Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial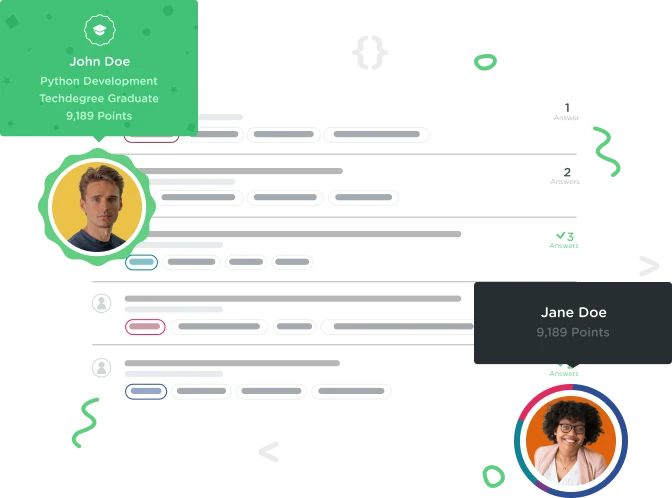

orange sky
Front End Web Development Techdegree Student 4,945 Pointsunexpected 'undefined' result
Hello!
1) when I run the code below, I get into the web console a result that I am expecting:
My expected result:
"greetingworldJames" test.html:18 5
2) But when, clear the console (Firefox web console), and I type: sayHello(); I get a different result. Why is that? *** if you see the code below name is defined to 'james', hence I got the result above.
My unexpected result:
sayHello(); TypeError: name is undefined "Helloworldundefined"
<!DOCTYPE html>
<html lang="en">
<head>
</head>
<body>
<script>
function sayHello (name, greeting){
if(typeof greeting === 'undefined')
{
greeting = 'Hello';
}
console.log (greeting + 'world' + name);
return name.length;
}
console.log (sayHello ("James", "greeting"));
</script>
</body>
</html>
4 Answers

Marcus Vieira
7,877 PointsHi!
Calling sayHello
without any parameters didn't work becaus the parameter name
was not being passed in as a parameter. Let's look at the stacktrace:
TypeError: name is undefined "Helloworldundefined"
So you can see that the first part of your code did work!
if (typeof greeting === 'undefined') {
greeting = 'Hello';
}
You didn't send a greeting
, so it used 'Hello': Helloworldundefined
But what's that undefined
doing there ?
it's the name
that you didn't pass as a parameter, so name = undefined
Therefore:
TypeError: name is undefined "Helloworldundefined"
To fix this, you could do:
function sayHello(name, greeting) {
if (typeof greeting === 'undefined') {
greeting = 'Hello';
}
if (typeof name === 'undefined') {
name = 'Default name'
}
console.log(greeting + 'world' + name);
return name.length;
}
console.log(sayHello("James", "greeting"));
Or:
function sayHello(name, greeting) {
console.log( (greeting || 'Hello') + ' world ' + (name || 'Default Name'))
console.log( 'Name length: ' + name.length )
}
On the second solution (greeting || 'Hello')
will do basically the same thing as your if
statement: it'll check if the variable greeting
is populated, if it is, it will use it, if not it'll say 'Hello'
I hope this helps!
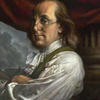
Jeff Busch
19,287 PointsHi orange,
You're not passing in any arguments to a function expecting arguments. The if statement assigns a value to greeting but name remains undefined. So the return statement is returning undefined which has no length.
Jeff
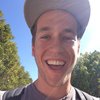
Chandler Tayek
5,513 PointsWhen you are in the console, you have to pass the function an argument for the name parameter like aka sayHello("James");. If you want the function to work without passing a name argument then you have to do a similar if statement to the one managing 'undefined' greeting parameters:
First fix(in console):
sayHello("James");
Second fix(in code):
<!DOCTYPE html>
<html lang="en">
<head>
</head>
<body>
<script>
function sayHello (name, greeting){
if(typeof name === 'undefined')
{
name = 'Mr. Noname';
}
if(typeof greeting === 'undefined')
{
greeting = 'Hello';
}
console.log (greeting + 'world' + name);
return name.length;
}
console.log (sayHello ("James", "greeting"));
</script>
</body>
</html>

orange sky
Front End Web Development Techdegree Student 4,945 PointsThank you all for your easy to understand explanations! I am really new to webconsole ...
Cheers!!!