Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial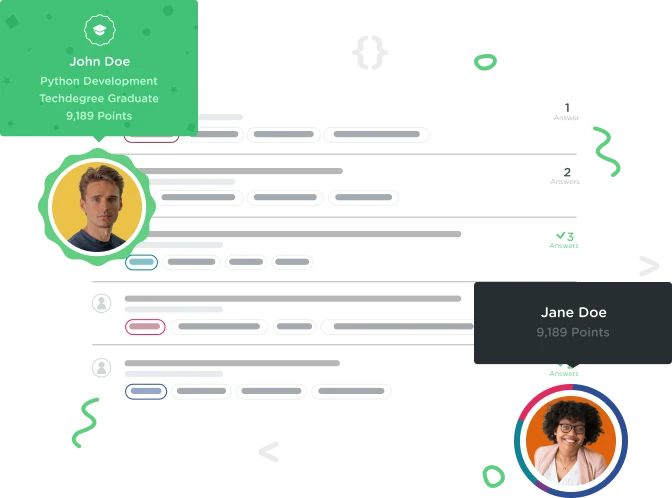

wc93
25,725 PointsUnexpected result when looping through mutable object such as lists?
Just wondering what exactly is going on? Does the for in loop get the index length once and then refer back to the list after the remove method has removed an item so the second iteration through the list it removes the third item?
I wrote a little python to try and figure out what was going on...
####### Unexpected results when looping through mutable list
nums = [1, 2, 3, 4, 5, 6]
nums2 = nums.copy()
# Unexpected result when looping through list and removing items
print("\nUnexpected looping results on mutable object\n")
for item in nums:
print(f"Item to be Removed: {item}")
print(f"List Before Remove: {nums}")
nums.remove(item)
print(f"List After Remove: {nums}")
# Using copy to remove unexpected results as each time through the list it is making a new copy of list
print(f"\n\nUsing copy to mitigate unexpected results while looping through mutable object\n")
for item in nums2.copy():
print(f"Item to be Removed: {item}")
print(f"List Before Remove: {nums2}")
nums2.remove(item)
print(f"List After Remove: {nums2}")
1 Answer
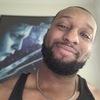
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi wc93,
I think maybe you're understanding what's going on, but I'm not super sure what you mean by "Does the for in loop get the index length once..."
The first iteration through the loop the item with an index of zero is removed. The second iteration through the loop the item with an index of one is removed. But the item with an index of one is no longer 2, because 1 has been removed. So now 2 is the first item in the list (or the item with the zero-ith index). So now the item with an index of one (or the item in the second position in the list) is 3. So 3 is removed. So now the list is [2, 4, 5, 6], now the item in the third position (or the item with an index of 2) is 5. So 5 will be removed.
wc93
25,725 Pointswc93
25,725 PointsThanks Brandon. That was how I was understanding it but not expressing properly. That is why I wrote the script to give me a better look at what was going on where.