Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial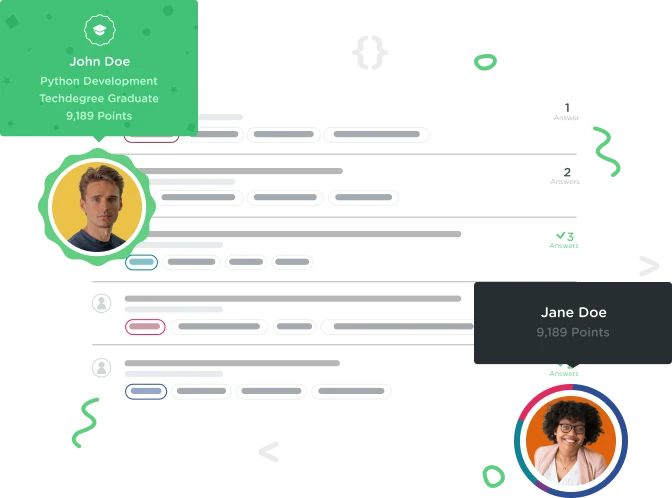

Jodie Wong
3,522 PointsUnderstanding what this code means exactly
I just wanted to clear up my understanding of the following code:
View.OnClickListener Listener = new View.OnClickListener() {
@Override
public void onClick(View view) { // Detects when button is clicked
// Then this code block is ran
// The button was clicked, updates fact label with new fact
String fact = "";
// Randomly select another fact
Random randomGenerator = new Random(); // Construct new random number generator
int randomNumber = randomGenerator.nextInt(3);
//fact = randomNumber + ""; // Converts the randomNumber to string
// Randomly update label with dynamic fact
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(Listener);
Ok so that 1st bit: View.OnClickListener Listener = new View.OnClickListener(). Creates a new onclicklistener called listener. But what is the "View.onClickListener" actually doing. It looks like View is a class and onClickListener is a method or variable or somehing.
2nd bit: There is a semicolon after the code block for the listener }; and then there's the "showFactButton.setOnClickListener(Listener);". My understanding is that "View.OnClickListener Listener = new View.OnClickListener()..." is like a function prototype where a function is being defined and declared. and the "showFactButton.setOnClickListener(Listener);" is calling that function where showFactButton is an object and setOnClickListener is the method/function we've just declared with the parameter "Listener".
Sorry if I'm sounding very jumbled or redundant. Most of this looks like C++ to me and I just don't want to get things from the 2 mixed up
1 Answer

Wesley Seago
10,424 Points"View.onClickListener listener = new onClickListener() {}"
In Java, the new keyword creates an object. In this statement, "View.onClickListener" defines the object type that is being declared.
"listener" is the variable name that you are assigning to the object that you are about to create. (this is case sensitive, and in your question you state Listener/listener. Be careful. Also, the variable name should be lowercase/camelCase, not Uppercase for a variable.)
"=" is the assignment operator. You have stated what type of object and what variable name. After the equals sign you will define the object.
"new" as stated above is a Java keyword for creating objects.
"onClickListener()" is actually a call to the constructor method.
In pseudo-speak you are saying "Create a new object of the type onClickListener, using the constructor method onClickListener(), and name it "listener".
The final portion, "showFactButton.setOnClickListener(listener)" is assigning the "onClickListener" with the variable name "listener" to the "showFactButton".
Pseudo-speak for this would be "Listen to the button named 'showFactButton', and if it is clicked, run the 'onClick' method that is associated with the 'listener' object.
I hope this helps to clear it up a little for you.

sahil shokeen
1,579 Pointsthanx a ton!!!
Murat Hasdemir
Front End Web Development Techdegree Graduate 20,968 PointsMurat Hasdemir
Front End Web Development Techdegree Graduate 20,968 Pointsfor first bit: View is a class and onClick is a method thats right in android we have some core classes which we can call and use their methods. You will see they are all common things that we uses in our apps. for second part : yes
for programming actually basics are same methodology is different so you can see so many similarities on the way.