Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial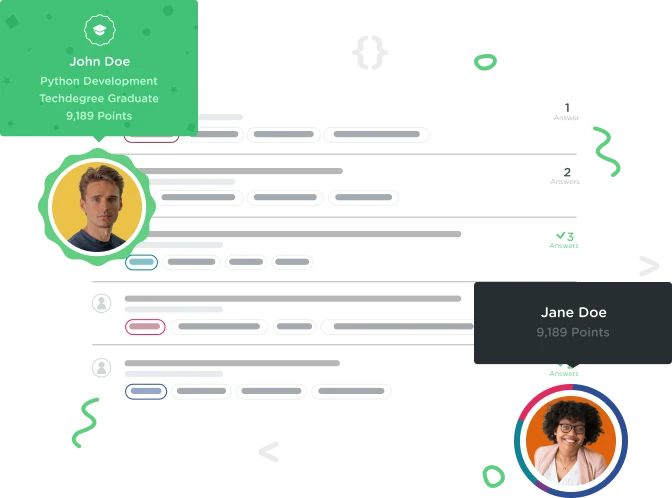
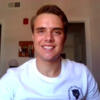
Evan Welch
1,815 PointsUnderstanding C# Interfaces & Fields and Properties
Say I have an interface
interface IPets
{
int HungerGage;
int HungerGageEquivelentAsProperty{ get; }
}
Using the same namespace, why can I not instantiate these properties and fields like this:
class Cat : IPets
{
int IPets.HungerGage = 100;
int IPets.HungerGageEquivelentAsProperty = 100;
}
Or instantiate these like this:
class Cat : IPets
{
public int HungerGage = 100;
public int HungerGageEquivelentAsProperty = 100;
}
I've been getting errors so much with these that obviously I don't understand. I've used the keyword override
plenty as well, and it doesn't seem to work consistently for me either. I've seen the int IInterface.field = variable
in the microsoft documentation, but it seems that my C# instructor here was able to flawlessly use public int field = variable
. For me, neither work, so help would be appreciated! Thanks
1 Answer

Simon Coates
8,223 PointsYou might care to provide links to the treehouse video and to the microsoft documentation (and maybe code snippets). I'm not really a c# expert, but I think microsoft says that you can't provide fields on an interface ("An interface may not declare instance data such as fields, auto-implemented properties, or property-like events."). It sounds like there might be an ability to use static fields but it sounds like it might be newish. I tried running a quick demo in workspace and whatever version of c# they use (I'd assume probably a little older), it won't let me define fields and I have to provide implementation for properties to get it to compile. For reference, my demo code was:
using System;
class MainClass {
public static void Main (string[] args) {
IPet fido = new Dog();
}
}
interface IPet
{
int HungerGageEquivelentAsProperty{ get; }
int SomethingElse { get; set; }
}
class Dog : IPet {
//won't compile if i don't provide implementation.
public int HungerGageEquivelentAsProperty{ get; } = 200;
public int SomethingElse { get; set; }
public Dog()
{
SomethingElse = 100;
}
}
it seems to at least let me use properties with an implicit syntax. If that's all you need, then maybe that gets you by for now.