Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial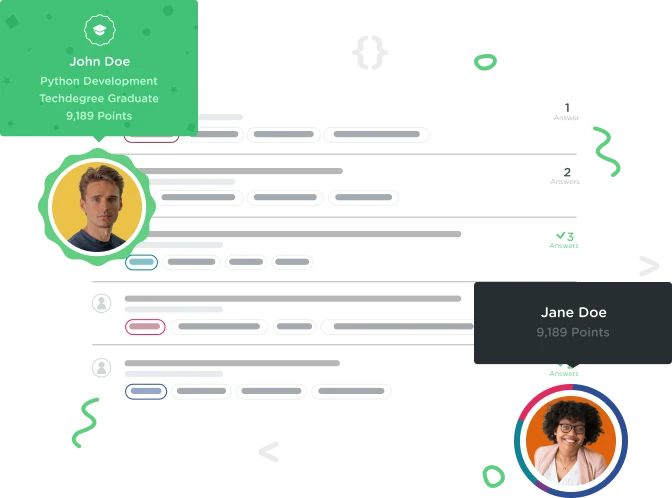
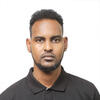
Abdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 PointsUnfortunately, the app has stopped working
as I am working on the project, my app stops if I add loadpage();
if I erase "loadpage();" everything works.
4 Answers
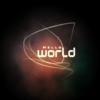
Kristian Terziev
28,449 PointsI notice you've used "Page" instead of "page" in quite a lot of places.
Ex:
(line 57 )String pageText = Page.getText();
(line 61) mChoice1.setText(Page.getChoice1().getText());
(line 62)mChoice2.setText(Page.getChoice2().getText());
Go throughout your code and review it. See if there are any more such errors. Remember that "Page" is a class (please write that with capital P in the page.java file). When you want to create an instance of that class you use it with a capital P, but the object itself is with lower case. In that case
Page page = new Page();
So fix those issues and see if there are still errors.
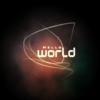
Kristian Terziev
28,449 PointsCould you include your code so we can take a look at it?
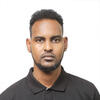
Abdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 Pointsyeah, sure, here its:
package shiidoow.interactivestory.ui;
import android.app.Activity;
import android.content.Intent;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import shiidoow.interactivestory.R;
import shiidoow.interactivestory.model.Story;
import shiidoow.interactivestory.model.page;
public class StoryActivity extends Activity {
public static final String TAG = StoryActivity.class.getSimpleName();
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private String mName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent = getIntent();
mName = intent.getStringExtra(getString(R.string.key_name));
if (mName == null)
mName = "Friend";
Log.d(TAG,mName );
mImageView = (ImageView) findViewById(R.id.StoryImageView);
mTextView = (TextView) findViewById(R.id.storyTextView);
mChoice1 = (Button) findViewById(R.id.choiceButton1);
mChoice2 = (Button) findViewById(R.id.choiceButton2);
loadpage();
}
private void loadpage (){
page Page = mStory.getpage(0);
Drawable drawable = getResources().getDrawable(Page.getmImageID());
mImageView.setImageDrawable(drawable);
String pageText = Page.getText();
pageText = String.format(pageText,mName);
mTextView.setText(pageText);
mChoice1.setText(Page.getChoice1().getText());
mChoice2.setText(Page.getChoice2().getText());
}
}
```java
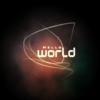
Kristian Terziev
28,449 PointsAlso please edit your coment and add "java" after the first three ` characters. As such
/ ```java
I would appreciate it if you include the error given in the log also. Thanks :)
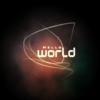
Kristian Terziev
28,449 Points page Page = mStory.getpage(0);
I believe this is your source of error. You should reverse the words. It's Page page because the first word defines the type of the second.
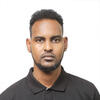
Abdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 Pointsow, I see. but isn't the first one referring to my java file? I have a file named "page.java" , I tried reversing words but I got many errors.
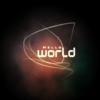
Kristian Terziev
28,449 PointsThe first word says that you are creating an instance of a class called Page (in this case). Please copy your errors and paste them here. If they are from a different file. Copy it also.
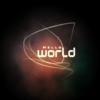
Kristian Terziev
28,449 PointsCare to include the StoryActivity class? If you can use some other website and just include all your files so we don't spam the comments. Provide a link for us after taht and we'll be able to review your code just fine.
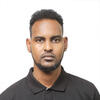
Abdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 PointsI do so, pasting all my files into another website and here is the link.
Abdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 PointsAbdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 PointsI wish things would be solved as I got confused which one is "Page or page" as I started changing for the rest of my code and the error still is there, but I'll go back to the Teachers Videos as I now know where my mistake was, Thank you very much Kristian Terziev :) for being helpful.....
Kristian Terziev
28,449 PointsKristian Terziev
28,449 PointsI'll try to explain classes to you in a simple matter so that you can tell the difference between "Page or page". Forget the apges for a moment. First a brief sentence as to what is a class - A class is a blue print that describes the behaviors/states that object of its type support. Now that may sound a little complex so let me give you an exmaple:
So what does the class I wrote do. By itself - nothing. It just says what an instance will be able to do. In that case what a parent will be able to do. Every parent can boss around (meaning tell you to do something), work, rest and tell you his name (or at least in my example). Therefore, every parent you "create" from now on, will be able to do those things.
Now, every class needs a constructor. It defines what is required to make an instance of the class. In this case, if you want to create a parent, he must have a name and age. So every time you create a parent you must pass "name" and "age" as parameters. As for the code inside the curly bracelets it assigns the name of the parent the one you gave and his age to the one you gave, also. The "this" stands to indicate that the variable is reffering to the one the parent has, not the one you passed. In this case - his name is equal to "nameOfParent" (the on you pass) and hsi age to "ageOfParent" (you pass that also) //NOTE: You may ahve more than one constructor. If your class was called Bucket (there are different kinds of bucktes) you may need different things for different buckets - material, color, size, shape, etc. You may also not need a thing and not write a constructor. A default one will be used.
So after all this talking, let's create a parent:
Parent mom = new Parent("Susan", 37);
This creates a parent called Susan at the age of 37. Her name is "mom". Now you can use "mom" to do the following things:
Therefore you can call those methods as such:
So now, when you need to get the parent's name (as you needed to get the page's text on line 57). What would you do? Will it be
Parent.getName();
OR
mom.getName();
Why? Let's view the first one I offered - You need a name, so you call a method called "getName". However, this method can be used only by instances of the class "Parent". You currently have only one - mom. Therefore, you must use her to get her name. You can't just ask Parent in general what is the name of a single instance. You must ask that instance itself. Meaning you should use the second example I gave
Now let's look at your Page/page problem. You have a class called Page. Remember that it is not a page itlsef - it just describes the states/behaviors that pages support. So when you create a new instance it looks like this:
Page firstPage = new Page(); // my page is called "firstPage" while yours was called just page
Now, just as the parent example, if you want to get the text of that page, what would you use:
firstPage.getText();
OR
Page.getText();
And that basically wraps it up. Try to really understand what goes on here. Forget the duplication of the word page. If you're more comfortable use different words so you can tell the difference between a class and its instance. Read what I wrote here, think about it and give me an example of class. I don't need any constructors or anything. Just a simple class with one or two function inside. Then create an instance of that class and do use one of it's methods. If anything is unclear, be sure to ask.
Abdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 PointsAbdiaziz Abdullahi Abdulle
Full Stack JavaScript Techdegree Student 7,458 PointsKristian Terziev, you're so helpful . I really appreciate that, and for this question you've asked:
"if you want to get the text of that page, what would you use:"
```java firstPage.getText(); OR
Page.getText(); ```java
things are clearer as a day now, it has to be
java firstPage.getText();
java if it's right, thanks in advance.Kristian Terziev
28,449 PointsKristian Terziev
28,449 PointsThat's correct :) Glad to know it cleared up.