Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial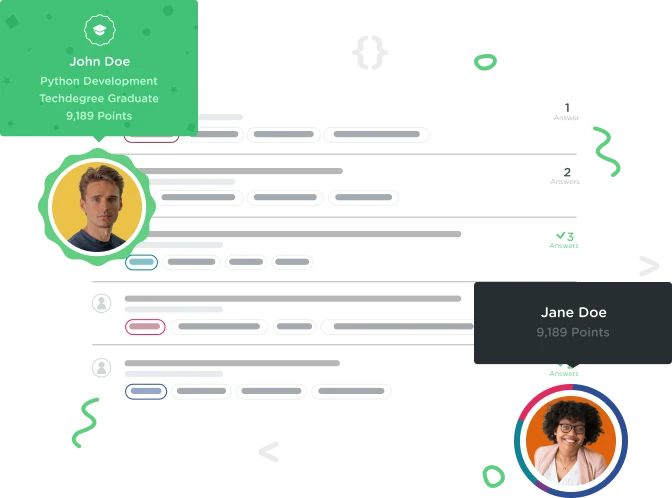

William J. Terrell
17,403 PointsUnderstanding the Code in the "Working with Functions" Quiz
I got most of the quiz correct, but I'm having some trouble understanding the "why" behind some of the answers involving code examples. I have included them below, with my questions regarding each individual one in the form of a comment inside the code itself.
1.)
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
}
echo $sum;
// This one outputs "6" when parsed, but I don't understand why
?>
2.)
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$output = $number . $output;
}
}
echo $output;
// This one outputs "321". I understand why it puts it out in that order, but why doesn't it include "4"?
// Is the function following the logic of "For as long as $i is less than $total, do this"...
//...and so "4" is not "run through" the system because it would be "equal" to $total, rather
//than "less than"?
?>
3.)
<?php
$x = 1;
function sum_two_numbers($x,$y) {
$z = $x + $y;
return $z;
}
$y = sum_two_numbers(2,3);
echo $x;
//This one outputs "1". I am assuming this is because "$x = 1;" is defined outside of the "scope"
//of the function, and so it is not "run through" the function (and thus not returned by it)?
?>
4.)
<?php
function sum_two_numbers($one,$two) {
$sum = $one + $two;
return $sum;
}
sum_two_numbers(1,5);
// Lastly, this one returns nothing. I assume that is just because no "echo" is called,
// but would there also be something to the line that just has "sum_two_numbers(1,5);"?
// Or am I just making it out to be more than it really is? :P
?>
Any insight would be appreciated :) In fact, it would be great if @Randy Hoyt could offer an observation on my understanding of the code thus far :)
Thanks!
1 Answer
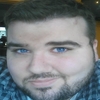
Marcus Parsons
15,719 PointsHi William,
1) In this first example, $i
acts as a counter variable. When the foreach loop executes, each number in the array $numbers becomes the variable $number. The first statement adds 1 to the current value of the $i variable. So, $i immediately becomes 1. Then, you come to the if statement that questions whether $i is less than $total or not. $total is 4 because there are 4 elements in the $numbers array. So long as $i is less than $total, then $sum will be equal to its previous value plus the current number the loop is on.
So here's a run through of that: $i becomes 1 when the loop starts and so the if statement executes, and $sum is equal to itself plus the current number value the loop is on, 0+1= 1. The next loop $i becomes 2. The if statement executes and $sum is equal to the same thing I said, $sum was 1 so its equal to itself plus the next number value: 1+2 = 3 (remember that $sum was 1 and the next number in the array is 2). And the loop continues on, $i becomes 3 and is still less than $total, so $sum = 3 + 3 (sum was 3 and the next number in the array is 3). Now, $sum = 6. The loop goes through again because it is on the last index of the array, $i becomes 4, but the if-statement does not execute because $i is not < $total (4 is not less than 4).
2) A very similar thing happens except that the number values are concatenated with a string. So, each number value that the loop goes through just gets pushed into a string. It only takes the first 3 numbers for the exact same reason as above. So, 1 is put in first, then 2, and then 3 which makes a string of "321".
3) You're exactly right. $x exists outside the scope of the function, and since it wasn't imported into the function, its value remains unchanged despite there being an $x placeholder used in the function. These are two different variables.
4) It does return something, but it doesn't output anything. You'd have to echo the contents out to the page in some form to see the results. The easiest way is just adding echo
before the function call so that the returned value will be sent to the echo command like this:
<?php
function sum_two_numbers($one,$two) {
$sum = $one + $two;
return $sum;
}
//added echo here
echo sum_two_numbers(1,5);
// Lastly, this one returns nothing. I assume that is just because no "echo" is called,
// but would there also be something to the line that just has "sum_two_numbers(1,5);"?
// Or am I just making it out to be more than it really is? :P
?>
I hope that clears some things up for you!
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsThanks! :)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou are very welcome, William. If you have any more questions, I'll do my best to answer them. :)
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsSo, just out of curiosity, would you know why this code:
outputs "01"?
Would that be because it takes in "Cake Batter" as array-index "0" and "Cookie Dough" as array-index "1" and outputs them together?
Thanks!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThat is pretty much exactly right. Just to clarify, though, it outputs them together because there aren't spacing, line breaks, or elements that break apart the two indices.
Just for giggles here's two ways you can look at the foreach loop:
Number One (no association)
Number Two