', $username);
$results->bindParam(':password', $password);
$results->execute();
} catch(Exception $e) {
echo "Could not recieve Data from Database";
exit;
}
$match = $results->fetch(PDO::FETCH_ASSOC);
return $match;
}
?>
Controller
<?php
session_start();
require("./admin-model.php");
//Set Session info
$_SESSION['username'] = isset($_SESSION['username']) ? $_SESSION['username'] : false;
$_SESSION['id'] = isset($_SESSION['id']) ? $_SESSION['id'] : false;
$_SESSION['nickname'] = isset($_SESSION['nickname']) ? $_SESSION['nickname'] : false;
$_SESSION['rank'] = isset($_SESSION['rank']) ? $_SESSION['rank'] : false;
if( !$_SESSION['username'] or !$_SESSION['id'] or !$_SESSION['nickname'] or !$_SESSION['rank'])
$username= (trim(htmlspecialchars($_POST['username'])));
$password= (trim(htmlspecialchars(md5($_POST['password']))));
// create a query string
$match = match_users($username, $password);
var_dump($match);
// a salt value, not apparently attached to anything!
/* $salt=uniqid(mt_rand());
// Check connection
if(!$match){
// User Was Not Found
echo "Nothing Found";
} else {
// we found a user in the database, set some session values for future reference
if( $match['active'] == 1){
$_SESSION['username'] = $match['username'];
$_SESSION['id'] = $match['id'];
$_SESSION['nickname'] = $match['nickname'];
$_SESSION['rank'] = $match['rank'];
// now goto whatever page is next
header("Location: ./index.php"); // set to whatever page needs to goto on login success
}else{
echo "Your account is suspended";
echo $match['username'];
}
} */
?>

Samuel Harrison
3,484 PointsI have fixed it the require file had to be in my if statement