Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial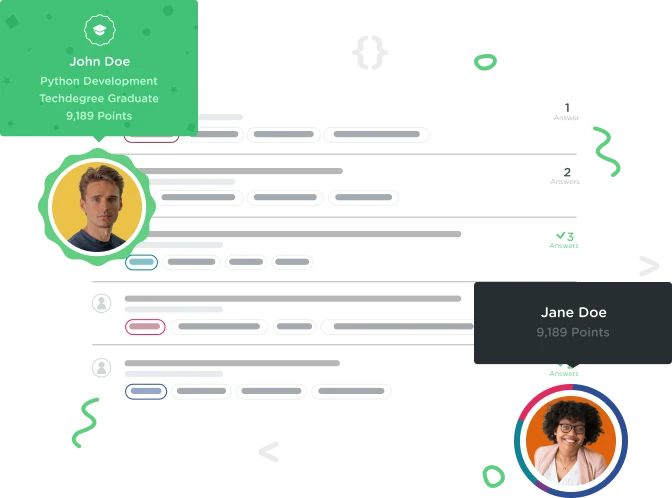
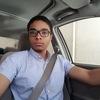
Luqman Shah
3,016 PointsUnderstanding returns - please review my code
function buyPhone(phone, currency, price) {
if (price === 400) {
alert("You've reached your budget");
} else if (price < 400) {
alert("You've stayed within budget")
} else {
alert("You are not within your maximum budget")
}
}
buyPhone('iPhone X', '$', 1000);
function buyPhone(phone, currency, price) {
if (price === 400) {
return "You've reached your budget"
} else if (price < 400) {
return "You've stayed within your budget"
} else {
return "You are not within the max budget"
}
}
alert( buyPhone('iPhone X', '$', 1000) );
console.js( buyPhone('iPhone X', '$', 1000) );
So I've made these two examples, to make myself better understand return values in js functions. So from the first code when we call the buyPhone function, we are limited to only alerts. So essentially, from the second example, the return value basically gives us more flexibility with how we want the programming within the code block to display(or return)?
The return value is just giving us multiple ways of executing the programming, whether it be in an alert dialog, the web page itself, stored in a variable?
If we wanted our code to be executed more flexibly with the first example, we'd have to write multiple lines of code within the code block, each with it's own object? Such as:
function buyPhone(phone, currency, price) {
if (price === 400) {
alert("You've reached your budget");
console.js("You've reached your budget");
} else if (price < 400) {
alert("You've stayed within budget");
console.js("You've stayed within budget")
} else {
alert("You are not within your maximum budget");
console.js("You are not within your maximum budget");
}
}
buyPhone('iPhone X', '$', 1000);
Am I understanding this correctly?
1 Answer
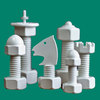
Steven Parker
231,599 PointsI think you have the basic idea, but the third example would not be good programming practice. If you needed to output the result mutliple ways, you'd probably construct a function with a return like in your second example. But instead of calling it twice it would be better to store the result of calling it once in a variable, and then use that in various ways:
var budgetMessage = buyPhone('iPhone X', '$', 1000);
alert( budgetMessage );
console.log( budgetMessage );
I'm also assuming you meant "console.log" instead of "console.js".
Luqman Shah
3,016 PointsLuqman Shah
3,016 PointsOhh ok that makes sense, thank you!