Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial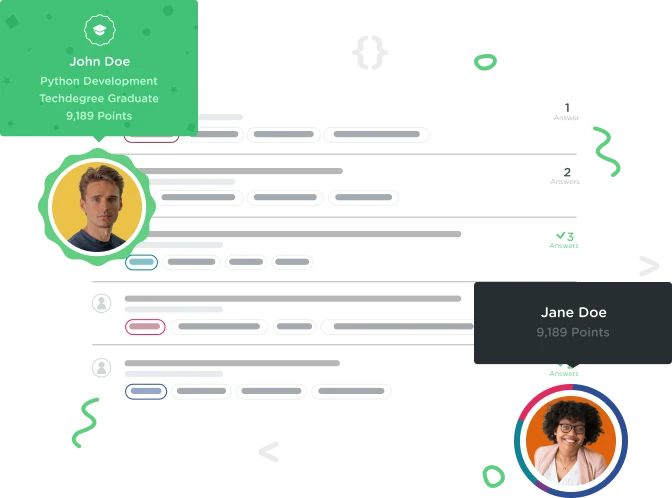

Alex Flores
7,864 PointsUnderstanding DOM, getElementById, .ready?
Hey everyone,
Somewhat new to coding and I'm just now starting to get into writing code a lot. As I'm writing it though, I'm trying to figure out how to write quality code that is best optimized for modern browsers. However, I'm having a tough time trying to conceptualize this. I have found that there are a number of ways to perform the same action, but others are considered better than others. For instance, both codes below do the exact same thing:
$("#info-click").click(function() {
$("#pages, #information").removeClass("hide");
$("#portfolio, #contact").addClass("hide");
});
$("#port-click").click(function() {
$("#pages, #portfolio").removeClass("hide");
$("#information, #contact").addClass("hide");
});
$("#contact-click").click(function() {
$("#pages, #contact").removeClass("hide");
$("#information, #portfolio").addClass("hide");
});
and
$(document).ready(function() {
$("#info-click").click(function() {
$("#pages, #information").removeClass("hide");
$("#portfolio, #contact").addClass("hide");
});
$("#port-click").click(function() {
$("#pages, #portfolio").removeClass("hide");
$("#information, #contact").addClass("hide");
});
$("#contact-click").click(function() {
$("#pages, #contact").removeClass("hide");
$("#information, #portfolio").addClass("hide");
});
});
However, I would think most people would say that latter one is better because I use $(document).ready(function() - I'm not sure why that is though. Also, I've been reading that I should use getElementById() when traversing the DOM, but is this always the case? should I use it here? Does anyone know what I should look up to get a better understanding on writing optimized code?
Thanks!
3 Answers
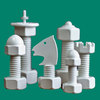
Steven Parker
231,275 PointsAs Jennifer pointed out, the latter version guarantees that the handlers are installed after the document is loaded and ready. But it wouldn't make much difference if you were careful to include the first version at the very bottom of the HTML.
And getElementById() is one of a set of methods that are all equally valuable for traversing the DOM in JavaScript. But when you use a JQuery selector like $("#contact-click"), it is calling these methods for you, but providing a more compact (and more readable) form for using them.
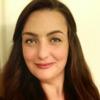
Jennifer Nordell
Treehouse TeacherThe reason we would prefer to have the latter example with the document ready part is because it ensures that we don't start trying to run our code before the document is ready. For example if it's trying to run that code but for some reason the element with the #info-click ID hasn't loaded into the DOM yet, this could produce errors or just not work at all.

Alex Flores
7,864 PointsThanks everyone!