Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial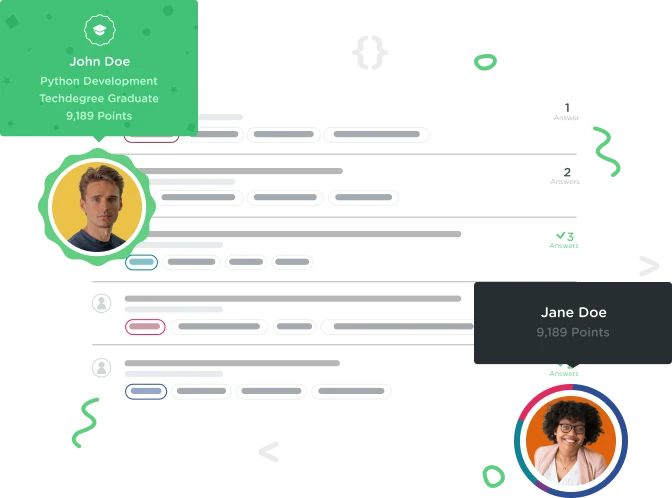
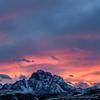
Jason Dsouza
1,378 PointsUnderstanding JS Callbacks
I really dont understand how to use JS Callbacks. I'm starting out with basic Js and not jQuery and so on. For example: Andrew used "callback" as an argument. When defining callbacks, is it necessary to add "callback" as an argument?
Ive gone through several documentations including MDN and non-MDN sources, but still havent been able to grasp the topic. Is there a simpler way of teaching callbacks.
PS- Andrew is a really good teacher, but I sometimes find it hard to understand what he says despite turning CLOSED CAPTIONS on!
3 Answers
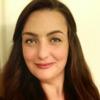
Jennifer Nordell
Treehouse TeacherHi there! This might take some getting used to, but just like numbers, arrays, strings can be passed around and assigned to variables in JavaScript, so can functions! You can pass a function from one to another.
In Andrew's example, he chose to name the parameter that's going to hold the function we're sending in a local variable named callback
. But let's see if we can come up with a different name and see if it makes more sense.
This is actually a slightly more advanced example, but I feel like it might drive home the point of how we can pass around functions just like any other kind of data. I've included commenting
function sayHello(name) {
// The string we send in is assigned to the variable name
console.log('Hello ' + name);
}
function sayHiBack(name) {
// The string we send in is assigned to the variable name
console.log('Hi there, ' + name + '! It is nice to meet you.');
}
function executeCallback(functionToExecute, personName) {
// functionToExecute is now assigned a reference to the sayHello function
// The next line is equivalent to sayHello(personName);
functionToExecute(personName);
}
// Call the executeCallback and send it the function sayHello and send in your name
executeCallback(sayHello, "Jason");
// call the executeCallback and send it the function sayHiBack and send in my name
executeCallback(sayHiBack, "Jennifer");
Hope this helps!

Thomas Gauperaa
3,584 PointsHi Jason, a callback function is a function that is called right after the main function has finished executing, a sort of latched on function. No, you don't have to write "callback" for it to be handled like a callback. Callback functions are really useful if a function can't/shouldn´t be run before the previous one has finished, or if you want to tidy up, close connections after something has finished running.
I've been learning React lately which has this function called "setState" to update the state in the app and update the components and page. The thing about setState is that you can´t rely on several setState calls being called in the order they were written. So if the second setState is depending on the first, then a callback is useful to be sure it doesn't run before the other one has finished.
I´ve also seen callback function being mentioned in a slightly different setting, like for instance ".map" which can be run on arrays. With ".map" you write a callback function that's run for every slot in the array. Let's say you had an array of names and you wanted a copy of the array where all the names started with a capital letter, you then do:
const names = ["joe", "rachel", "ross"];
let namesCapital = [];
namesCapital = names.map (function (name, index) { /*this anonymous function is the callback function*/
return name.charAt(0).toUpperCase() + name.slice(1);
});
console.log(namesCapital);
Hope this helps.
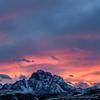
Jason Dsouza
1,378 PointsI seem to have grasped a hold on callbacks. I think with practice, this will become much easier for me.
Thanks a lot, Jennifer!!
Jan Xavier Virgen
Full Stack JavaScript Techdegree Graduate 19,751 PointsJan Xavier Virgen
Full Stack JavaScript Techdegree Graduate 19,751 PointsThis is really cool!