Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial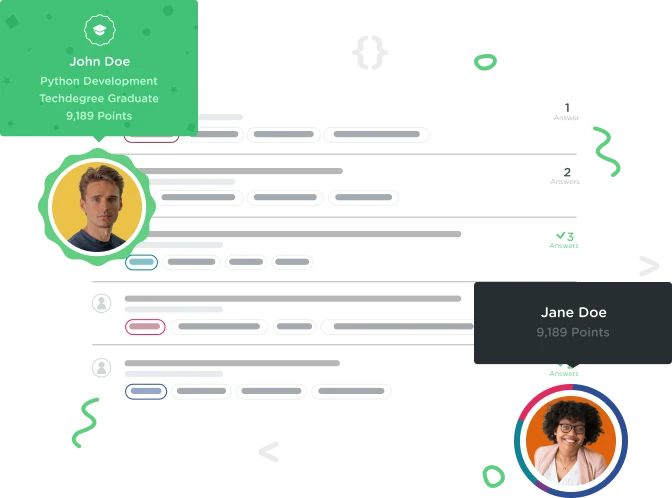

Jeffry Hidayat
8,844 PointsUnderstanding the importance of next() or next(err).
So in your route.js line 92-107 that specifies --->. router.post("/:id/answers/:aid/vote-:dir", (req, res, next) => {....
I was playing around with it a bit to understand deeper what is the role of next().
// Below code will work just fine, but I am trying to understand what will return next(err) does since "(req, res) => {" does not specify next in it.... router.post("/:id/answers/:aid/vote-:dir", (req, res, next) => { console.log(req.url); if (req.params.dir.search(/^(up|down)$/) === -1) { console.log("not found"); var err = new Error("Not Found"); err.status = 303; next(err); } else { console.log("test 1"); req.vote = req.params.dir; console.log("vote is " + req.vote); next(); }}, (req, res) => { console.log("test 2"); req.answer.vote(req.vote, function(err, question){ if (err) { console.log("Found error!"); console.log("Found an error: " + err.message); return next(err); } res.json(question); }); } );
// So I modified the function a bit to be as below... router.post("/:id/answers/:aid/vote-:dir", (req, res, next) => { console.log(req.url); if (req.params.dir.search(/^(up|down)$/) === -1) { console.log("not found"); var err = new Error("Not Found"); err.status = 303; next(err); } else { console.log("test 1"); req.vote = req.params.dir; console.log("vote is " + req.vote); next(); }}, (req, res, next) => { console.log("test 2"); req.answer.vote(req.vote, function(err, question){ if (err) { console.log("Found error!"); console.log("Found an error: " + err.message); return next(err); } res.json(question); next(); }); }, (req, res, next) => { console.log("Hey I am next"); var err = new Error("Testing Not Found"); err.status = 505; next(err); } );
From the modified code snippet above, I notice everytime I do post 'vote-up', I am getting below in the console log:
Hey I am next POST /questions/5ac5120f4ac14dc70685bd41/answers/5ac5122b4ac14dc70685bd42/vote-up 200 3.720 ms - 264 status is 505. ----> This is printed out by the exception hander in app.js Error [ERR_HTTP_HEADERS_SENT]: Cannot set headers after they are sent to the client at validateHeader (_http_outgoing.js:503:11) at ServerResponse.setHeader (_http_outgoing.js:510:3) at ServerResponse.header (/Users/administrator/express_api/node_modules/express/lib/response.js:767:10) at ServerResponse.send (/Users/administrator/express_api/node_modules/express/lib/response.js:170:12) at ServerResponse.json (/Users/administrator/express_api/node_modules/express/lib/response.js:267:15) at app.use (/Users/administrator/express_api/app.js:56:8) at Layer.handle_error (/Users/administrator/express_api/node_modules/express/lib/router/layer.js:71:5) at trim_prefix (/Users/administrator/express_api/node_modules/express/lib/router/index.js:315:13) at /Users/administrator/express_api/node_modules/express/lib/router/index.js:284:7 at Function.process_params (/Users/administrator/express_api/node_modules/express/lib/router/index.js:335:12) at next (/Users/administrator/express_api/node_modules/express/lib/router/index.js:275:10) at Layer.handle_error (/Users/administrator/express_api/node_modules/express/lib/router/layer.js:67:12) at trim_prefix (/Users/administrator/express_api/node_modules/express/lib/router/index.js:315:13) at /Users/administrator/express_api/node_modules/express/lib/router/index.js:284:7 at Function.process_params (/Users/administrator/express_api/node_modules/express/lib/router/index.js:335:12) at Immediate.next (/Users/administrator/express_api/node_modules/express/lib/router/index.js:275:10)
AND... if I don't pass in next in the last call back parameter, I am getting below output.
POST /questions/5ac5120f4ac14dc70685bd41/answers/5ac5122b4ac14dc70685bd42/vote-up 200 40.144 ms - 264 status is 500 Error [ERR_HTTP_HEADERS_SENT]: Cannot set headers after they are sent to the client at validateHeader (_http_outgoing.js:503:11) at ServerResponse.setHeader (_http_outgoing.js:510:3) at ServerResponse.header (/Users/administrator/express_api/node_modules/express/lib/response.js:767:10) at ServerResponse.send (/Users/administrator/express_api/node_modules/express/lib/response.js:170:12) at ServerResponse.json (/Users/administrator/express_api/node_modules/express/lib/response.js:267:15) at app.use (/Users/administrator/express_api/app.js:56:8) at Layer.handle_error (/Users/administrator/express_api/node_modules/express/lib/router/layer.js:71:5) at trim_prefix (/Users/administrator/express_api/node_modules/express/lib/router/index.js:315:13) at /Users/administrator/express_api/node_modules/express/lib/router/index.js:284:7 at Function.process_params (/Users/administrator/express_api/node_modules/express/lib/router/index.js:335:12) at next (/Users/administrator/express_api/node_modules/express/lib/router/index.js:275:10) at Layer.handle_error (/Users/administrator/express_api/node_modules/express/lib/router/layer.js:67:12) at trim_prefix (/Users/administrator/express_api/node_modules/express/lib/router/index.js:315:13) at /Users/administrator/express_api/node_modules/express/lib/router/index.js:284:7 at Function.process_params (/Users/administrator/express_api/node_modules/express/lib/router/index.js:335:12) at Immediate.next (/Users/administrator/express_api/node_modules/express/lib/router/index.js:275:10)
-----> Notice the error code is 500 because in app.js exception handling, we are doing app.use((err, req, res, next) => { res.status(err.status || 500); .......
So could you please help me explain the role of next in below points:
What if we call next() in the callback function definition, but we don't specify it in the call back function parameter?
Why am I getting ERR_HTTP_HEADERS_SENT when calling next in the last callback function?
How does it know whether it calls the next call back in line or exception handling function when we call next() vs next(err)?
Appreciate your help in advanced.
2 Answers
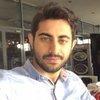
HIDAYATULLAH ARGHANDABI
21,058 PointsFor errors returned from asynchronous functions invoked by route handlers and middleware, you must pass them to the next() function, where Express will catch and process them. -next (); will pass to the next middleware. and next(err) will pass to the next middle ware will also return an error

Zack Jackson
30,220 Pointscalling next tells express to move on to the next piece of middleware. When you pass in err, it goes to the next piece of middleware but the error is caught and handled. Without the next call, it would seem like your app froze essentially.