Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial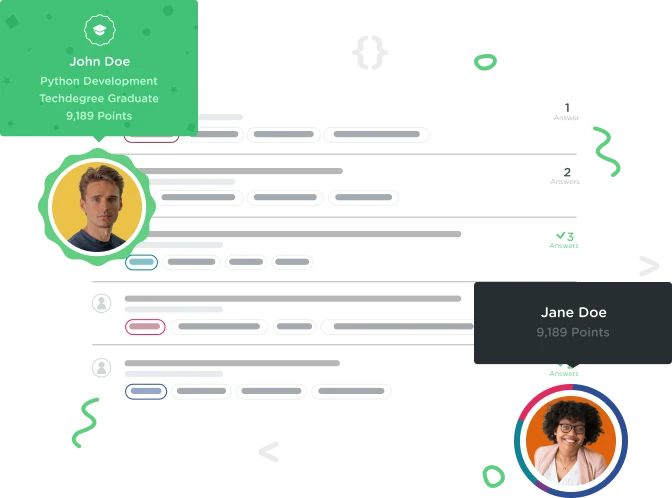

Paden Clayton
3,101 PointsUnderstanding Method Structure
Ok forgive me if this seems self explanatory but I've been using C style languages for most of my life and I'm having a hard time with the transition to the Obj-C logic here.
In PHP/Javascript/etc we do this:
function functionName( $var1, $var2 ) {
// Add the 2 vars
}
$varName = functionName( 3, 4 ); // Results in 7
In C we do this (forgive me if this is off, my C is about 13 years rusty):
int functionName( int var1, int var2 ) {
// Add the 2 vars
}
int var1 = 3;
int var2 = 4;
int varName = functionName( var1, var2 ); // Results in 7
According to the video we are doing this for Obj-C:
-(int)functionNameVar1:(int)var1 var2Something:(int)var2 { // Yes functionName is a method now
// Add the 2 vars
}
int var1 = 3;
int var2 = 4;
int varName = [class functionNameVar1:var1 var2Something:var2 ]; // Results in 7
Ok my question here is understanding, what seems to be, the butchered code in Obj-C.
- Why in Obj-C are combining a var into the name of the method?
- Why is there what almost seems to be an unnecessary repetition for the var2Something:(int)var2? ( In the example this would actually look like var2(int)var2 )
- Is the var2Something prefix necessary because now we no longer use the parenthesis so the compiler has to know that its a variable of the method being called?
I hope someone can help me wrap my head around this, it just seems overly complicated for such a simple portion of coding.
1 Answer

Ron Casey
1,419 PointsI'm new to Objective-C, but here's my take thus far.
1) Objective-C method declaration is all about self-documentation. The idea is a person can get a solid grasp on what your method does by simply reading the method name.
This makes sense if you take a look at some methods provided in the SDKs:
- (void)setTitleColor:(UIColor *)color
forState:(UIControlState)state;
When calling this method (which is an instance method on UIButton), I can infer I am setting the color of the button for a specific state. I can see which objects I need to pass to UIButton to accomplish that. So when I call the method:
[button setTitleColor:redColor forState:Highlighted];
Someone reading my code could easily see I just set the color of my button to red, when the button is in the Highlighted state.
Let's take a look at what this would look like in C:
void setTitleColorForState (UIColor* color, UIControlState state);
button.setTitleColorForState(redColor, Highlighted);
Imagine a method that took in lots of objects, like NSBitmapImageRep init:
- (id)initWithBitmapDataPlanes:(unsigned char **)planes
pixelsWide:(NSInteger)width
pixelsHigh:(NSInteger)height
bitsPerSample:(NSInteger)bps
samplesPerPixel:(NSInteger)spp
hasAlpha:(BOOL)alpha
isPlanar:(BOOL)isPlanar
colorSpaceName:(NSString *)colorSpaceName
bitmapFormat:(NSBitmapFormat)bitmapFormat
bytesPerRow:(NSInteger)rowBytes
bitsPerPixel:(NSInteger)pixelBits;
Imagine that in C:
image.initWithBitmapDataPlanes (w, h, sample, spp, TRUE, FALSE, colorSpace, bitmapFormat, x, y);
2) Xcode has amazing auto code completion. The vast majority of the time you'll only need to type out your method declaration once, and every time you call it you'll just be entering in object names while hitting tab.
3) Yes. See 1 & 2 for why.
Paden Clayton
3,101 PointsPaden Clayton
3,101 PointsThanks, this cleared it up, I think the major difference in the thought process is that in c and other laguages we try to make our variables speak for themselves and that servers as the only doumentation for example:
image.initWithBitmapDataPlanes (pixelsWide, pixelsHigh, bitsPerSample, samplesPerPixel, hasAlpha, etc)
Those variables server as both the indicator of what the input should be and are the same name we use internally in the function. Whereas in Obj-C the makeup is more like this:
publicNameForDocumentationPurposes:(type)internalNameUsedInMethod
This definitely clears things up so thanks again!