Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial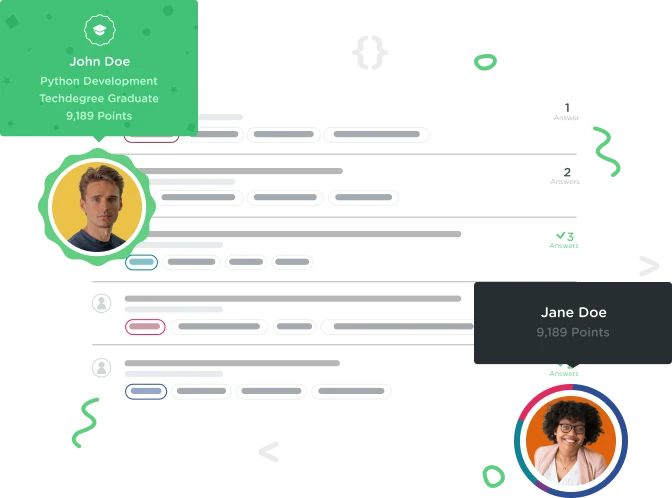

Steven Griffiths
3,165 PointsUnderstanding this if else code block + isset
php
if (isset($_GET["id"])){
$product_id = $_GET["id"];
if (isset($products[$product_id])) {
$product = $products[$product_id];
echo "we have a valid shirt ID";
}
else {
echo "we have an invalid shirt ID";
}
} else {
echo "We don't have a shirt ID";
}
My god this is confusing. Been watching the video over and over and have looked at the other comments on the forum.
I 'm pretty sure this wrapping of if and else statements, and using isset inside them is where I gave up in my previous attempts at PHP, as it just makes me feel as though my brain is malfunctioning!
Can somebody try explaining this all over again? I can't get my head around this block of code at all.
Thanks in advance
2 Answers

Christian Andersson
8,712 PointsOkay, line-by-line:
if (isset($_GET["id"])) {
checks whether or not the GET-variableid
has a value, or in other words: is set. If it does, enter the if-clause below. If the GET-variable doesn't have a variable, then print the messagewe don't have a shirt ID
.$product_id = $_GET["id"];
give the value of the GET-variable to a local variable calledproduct_id
. This is not a necessary step, but I guess a good practise.if (isset($products[$product_id])) {
checks whether or not the arrayproducts
on rowproduct_id
has a value. The variableproduct_id
is a number so it checks if the array has a value on theproduct_id
-th row. E.g if a said product has an id of 5 then this code checks if the arrayproducts
has a value on the 5th row. If not - it doesn't exist and print the messagewe have an invalid shirt ID
.$product = $products[$product_id];
just like in line 2, this isn't necessary. But it makes it easier to manage data if it's put on a shorter variable.
Hope this helps :)

Joseph Perez
25,122 PointsSo the isset function checks wether the variable is set or null. If the variable is set to something other then null or false then it will return true. Basically it will return true if the variable exists.
In the first part of the block of code it's getting the id. It then checks to see if it's set. If it returns true and it is indeed set then it assigns the id to the $product_id variable. After it has done that it continues down the script to the next if statement. Then it checks to see if the products id of the products array is set. If that is set and returns true just like in the previous if statement, it will assign the product id of the products array to a variable called $product. After that it will then echo out a message saying that it's a valid shirt id.
The else statements would be triggered if the if isset function returned false rather then true. So in the first if statement it would check to see if there was even an id to begin with. If it returns false it then moves to the else block of the first if statement and then echos out the message that there is no shirt id. Same thing applies to the second if statement. If it returns false then it will move down to it's else block and execute the code inside it, which is just echoing out the message that it's an invalid shirt id.
Hopefully that helped you a little bit. I'm new to answering on the forums so sorry that I didn't include some pictures as reference. I'm still getting the hang of it. Stick with it though! It might not make sense now but you will have that moment where it all starts to come together. :)

Steven Griffiths
3,165 PointsThanks for the help Joseph.
The way you put it about the isset function made me realise how simple that is. It's just when I'm confronted with a few different concepts in one go I can't see the wood for the trees.
Coding does not come natural to me. For each new concept I learn with code I have to run over it again and again, and play around with it and test it until it really sinks in.
I am very much looking forward to that moment you mention when it all comes together.
Will keep you posted! :-)
Steven Griffiths
3,165 PointsSteven Griffiths
3,165 PointsThanks for taking the time to run through that. It's mostly clear. I understand isset 100% now. Just a few other things I would like to clarify though.
$product_id = $_GET["id"];
You say this is not necessary, so in theory could I use instead?
$_GET["id"]
And what about this?
$product = $products[$product_id];
OK, can you explain what the alternative is here. You say it's good practice so I won't use the alternative, but knowing may help me understand what's going on fully.
Thanks again for the help. You've explained it very well.
Christian Andersson
8,712 PointsChristian Andersson
8,712 Pointsyes you can use
$_GET["id"]
if you'd like - there is nothing wrong about that.But I'd say it's a good practise not to use it though because... well it's just "cleaner" for you as a programmer to just use a local variable.
I think of it as the same reason you would want to first copy a math problem onto your sheet in a test before solving it. It makes it easier to read and easier to see the known's and unknown's of the problem and thus easier to solve it if everything is written down in one place rather than having the mess of papers laying everywhere.
If you don't feel comfortable doing it this way, or if you feel it's redundant, then that's perfectly fine and acceptable too. But I think that increases the chances of error.
Same thing goes for
$product = $products[$product_id];
. There is absolutely nothing wrong with using$products[$product_id];
if you'd prefer, but it's both easier to type and remember and less likely to miss-type if you use a shorter variable of your choice.This practise is almost always just to make your life easier, and doesn't have an impact on the actual program. Use it where you feel it's necessary to use a local variable. Basically where you think that you have a variable that is (1) hard to remember, (2) long to type out, or (3) will be frequently used.