Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial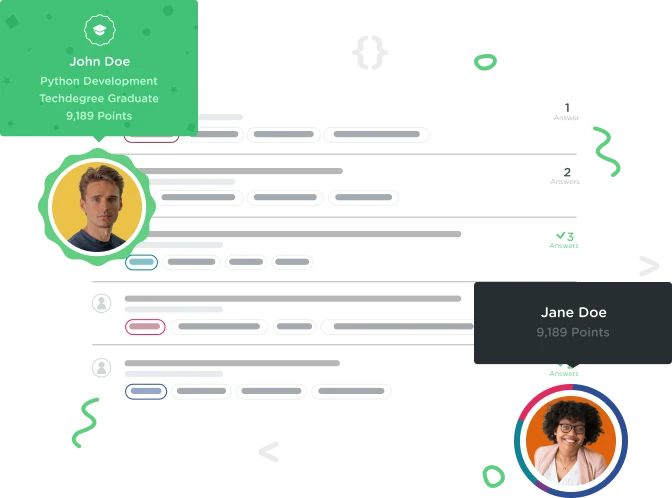

benjaminmosery
6,346 PointsUnexpected Token )
I have the following code, stated below, that, when runs, gives me the message "Unexpected Token )". Apparently I have an extra parenthesis but nothing seems out of place. A little guidance would be appreciated.
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
//Require https module
const https = require(`https`);
// function to print message to console
function printMessage(username,badgeCount,points){
const message = `${username} has ${badgeCount} total badge(s) and ${points} Total points.` ;
console.log(message);
}
//Connect to the API URL (https://teamtreehouse.com/username.json)
function GetProfile (username){
try {
//GET USERNAME PROFILE FROM TREEHOUSE USING FUNCTION
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
//REQUEST TREEHOUSE WEBSITE
//CALL RESPONSE IN DIRECTORY USING.DIR
//Read the Data in
let body = ``;
response.on('data', data => {
//GETTING THE BODY BY WRITING IN THE WEBSITE RESPONSE
body += data.toString(); //HAVING THE DATA ADDED TO THE BODY
}); //TURNED INTO A STRING
response.on(`end`, () => {
const profile = JSON.parse(body);
//PARSING JSON THE DATA IN THE BODY, STORING THE PARSED DATA IN A VARIABLE
//Print the Data
printMessage(username,profile.badges.length,profile.points.total);
//PRINT THE DATA USING PRINTMESSAGE, INCLUDE THE USERNAME VARIABLE, BADGE LENGTH FROM PROFILE DATA, AND NUMBER OF POINTS IN JAVASCRIPT FROM PROFILE DATA.
}); //END THE DATA RESPONSE
}); //END THE REQUEST
request.on('error', error => console.error('Problem with request: ${error.message}'));
} catch (errors) {console.error(error.message);} //GETPROFILE FUNCTION END
const users = process.argv.slice(2); //USER ARRAY, USES THE .argv ARRAY, CONTAINED IN THE process ARRAY TO PRESENT USER NAMES, STARTING AT THE SECOND VARIABLE IN THE argv array.
users.forEach(GetProfile); //USE THE .forEACH method TO CYCLE THROUGH EACH MEMBER OF THE USERS ARRAY AND GET THEIR PROFILE INFORMATION USING GetProfile
3 Answers
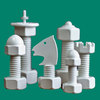
Steven Parker
229,744 PointsI don't know about the parenthesis, but there seems to be a closing brace missing.
The open brace that starts the body of the "GetProfile" method doesn't seem to have a counterpart.

benjaminmosery
6,346 PointsWhen I run this code, I still get "Unexpected Token )":
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
//Require https module
const https = require(`https`);
// function to print message to console
function printMessage(username,badgeCount,points){
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in Javascript.` ;
console.log(message);
}
//Connect to the API URL (https://teamtreehouse.com/username.json)
function GetProfile (username){
//GET USERNAME PROFILE FROM TREEHOUSE USING FUNCTION
try { //TRY FUNCTION TO CATCH ERRORS
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
//REQUEST TREEHOUSE WEBSITE (NO HTTPS:// TO LEARN ABOUT DEVELOPER-SIDE ERROR)
//CALL RESPONSE IN DIRECTORY USING.DIR
//Read the Data in
let body = ``;
response.on('data', data => {
//GETTING THE BODY BY WRITING IN THE WEBSITE RESPONSE
body += data.toString(); //HAVING THE DATA ADDED TO THE BODY
}); //TURNED INTO A STRING
response.on(`end`, () => {
//Parse the Json Data (as its in string format)
try { //TRY TO CATCH INVAID USERNAME ERRORS
const profile = JSON.parse(body);
//PARSING JSON THE DATA IN THE BODY, STORING THE PARSED DATA IN A VARIABLE
//Print the Data
printMessage(username,profile.badges.length,profile.points.JavaScript);
//PRINT THE DATA USING PRINTMESSAGE, INCLUDE THE USERNAME VARIABLE, BADGE LENGTH FROM PROFILE DATA, AND NUMBER OF POINTS IN JAVASCRIPT FROM PROFILE DATA.
} catch (error) {console.error(error.message); }
//TRY AND CATCH INVALID USERNAME ERRORS, PRINT OUT ERROR MESSAGE
}); //END THE DATA RESPONSE
}); //END THE REQUEST
request.on('error', error => console.error(`Problem with request: ${error.message}`))
//Error Handler (as request) to deal with possible Error Events in the Program
} catch(error) { console.error(error.message); }
//TRY AND CATCH ERROR, PRINT ERROR MESSAGE TO CONSOLE
const users = process.argv.slice(2); //USER ARRAY, USES THE .argv ARRAY, CONTAINED IN THE process ARRAY TO PRESENT USER NAMES, STARTING AT THE SECOND VARIABLE IN THE argv array.
users.forEach(GetProfile); //USE THE .forEACH method TO CYCLE THROUGH EACH MEMBER OF THE USERS ARRAY AND GET THEIR PROFILE INFORMATION USING GetProfile
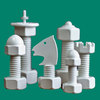
Steven Parker
229,744 PointsThere still doesn't seem to be any matching closing brace to go with the one that starts the "GetProfile" function. Try fixing that first.
If you still get an error after that, does it indicate which line it is on?

benjaminmosery
6,346 PointsSteven Parker, your suggestion did it. Apparently I missed a closing bracket that wouldn't let the Profile function run. Thank you!