Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial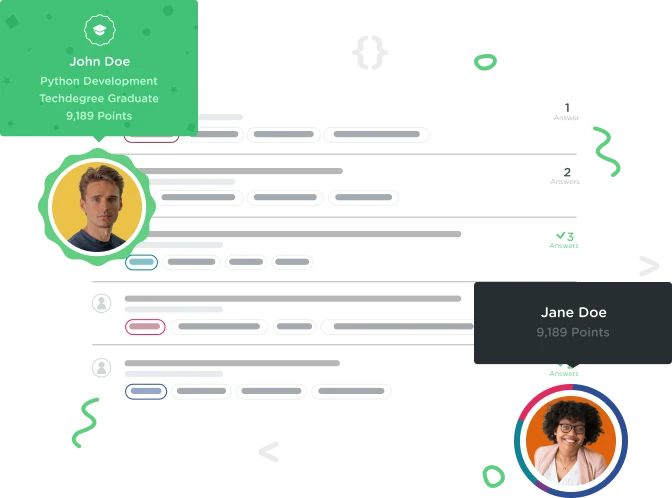

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsUnderstanding the Refactor functions
I really want to understand how these functions work to the point where I could explain and teach them to someone else. I have even resorted to handwriting all of this code to help internalize and understand what is going on. However, I need your help fully understanding.
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
This function allows me to turn this code:
const label = document.createElement('label');
label.textContent = 'Confirmed';
...into this:
const label = createElement('label', 'textContent', 'Confirmed')
However, this surely very simple part confounds me. How does the function know to use the const variable label
as the element
in element[property]
when label
was passed into function only as the parameter of elementName
?
I type const label = createElement(x, y, x)
but label
is outside the function except as elementName
Does anyone understand what I am getting at? I feel as though I am overthinking this but when I go to explain it out loud, I am confused.
But then this leads me to the following question:
if the function appendToLI
allows us to take this code:
const editButton = createElement('button', 'textContent', 'edit');
li.appendChild(editButton);
and turn it into
appendToLI('button', 'textContent', 'edit');
then what happened to const editButton
and appendChild(editButton)
? Because as far as I understand we just removed the variable or element of editButton
completely.
I've watched this video a few times and I think I mostly understand but these things still remain unclear to me. I will continue to watch over again.
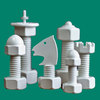
Steven Parker
231,269 PointsYou should start a fresh, new question and be sure to show your code or provide a link to a workspace snapshot.
2 Answers
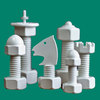
Steven Parker
231,269 PointsThe "label" outside the function is set to the same thing as "element" (not "elementName") is inside the function. And the parameters only stand for the actual values that are supplied when the function is called.
And you're right that the other code removed the variable "editButton" completely. What it used to do is now done by the variable "element" inside the function, but it is not returned by the function. This works because it is not needed by the rest of the program once it has been appended to the list item.

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsI guess what has not clicked for me yet is I could write const label = createElement(x, y, z);
but how does "label" here which is just the variable that createElement is assigned to make its way inside to be equal to "element"?
I do not mean to be dense here. Thank you for your patience.

Zimri Leijen
11,835 Pointsconst label and const element are just names.
You can name them apple and orange and it would still work.

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsZimri Leijen , I thought this too but if I go into my code and change "const label" to "const xyz" I get errors in the console and the website no longer works.
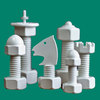
Steven Parker
231,269 PointsYou would need to go through the rest of the code and change other references to "label" into "xyz" to make it work. The actual name is not important, but it must be used consistently throughout the program.

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsYeah, that makes sense. As for the rest of it, I still do not understand, but with time and practice perhaps things will become more clear.

Zimri Leijen
11,835 PointsI'll just focus on the first part of the question.
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
const label = createElement('label', 'textContent', 'Confirmed')
What this effectively does is, it will call the createElement with the arguments 'label', 'textContent' and 'Confirmed'
So effectively, it says Hey, function createElement, please do your job, and interpret elementName as being 'label', property as being 'textContent' and value as being 'Confirmed'
So createElement goes to work, does his job, so
const element = document.createElement('label') // remember we swap elementName for 'label', because those were the arguments provided)
element['textContent'] = 'Confirmed'// again, remember to fill in the arguments. btw this is equivalent to element.textContent = 'Confirmed'. They're just using brackets, because dot notation doesn't support dynamic values, while brackets do.
return element.
hope that helps.

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsThank you. I do understand that part but the variable "element" is where I get confused.
Jelena Feliciano
Full Stack JavaScript Techdegree Student 12,729 PointsJelena Feliciano
Full Stack JavaScript Techdegree Student 12,729 PointsI'm having similar issues. looks like my edit and remove buttons turned in to text