Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial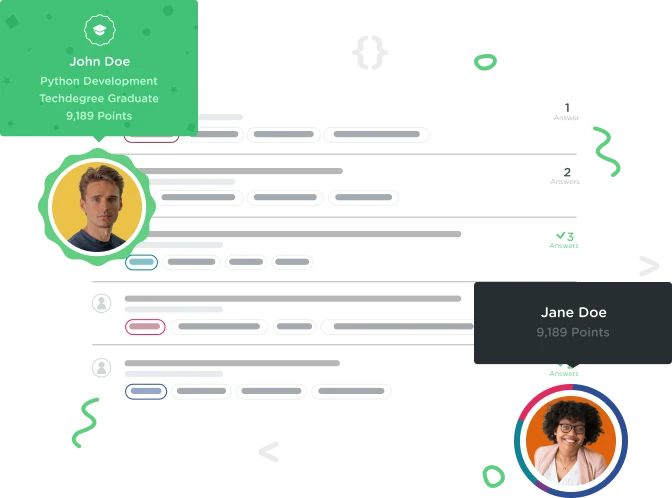

Deborah Williamson
2,997 PointsUnderstanding the challege
I feel like I'm the only one who didn't really grasp what we're so suppose to do. Even watching this video didn't clear up things for me. No matter how many times I watch it. It's really overwhelming and I don't want to move on without fully getting it. Help?
3 Answers
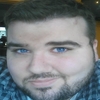
Marcus Parsons
15,719 PointsHey Deborah,
I'm not quite sure what all you do or don't know, so please excuse me in advance if I am a tad thorough in my explanation.
In the first challenge, your goal is to generate a number between 0 and some specified integer. So, the base part of the code is to take Math.random() (which generates a number from 0-0.99999...) and multiply it by the specified integer. But, since you can see those decimal points that are generated from Math.random(), we want to use Math.floor() to shave off any decimal points on the integer and round it down to the nearest integer i.e. 5.1 or 5.9 both would become 5, 3.3 or 3.8 would be 3, etc. So now we have:
Math.floor(Math.random() * specifiedInteger);
But, we're still not done because we want to include the specified integer and with just that code above, we could only get a maximum number of 1 less than the specified integer. If you want to see the math on that, I'll show you what I mean. So, we add 1 to the statement above. This 1 can be placed either inside the floor() function or outside of it. Either way, you will achieve the desired result.
Math.floor(Math.random() * specifiedInteger) + 1;
//Or:
//Math.floor(Math.random() * specifiedInteger + 1);
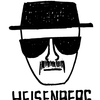
Carlo Sabogal
7,468 PointsMarcus, why did you mention: "If we typed out: Math.floor(Math.random() * randMax + randMin); we would have a range of [0,150) which is not what we want."
if random is 0, then this is 0*100 = 0 + 50 = 50, so the range isn't [50,150]?
thanks
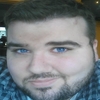
Marcus Parsons
15,719 PointsYou are correct! I mistyped what I wrote. I'll change that.

Deborah Williamson
2,997 PointsYour breakdown was perfect. I just need to wrap my head around the second challenge. Thank you so much for the help.
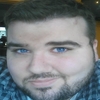
Marcus Parsons
15,719 PointsYou are very welcome, Deborah! That's also why I wrote it in so much detail, so that you can follow along with it later. :) Happy Coding!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThe 2nd challenge is, of course, a bit more complicated. You want the user to specify the range of values for which they wish a number to be randomly taken from. So, let's say we have two variables
randMin
andrandMax
. And to put these into perspective, I'm going to use mathematical interval notation where a [ or ] means an inclusive integer, and ( or ) mean exclusive integers.So, let's start with this code:
Math.floor(Math.random() * randMax);
This code above will generate a number from 0 to randMax. Let's say, for mathematics sake, that we are trying to get a number between 50 (randMin) and 100 (randMax). So, the range right now with those numbers would be [0,100).
But, what we need is from [50, 100]. So, if we think about it, we could just add in
randMin
torandMax
. But, we can't do that within the floor statement because remember, Math.random() can go to 0 and anything times 0 is 0. If we typed out:Math.floor(Math.random() * (randMax + randMin));
we would have a range of [0,150) which is not what we want.But, if we place
randMin
outside of the floor statement, we would get an interesting result:Math.floor(Math.random() * randMax) + randMin;
Now, the range becomes [50,150) because the inside can go all the way to 0 but the outside will add in 50, and the max stays the same. Think of the outside now as the minimum range. So, we're getting closer.
What we now need to do is take away 50 from the top range. Well, we couldn't just subtract randMin from itself on the outside because that would be just adding 0 which gets us back to square one.
But, if we subtract it away from randMax on the inside, the inside can now go to a minimum of 0 and when you add 50 from the outside makes the minimum 50.
Math.floor(Math.random() * (randMax - randMin)) + randMin;
And when you take a maximum of 49 on the inside (which is the floored value 0.9999 * 100 - 50) and add that to the minimum on the outside, we get 99, which means the range is now from [50, 100).
The final step to make that last number inclusive is the exact same final step as the first challenge: just add a 1. It is important where you place the 1 here, because we're dealing with two dynamic numbers instead of just one. The 1 must go inside the floor statement with the randMax and randMin variables.
So, the final solution to getting a random number between two values is:
Math.floor(Math.random() * (randMax - randMin + 1)) + randMin;
If you want to test this out, run your web console (or create a test HTML page) and type this in:
I know this is rather lengthy, but as I said, I tend to try to be as thorough as possible in my explanations. I hope that helps!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThe absolute best way to learn this concept is to try to re-create this in your own way, and play around with all of the code! Trial and Error leads to awesomeness!