Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial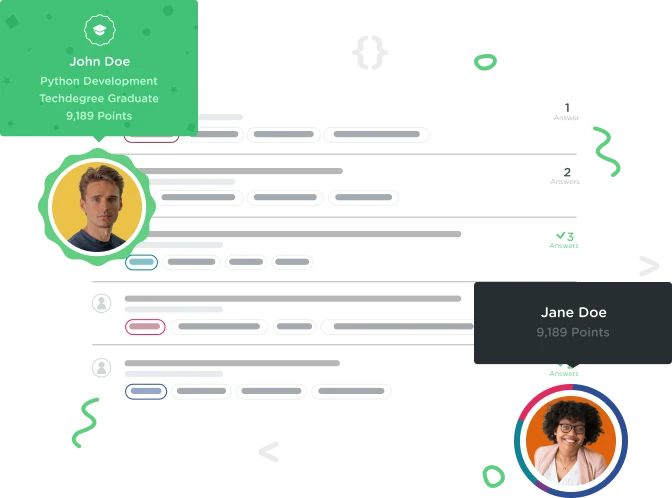

Simon Tanner
5,776 PointsUnderstanding parameters
Hi, please could you help me understand how the list
parameter in function printList( list )
knows how to access (sorry can't think of the right term?) the elements in the array var playlist
. I'm a bit lost and thought it would have needed to be function printList (playList)
for it to work? Thanks for any help!
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
function print(message) {
document.write(message);
}
function printList( list ) {
var listHTML = '<ol>';
for ( var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList);
4 Answers
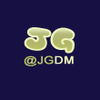
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Simon,
In the function, list
is simply a variable that's been passed into the function as a parameter. It's simply a placeholder for a value for which an action will be taken.
In the example, list is a placeholder for the array. Rather than reference the array directly, it's actually passed into the function call as an argument.
so list
is in actuality the playList array. :-)
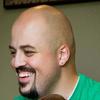
Vinnie Dauer
12,824 PointsThe function doesn't automatically know to access playList. It accesses playList when you call the function with playList as the "list" parameter. A function is written like this so that you can call any array parameter when the function is called. I hope that clears it up.

Simon Tanner
5,776 PointsThanks a lot Jonathan and Vinnie - both really helpful!
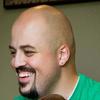
Vinnie Dauer
12,824 PointsNo problemo!

Fabio Silva
5,097 PointsI still don't get it! What if there were more arrays? How the function would know which array to access?
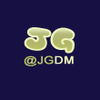
Jonathan Grieve
Treehouse Moderator 91,253 PointsIf you wanted to include more arrays you'd declare the array outside the function and then pass it in as another parameter,
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
var playListTwo = [
'new',
'songs',
'would',
'go',
'here'
];
function print(message) {
document.write(message);
}
function printList( list, list2 ) {
var listHTML = '<ol>';
for ( var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>';
}
for(var i=0; i <list2.length; i +=1) {
listHTML += '<li>' + list2[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList, playList2);
There comes a point where you'd have to find anther way to do this if you have too many parameters but that's another story. :)