Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial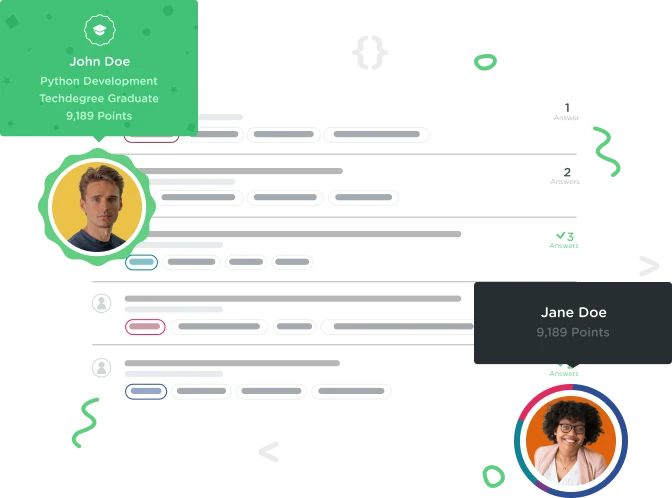

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsUnderstanding searching records
BELOW Is the coded i have created to generate a student record search. I still need to understand why it wont work. Also, if some clarification on how the function is performing the search can be explained that would be helpful.
''' var message = ''; var student; var search;
function print(message) { var outputDiv = document.getElementById('output'); outputDiv.innerHTML = message; }
function getStudentReport(student){ var report = '<h2>Student: ' + student.name + '</h2>'; report += '<p>Track: ' + student.track + '</p>'; report += '<p>Points: ' + student.points + '</p>'; report += '<p>Achievements: ' + student.achievements + '</p>'; return report; }
while (true){ search = prompt ('Search student records: type a name [Jody] (or type "quit" to end)'); if (search === null || search.toLowerCase() === 'quit'){ break; } }
for (var i = 0; i < students.length; i += 1) { student = students[i]; if (student.name === search) { message = getStudentReport( student ); print(message); } } } }'''
2 Answers
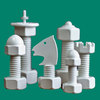
Steven Parker
231,269 PointsThe "for" loop that searches the student array is currently outside of the main "while" loop, so it does not get performed until "quit" is entered (and you probably don't have any student named "quit"!).
Also, there appear to be two extra closing braces at the end of the program that don't have any matching open braces.

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsIn regards to another project. My first project of the techdegree. I hope you could look over what i have so far for the Random quote generator and tell me if I'm even close to being finished. I would really appreciate it.
// event listener to respond to "Show another quote" button clicks
// when user clicks anywhere on the button, the "printQuote" function is called
document.getElementById('loadQuote').addEventListener("click", printQuote, false);
var quotes;
var randomInt;
var quoteObject;
var html;
//Object literal quotations
var quotes = {
quote: "Do not pray for an easy life, pray for the strength to endure a difficult one.",
source: "Bruce Lee",
quote: "The only way to do great work is to love what you do. If you haven't found it yet, keep looking. Don't settle.",
source: "Steve Jobs",
quote: "Talk to yourself once a day, otherwise you may miss meeting an excellent person in this world.",
source: "Swami Vivekananda",
quote: "You can do anything but not everything",
source: "Making it all work by David Allen 2009",
quote: "I think it is possible for ordinary people to choose to be extraordinary.",
source: "Elon Musk"
};
//Random number function
function getRandomQuote() {
var randomInt = Math.floor(Math.random * quotes.length);
return quotes[randomInt];
}
// Function that calls on randomQuote then prints to HTML
function printQuote() {
var html = '<p class = "quote">' + getRandomQuote.quote + '</p>' +
'<p class = "source">' + getRandomQuote.quote + '</p>';
var quoteObject = document.getElementId('quote-box').innerHTML;
print(quotes);
}
//Random changing background color
function randomBackground () {
var red = Math.floor( Math.random() * 255 );
var green = Math.floor( Math.random() * 255 );
var blue = Math.floor( Math.random() * 255);
var rgbColour = 'rgb(' + red + ',' + green + ',' + blue +')';
document.body.style.backgroundColor = rgbColour;
}

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsThanks
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThose 3 marks to start and end a code section should be accents ("backticks"), not apostrophes.
And add "js" to the first set to get syntax coloring.