Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial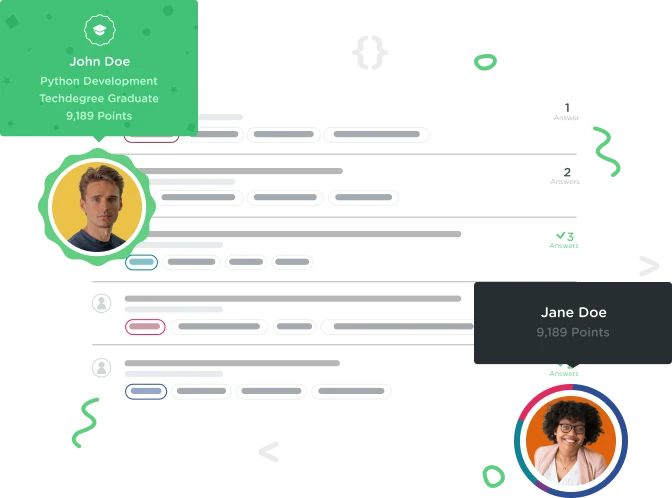

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 PointsUndefinedError: 'forms.RegisterForm object' has no attribute 'hidden'
i tried as others had noted in removing the () from the hidden tag to no avail. any other thoughts?
6 Answers

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 Pointsapp.py
from flask import (Flask, g, render_template, flash, redirect, url_for)
from flask_login import LoginManager
import forms
import models
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = 'lasdkfjalsj234kjlkjvi234'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(userid):
try:
return models.User.get(models.User.id== userid)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
"""Connect to the database before each request"""
g.db = models.DATABASE
g.db.connect()
@app.after_request
def after_request(response):
"""Close the database connection after each request"""
g.db.close()
return response
@app.route('/register', methods=('GET', 'POST'))
def register():
form = forms.RegisterForm()
if form.validate_on_submit():
flash("Yay you registered!", "success")
models.User.create_user(
username=form.username.data,
email=form.email.data,
password=form.password.data
)
return redirect(url_for('index'))
return render_template('register.html', form=form)
@app.route('/')
def index():
return 'Hey'
if __name__ == '__main__':
models.initialize()
try:
models.User.create_user(
username='cstuart',
email='crstuart@gmail.com',
password='password',
admin = True
)
except ValueError:
pass
app.run(debug=DEBUG, host=HOST, port=PORT)

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 Pointsnote i'm also getting a 'Connection Already Open' Error and i did find another question/solution and did add that code--but it's not helping.

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 Pointsforms.py
from flask_wtf import Form
from wtforms import StringField, PasswordField
from wtforms.validators import (DataRequired, Regexp, ValidationError, Email,
Length, EqualTo)
from models import User
def name_exists(form,field):
if User.select().where(User.username == field.data).exists():
raise ValidationError('User with that name allready exists')
def email_exists(form,field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('Email with that name allready exists')
class RegisterForm(Form):
username = StringField(
'Username',
validators=[
DataRequired(),
Regexp(
r'^[a-zA-Z0-9_]+$',
message=("Username should be one word, letters"
"numbers, and underscores only.")
),
name_exists
])
email = StringField(
'Email',
validators=[
DataRequired(),
Email(),
email_exists
])
password = PasswordField(
'Password',
validators=[
DataRequired(),
Length(min=5),
EqualTo('password2', message='Passwords must match')
]
)
password2 = PasswordField(
'Confirm Password',
validators = [DataRequired()]
)

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 Pointsmodels.py
import datetime
from flask_bcrypt import generate_password_hash
from flask_login import UserMixin
from peewee import *
DATABASE = SqliteDatabase('social.db')
class User(UserMixin, Model):
username = CharField(unique=True)
email = CharField(unique=True)
password = CharField(max_length=100)
joined_at = DateTimeField(default=datetime.datetime.now)
is_admin = BooleanField(default=False)
class Meta:
database = DATABASE
order_by = ('-joined_at',)
@classmethod
def create_user(cls, username, email, password, admin=False):
try:
with DATABASE.transaction():
cls.create(
username=username,
email=email,
password=generate_password_hash(password),
is_admin=admin)
except IntegrityError:
raise ValueError("User already exists")
def initialize():
DATABASE.connect()
DATABASE.create_tables([User], safe=True)
DATABASE.close()

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 Pointssorry i didn't post the register code--my bad ....it turns out however that there was a typo in the hidden tag
instead of form.hidden_tag()
i had form.hidden.tag()
how do you change the color of the code? Thanks Chris
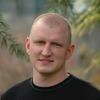
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsSo for the syntax highlighting immediate after the first 3 backticks ( on the same line no spaces) you put the language you want the syntax to be. Checkout the Markdown Cheatsheet example Code Block. It is highlighting with html.

Christopher Stuart
Full Stack JavaScript Techdegree Graduate 27,771 Pointsah...ok thank you chris
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsChris Howell
Python Web Development Techdegree Graduate 49,702 PointsHey Christopher Stuart
Where are you trying to call hidden at? I didnt see you calling it anywhere in the code above? So somewhere in your template tags? If so, could you add the template code for those template files :)
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsChris Howell
Python Web Development Techdegree Graduate 49,702 PointsEDIT I changed your code snippets to syntax highlight with Python. So it wasnt all in 1 color. :)