Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial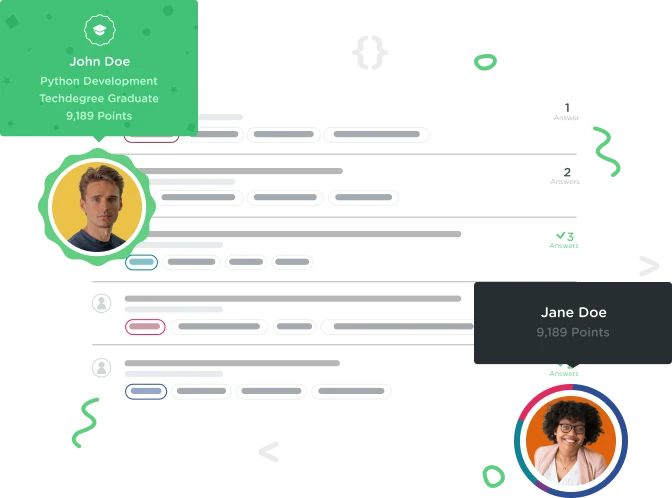
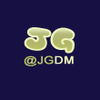
Jonathan Grieve
Treehouse Moderator 91,253 PointsUnderstanding ReactJS
Hi there. :)
I've got some React code and it works but I'm at stage 4 of the course and getting to the point I'm starting to struggle to keep up with what's being taught.
So I've added some comments to what I've done so far, Section 4, video 2, to see if I'm understanding what's happening so far.
Thanks :)
/*object literal holding player and score details*/
var PLAYERS = [
{
name: "Jack Bauer",
score: 31,
id: 1,
},
{
name: "Tony Almeida",
score: 6,
id: 2,
},
{
name: "Renee Walker",
score: 7,
id: 3,
},
];
/*Header of app - displaying scoreboard to screen*/
function Header(props) {
return (
<div className="header">
<h1>{props.title}</h1>
</div>
);
}
/*Declare a propType that is a required string*/
Header.propTypes = {
title: React.PropTypes.string.isRequired,
};
function Player(props) {
return (
<div className="player">
<div className="player-name">
{props.name}
</div>
<div className="player-score">
<Counter score ={props.score}/>
</div>
</div>
);
}
/*Make 2 properties of player component. a string and number, both required properties*/
Player.propTypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
};
/*Increment and decrement scores on button click*/
function Counter(props) {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<div className="counter-score"> {props.score} </div>
<button className="counter-action increment"> + </button>
</div>
);
}
/*Counter component a required number property*/
Counter.propTypes = {
score: React.PropTypes.number.isRequired
}
/* Application state */
var Application = React.createClass({
propTypes: {
title: React.PropTypes.string,
initialPlayers: React.PropTypes.arrayOf(React.PropTypes.shape({
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
id: React.PropTypes.number.isRequired,
})).isRequired,
},
/*component that renders default title to screen*/
getDefaultProps: function() {
return {
title: "Scoreboard",
}
},
/*Grabs initial list of players from object literal*/
getInitialState: function() {
return {
players: this.props.initialPlayers,
};
},
render: function() {
return (
<div className="scoreboard">
<Header title= {this.props.title} />
<div className="players">
{
this.state.players.map(function(player) {
return <Player name={player.name} score={player.score} key={player.id} />
})
}
</div>
</div>
);
}
})
/*Render react app to the browser*/
ReactDOM.render(<Application initialPlayers={PLAYERS} />, document.getElementById('container'));
Laurie Williams
10,174 PointsLaurie Williams
10,174 PointsI think the problem with the course is that it doesn't show home components are compartmentalized.
It wasn't until I put my components into separate files that I understood how state is passed from the parent to the child components.
I recommend separating your components into individual files.
Here's my github if anyone is interested seeing how I separated my components:
https://github.com/LaurieWilliamsNZ/React-Scoreboard.git