Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial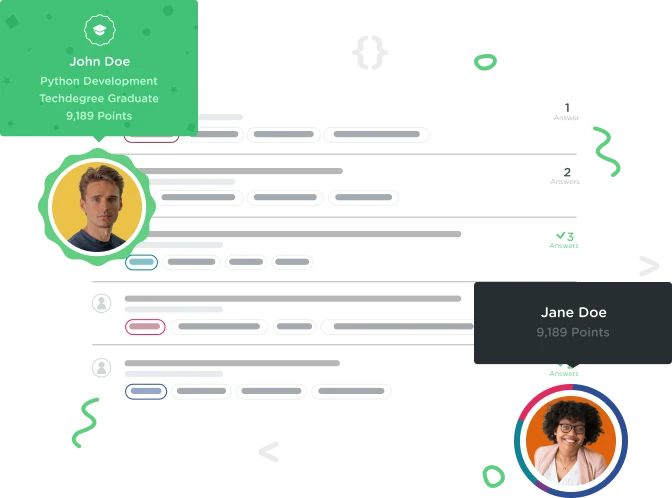
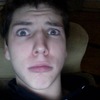
Charlie Person
8,692 PointsUnexpected results in the console.
I'm unclear on what is happening here. Jim said that if you call callTwice with () after the parameter, myFunction, then it would be undefined. So I just wanted to check to see what would happen. I tried it and rather than just giving me an undefined error, the console actually console.logged 'Function was called' one time and then 'Uncaught TypeError: undefined is not a function'. I don't quite understand why the first targetFunction worked but not the second. I would really appreciate some help. I wasn't vary clear on this lesson and I feel like it's quite crucial. Thanks!
var myFunction = function() { console.log('Function was called'); };
var callTwice = function(targetFunction) { targetFunction(); targetFunction(); }
callTwice(myFunction());
2 Answers

mrben
8,068 PointsHello
The callTwice
function is expecting a callable function to be passed in.
When you call it like this though, i.e. with the ()
...
callTwice(myFunction());
... you're not passing in the function but the result of running the function, which is undefined since myFunction
doesn't return anything. The ()
triggers the function and it's running there and then.
So myFunction
runs once on the line above, but then doesn't run again inside callTwice
because that function only has the return value (undefined) of the call to myFunction
.
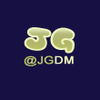
Jonathan Grieve
Treehouse Moderator 91,253 PointsI think (and I may be wrong because it's an anonymous function assigned to a variable) it's because you've add a semi colon after a function declaration. You don't need to add a semi colon after a function but you do after a function call.
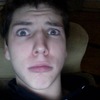
Charlie Person
8,692 PointsGood advice- but didn't quite answer my question. I just don't understand what is happening in the code. If you could break it down for me I would really appreciate it!
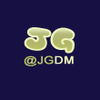
Jonathan Grieve
Treehouse Moderator 91,253 PointsI'll try. :D
First let me copy out your code into a Markdown format. Check the link below for how to use it by the way. :-)
var myFunction = function() { console.log('Function was called'); };
var callTwice = function(targetFunction) { targetFunction(); targetFunction(); }
callTwice(myFunction());
First there's an anonymous function. It turns out my advice to you was bad advice. You do use a semi colon to close an anonymous function because it is in itself a JavaScript statement.
It logs to the console, function was called. But at this stage, nothing had been called yet.
Next we have the function callTwice which takes a parameter target function. The parameter makes 2 function calls inside the named function. This time as you know, we don't use a semi colon.
Now this is where we start to do things a little differently. It looks like you're trying to call the anonymous function using the variable and and use that as the target function.
But you need to do a little more.
callTwice(function(){
console.log(myFunction);
});
Which should output your message "Function was called", twice in the console,
Full code
var myFunction = function() { console.log('Function was called'); };
var callTwice = function(targetFunction) { targetFunction(); targetFunction(); }
callTwice(function(){
console.log(myFunction);
});
Let me know if this work. :)
Charlie Person
8,692 PointsCharlie Person
8,692 PointsAhaaaa!!!! So you're saying that callTwice never runs right??????
mrben
8,068 Pointsmrben
8,068 PointscallTwice
runs but it tries to callundefined
and make it run ... which doesn't make any sense.This line:
callTwice(myFunction());
Is equivalent to:
callTwice(undefined);
What you really want, if you want to make it run twice, is to run this:
callTwice(myFunction);
Charlie Person
8,692 PointsCharlie Person
8,692 PointsYou're the man mr. Ben. The freaking MAN! Thank you so much! Really appreciate it. We'll meet one day. And when that day comes, I'll buy you a sandwich. You're the kind of dude that makes treehouse what it is.
mrben
8,068 Pointsmrben
8,068 PointsAww thanks, Charlie! No problem. :-)