Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial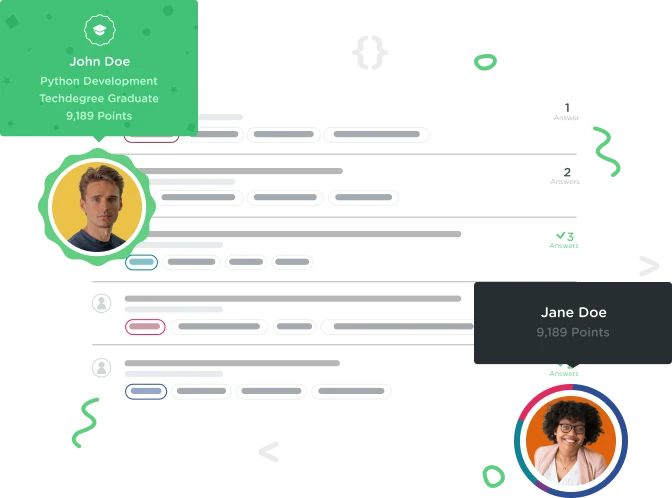
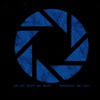
James N
17,864 Pointsunexpected EOF while parsing?
after typing that big, long, mess of code; i'm getting an error saying "unexpected eof while parsing" i'll probably find out what i did wrong in like, 2 seconds (lol) but, in case i don't, i have this! thanks for the help!
from copy import copy
import json
from operator import attrgetter, itemgetter
from functools import reduce
class Book:
def __init__(self, **kwargs):
for k, v in kwargs.items():
setattr(self, k, v)
def __str__(self):
return self.title
def __repr__(self):
return str(self)
def get_books(filename, raw=False):
try:
data = json.load(open(filename))
except FileNotFoundError:
return []
else:
if raw:
return data['books']
return [Book(**book) for book in data['books']]
BOOKS = get_books('books.json')
RAW_BOOKS = get_books('books.json',raw=True)
#pub_sort = sorted(RAW_BOOKS, key=itemgetter("publish_date"))
#pages_sort = sorted(BOOKS, key=attrgetter('number_of_pages'), reverse = True)
#print(pages_sort[0].number_of_pages, pages_sort[-1].number_of_pages)
#important_list = [1, 9, 5]
#important_list.sort() #Bad idea! perm. sorting!
# sorted(important_list) #Sorts a copy. not as bad.
#print(sorted(important_list))
#print(important_list)
def sales_price(book):
"""Apply a 20% discount to the book's price"""
book = copy(book)
book.price = round(book.price-book.price*.2, 2)
return book
#sales_books = list(map(sales_price, BOOKS))
#sales_books2 = [sales_price(book) for book in BOOKS]
#print(BOOKS[0].price)
#print(sales_books2[0].price)
def has_roland(book):
return any(["Roland" in subject for subject in book.subjects])
def titlecase(book):
book = copy(book)
book.title = book.title.title()
return book
#print(list(map(titlecase, filter(has_roland, BOOKS))))
def is_good_deal(book):
return book.price <= 5
#cheap_books = sorted(
# filter(is_good_deal, map(sales_price, BOOKS)),
# key=attrgetter("price")
#)
#print(cheap_books[0])
#print(cheap_books[0].price)
### REDUCE ###
def product(x, y):
return x * y
#print(reduce(product, [1,2,3,4,5]))
def add_book_prices(book1, book2):
return book1 + book2
#total = reduce(add_book_prices, [b.price for b in BOOKS])
#print(total)
def long_total(a = None, b = None, books = None):
if a is None and b is None and books is none:
return None
if a is None and b is None and books is not None:
a = books.pop(0)
b = books.pop(0)
return long_total(a, b, books)
if a is not None and books and books is not None and b is None:
b = books.pop(0)
return long_total(a, b, books)
if a is not None and b is not None and not books:
return long_total(a+b, None, books)
if a is not None and b is None and not books or books is None:
return a
print(long_total(None, None, [b.price for b in BOOKS])
phew! look at all that code!
1 Answer
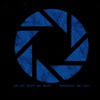
James N
17,864 PointsHah! told you so! found out what i did wrong!
(forgot to put bracket at end on line 101)
i also encountered a 2nd error, (forgetting to capitalize None on line 87)
but now the file returns None!
from copy import copy
import json
from operator import attrgetter, itemgetter
from functools import reduce
class Book:
def __init__(self, **kwargs):
for k, v in kwargs.items():
setattr(self, k, v)
def __str__(self):
return self.title
def __repr__(self):
return str(self)
def get_books(filename, raw=False):
try:
data = json.load(open(filename))
except FileNotFoundError:
return []
else:
if raw:
return data['books']
return [Book(**book) for book in data['books']]
BOOKS = get_books('books.json')
RAW_BOOKS = get_books('books.json',raw=True)
#pub_sort = sorted(RAW_BOOKS, key=itemgetter("publish_date"))
#pages_sort = sorted(BOOKS, key=attrgetter('number_of_pages'), reverse = True)
#print(pages_sort[0].number_of_pages, pages_sort[-1].number_of_pages)
#important_list = [1, 9, 5]
#important_list.sort() #Bad idea! perm. sorting!
# sorted(important_list) #Sorts a copy. not as bad.
#print(sorted(important_list))
#print(important_list)
def sales_price(book):
"""Apply a 20% discount to the book's price"""
book = copy(book)
book.price = round(book.price-book.price*.2, 2)
return book
#sales_books = list(map(sales_price, BOOKS))
#sales_books2 = [sales_price(book) for book in BOOKS]
#print(BOOKS[0].price)
#print(sales_books2[0].price)
def has_roland(book):
return any(["Roland" in subject for subject in book.subjects])
def titlecase(book):
book = copy(book)
book.title = book.title.title()
return book
#print(list(map(titlecase, filter(has_roland, BOOKS))))
def is_good_deal(book):
return book.price <= 5
#cheap_books = sorted(
# filter(is_good_deal, map(sales_price, BOOKS)),
# key=attrgetter("price")
#)
#print(cheap_books[0])
#print(cheap_books[0].price)
### REDUCE ###
def product(x, y):
return x * y
#print(reduce(product, [1,2,3,4,5]))
def add_book_prices(book1, book2):
return book1 + book2
#total = reduce(add_book_prices, [b.price for b in BOOKS])
#print(total)
def long_total(a = None, b = None, books = None):
if a is None and b is None and books is None:
return None
if a is None and b is None and books is not None:
a = books.pop(0)
b = books.pop(0)
return long_total(a, b, books)
if a is not None and books and books is not None and b is None:
b = books.pop(0)
return long_total(a, b, books)
if a is not None and b is not None and not books:
return long_total(a+b, None, books)
if a is not None and b is None and not books or books is None:
return a
print(long_total(None, None, [b.price for b in BOOKS]))
nakalkucing
12,964 Pointsnakalkucing
12,964 PointsThanks! I had the same problem with my code. :D